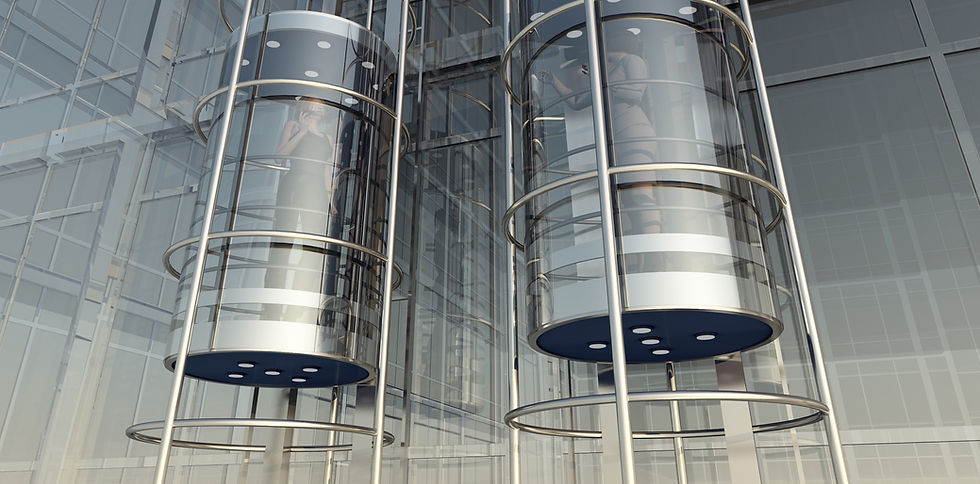
In Spring Boot, the Model-View-Controller (MVC) architectural pattern is commonly used to build RESTful web services. MVC separates the application logic into three interconnected components: the Model, the View, and the Controller. Here's how MVC is typically implemented in a Spring Boot REST web service, along with a real-world example:
"The Model represents the data and business logic, the View handles data serialization and transformation, and the Controller manages the flow of requests and responses."
Model: The Model represents the data and business logic of the application. It encapsulates the domain objects or entities that the application manipulates. In the case of a RESTful web service, the Model typically consists of Java classes that represent the data being exchanged between the client and the server. These classes may have annotations to define the data structure and relationships with other entities.
Example: Let's consider a blog application where users can create and view blog posts. The Model might include classes like Post and User, representing the blog post and user entities, respectively. These classes would contain attributes such as title, content, author, and timestamps.
View: In a RESTful web service, the View component is responsible for rendering the response data to the client. However, unlike traditional web applications that generate HTML views, RESTful services often return data in a structured format like JSON or XML. In this context, the View component is typically implemented using data serialization and transformation libraries.
Example: In our blog application, the View component might serialize the Post objects into JSON format to be returned as the response to the client. The serialized data would include the post's title, content, author, and other relevant details.
Controller: The Controller acts as an intermediary between the client and the Model and manages the incoming requests and outgoing responses. It receives requests from the client, invokes the appropriate business logic in the Model, and prepares the response by utilizing the View. In Spring Boot, the Controller is often implemented using annotations like @RestController and @RequestMapping.
Example: In our blog application, the Controller would define RESTful endpoints to handle operations such as creating a new post or retrieving a list of posts. For instance, a PostController class might have methods annotated with @PostMapping and @GetMapping to handle requests for creating a new post and retrieving posts, respectively. These methods would interact with the underlying Model to perform the necessary actions and use the View component to serialize the response data.
Overall, the MVC pattern in Spring Boot REST web services helps in separating concerns, improving maintainability, and promoting the re-usability of components.
Request Processing Flow: When a client sends a request to a Spring Boot REST web service, the request is first intercepted by the DispatcherServlet, which acts as the front controller. The DispatcherServlet routes the request to the appropriate Controller based on the URL mapping configured in the application.
Controller Actions: Inside the Controller, methods are defined to handle different types of requests and perform the necessary operations. These methods are commonly referred to as "Controller actions" or "Controller methods." They are typically annotated with specific annotations like @GetMapping, @PostMapping, @PutMapping, or @DeleteMapping to define the type of request they handle.
Example: In our blog application, the PostController might have a method annotated with @PostMapping to handle the creation of a new blog post. This method would receive the request with the necessary data (e.g., title, content, author) and invoke the appropriate methods in the Model to create a new Post object.
Model Interaction: Inside the Controller methods, the Model component is interacted with to perform the necessary business logic. This may include tasks such as retrieving data from a database, validating input, updating entities, or applying transformations to the data.
Example: In our blog application, the Controller method for creating a new post would interact with the Model to persist the new Post object in a database. This could involve calling a service layer or repository to handle the data access and manipulation.
View Serialization: After the necessary operations are performed in the Model, the Controller prepares the response using the View component. In a Spring Boot REST web service, the View typically involves serializing the response data into a suitable format, such as JSON or XML, which can be easily consumed by the client.
Example: In our blog application, the Controller would utilize a serialization library (e.g., Jackson for JSON) to convert the Post object into JSON format. The serialized JSON response would include the post's title, content, author, and any other relevant details.
Response to Client: Finally, the serialized response data is sent back to the client as the HTTP response. The DispatcherServlet takes care of sending the response back to the client, and the client can then process the received data according to its requirements.
Overall, the MVC pattern in a Spring Boot REST web service helps in achieving a clear separation of concerns. The Model handles data and business logic, the Controller manages request processing and interaction with the Model, and the View component serializes the response data into a suitable format. This separation promotes modularity, code reusability, and easier maintenance of the application.
Коментарі