30 Essential Annotations for Developing Spring Boot REST Web Services
- CODING Z2M
- Apr 19, 2023
- 7 min read
Updated: Jul 15, 2023
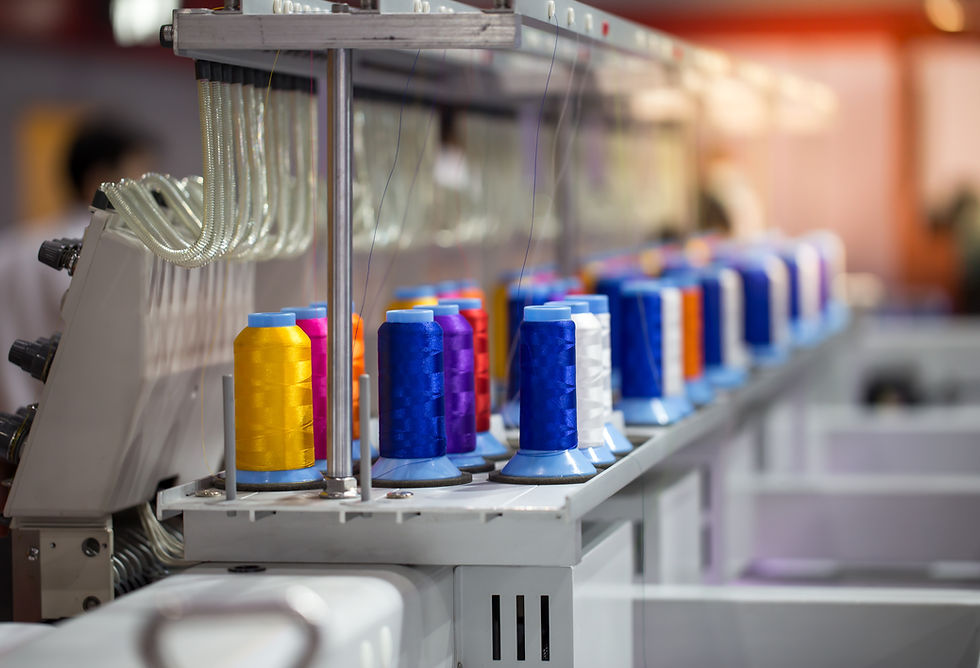
The Spring Framework provides a wide range of annotations that can be used to develop RESTful web services in a Spring Boot application. These annotations can be used to define endpoints, data models, and other aspects of the application. In this blog post, we will cover some of the most commonly used Spring Framework annotations in a Spring Boot REST API.
@RestController
The @RestController annotation is used to define a class as a RESTful web service controller. It combines the @Controller and @ResponseBody annotations, which means that it can be used to map HTTP requests to specific methods and return the response directly as JSON or XML data.
Here is an example of a RESTful web service controller that uses the @RestController annotation: @RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/users")
public List<User> getUsers() {
// retrieve list of users from database or other data source
return userList;
}
@PostMapping("/users")
public User createUser(@RequestBody User user) {
// save new user to database or other data source
return user;
}
// other methods for updating and deleting users
} @RequestMapping
The @RequestMapping annotation is used to map HTTP requests to specific methods in a RESTful web service controller. It can be used to define the URI path, HTTP method, and other request parameters.
Here is an example of using @RequestMapping to define a URI path for a RESTful web service endpoint: @RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/users")
public List<User> getUsers() {
// retrieve list of users from database or other data source
return userList;
}
// other methods for creating, updating, and deleting users
}
@PathVariable
The @PathVariable annotation is used to extract a variable from the URI path in a RESTful web service endpoint. It can be used to define dynamic parameters in the URI path, such as an ID for retrieving a specific resource.
Here is an example of using @PathVariable to retrieve a specific user by ID: @RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/users/{id}")
public User getUserById(@PathVariable Long id) {
// retrieve user with specified ID from database or other data source
return user;
}
// other methods for creating, updating, and deleting users
}
@RequestBody
The @RequestBody annotation is used to map the HTTP request body to a Java object in a RESTful web service endpoint. It can be used to accept JSON or XML data in the request body and map it to a Java object for processing.
Here is an example of using @RequestBody to create a new user: @RestController
@RequestMapping("/api")
public class UserController {
@PostMapping("/users")
public User createUser(@RequestBody User user) {
// save new user to database or other data source
return user;
}
// other methods for retrieving, updating, and deleting users
}
@ResponseStatus The @ResponseStatus annotation is used to define the HTTP response status code for a RESTful web service endpoint. It can be used to define a custom status code, such as 201 Created for a successful POST request.
Here is an example of using @ResponseStatus to define a custom status code for a successful POST request: @RestController
@RequestMapping("/api")
public class UserController {
@PostMapping("/users")
@ResponseStatus(HttpStatus.CREATED)
public User createUser(@RequestBody User user) {
// save new user to database or other data source
return user;
}
// other methods for retrieving, updating, and deleting users
}
@RequestParam This annotation is used to bind a request parameter to a method parameter in a Spring controller. For example: @GetMapping("/users")
@ResponseBody
public List<User> getUsersByRole(@RequestParam String role) {
// logic to retrieve users with specified role
return userList;
} @ExceptionHandler This annotation is used to specify a method to handle exceptions thrown by the controller. For example: @ExceptionHandler(UserNotFoundException.class)
@ResponseStatus(HttpStatus.NOT_FOUND)
public void handleUserNotFound() {
// logic to handle user not found exception
}
Aabout @SpringBootApplication @Configuration @ComponentScan @EnableAutoConfiguration
All four of these annotations are used in Spring Boot applications to simplify the configuration process and reduce the amount of boilerplate code required to set up a basic Spring application.
@SpringBootApplication This annotation is a combination of three other annotations: @Configuration, @EnableAutoConfiguration, and @ComponentScan. It is typically placed on the main application class and serves as a convenient way to enable all the features provided by those three annotations. It enables Spring Boot's autoconfiguration feature, which automatically configures certain aspects of the application based on the classpath and other settings.
@Configuration This annotation is used to indicate that a class is a Spring configuration class. Configuration classes provide bean definitions and other configuration information to the Spring context. They are typically used to define beans that need to be created by the Spring container, such as data source objects or web service clients.
@ComponentScan This annotation is used to specify the base package for component scanning. Component scanning is a process by which Spring looks for classes annotated with @Component, @Service, @Repository, and other similar annotations, and registers them as beans in the Spring context. By default, Spring Boot will scan for components in the package that contains the main application class.
@EnableAutoConfiguration This annotation is used to enable Spring Boot's autoconfiguration feature. Autoconfiguration uses classpath scanning and reflection to automatically configure certain aspects of the application based on the dependencies and other settings. For example, if the application includes the Spring Data JPA dependency, Spring Boot will automatically configure a data source and entity manager for use with JPA.
@Autowired
This annotation is used to automatically wire a bean dependency. When placed on a constructor, setter method, or field, Spring will attempt to find a matching bean in the application context and inject it. This eliminates the need for manual configuration of dependencies in XML or Java-based configuration.
@Qualifier
This annotation is used in conjunction with @Autowired to specify the exact bean to be injected. If there are multiple beans of the same type in the context, @Qualifier allows you to select the specific bean to be injected by name.
@Transactional
This annotation is used to mark a method, or an entire class, as transactional. When used on a method, Spring will automatically begin a transaction before the method is called, and commit or rollback the transaction when the method completes. Transactions ensure that all database operations are atomic and consistent.
@Async
This annotation is used to indicate that a method should be executed asynchronously. When placed on a method, Spring will automatically create a new thread to execute the method and return immediately, allowing the calling thread to continue processing.
@ControllerAdvice This annotation is used to define global exception handling for a Spring application. When an exception is thrown from a controller method, Spring will first look for an exception handler method in the same controller, and if one is not found, it will look in any classes annotated with @ControllerAdvice.
@RequiredArgsConstructor
RequiredArgsConstructor is a feature provided by the Lombok library which can be used in Spring Boot applications to automatically generate constructors for classes that have final fields. To use RequiredArgsConstructor in a Spring Boot application, you will need to add the Lombok dependency to your project's build configuration file (e.g. pom.xml for Maven or build.gradle for Gradle), and then annotate your class with @RequiredArgsConstructor.
@Data The @Data annotation generates getters and setters for all fields in a class, a toString() method, an equals() method, and a hashCode() method. This annotation is often used to create lightweight POJO (Plain Old Java Object) classes.
@NoArgsConstructor The @NoArgsConstructor annotation generates a no-argument constructor for a class. This is useful when you need to create instances of a class without passing any arguments to its constructor.
@AllArgsConstructor The @AllArgsConstructor annotation generates a constructor that takes arguments for all fields in a class. This is useful when you need to create instances of a class and set all of its fields in one go.
@Builder The @Builder annotation generates a builder pattern for a class. This pattern allows you to create instances of a class by chaining method calls together, which can make the code more readable and easier to maintain. The builder pattern is especially useful when you have a class with many optional fields.
Stereotype Annotations Stereotype annotations are a group of annotations in the Spring Framework that are used to provide metadata about the role or purpose of a particular class. These annotations are used to add clarity and simplify the configuration of Spring applications.
The four most commonly used stereotype annotations in Spring are:
@Component This annotation is a generic stereotype annotation for any Spring-managed component. It is used to mark a Java class as a Spring-managed bean and enables the class to be automatically detected and registered by Spring.
@Service This annotation is a specialization of the @Component annotation and is used to mark a class as a service component in the service layer of a Spring application. It is typically used to define business logic and other services.
@Repository This annotation is also a specialization of the @Component annotation and is used to mark a class as a repository component in the persistence layer of a Spring application. It is typically used to define DAO classes and database access logic.
@Controller This annotation is used to mark a class as a controller component in the web layer of a Spring application. It is typically used to define request handling methods for web requests.
By using these stereotype annotations, developers can easily group and manage related classes in a Spring application. Additionally, Spring provides many convenience features, such as automatic dependency injection and transaction management, that work seamlessly with these annotations.
It's worth noting that these stereotype annotations are all meta-annotations themselves
@Bean
This annotation is used to indicate that a method produces a bean to be managed by Spring. The method is responsible for creating and configuring the bean, which will be returned by Spring when requested.
@Profile
This annotation is used to specify which profile(s) a bean should be included in. Profiles are used to specify different configurations for different environments, such as development, testing, or production.
@PropertySource
This annotation is used to specify the location of a properties file that contains key-value pairs that can be injected into Spring-managed beans using the @Value annotation.
@Value
This annotation is used to inject a value from a properties file or a system environment variable into a Spring-managed bean. It can be used to inject simple values like strings or integers, or complex objects like lists or maps.
@ConfigurationProperties
This annotation is used to bind a configuration properties file to a Java class. It allows developers to specify default values for properties and to validate the configuration properties at runtime.
Conclusion
These are just a few examples of the many annotations available in the Spring Framework that can be used to develop RESTful web services in a Spring Boot application. By using these annotations, developers can quickly and easily define endpoints, data models, and other aspects of the application without having to write a lot of boilerplate code.
In addition to these annotations, there are many other features and tools available in the Spring Framework and Spring Boot that can be used to develop powerful and scalable RESTful web services. These include Spring Data, which provides a simple and consistent way to access data from a variety of sources, and Spring Security, which provides robust authentication and authorization features.
Overall, the Spring Framework and Spring Boot provide a powerful and flexible platform for developing RESTful web services. By using the right combination of annotations, features, and tools, developers can create high-quality, reliable, and scalable web services that meet the needs of their users and their business.
Comentarios