Comparing Java's Property Copying and Object Building Techniques
- CODING Z2M
- Sep 2, 2023
- 4 min read
Updated: Sep 4, 2023
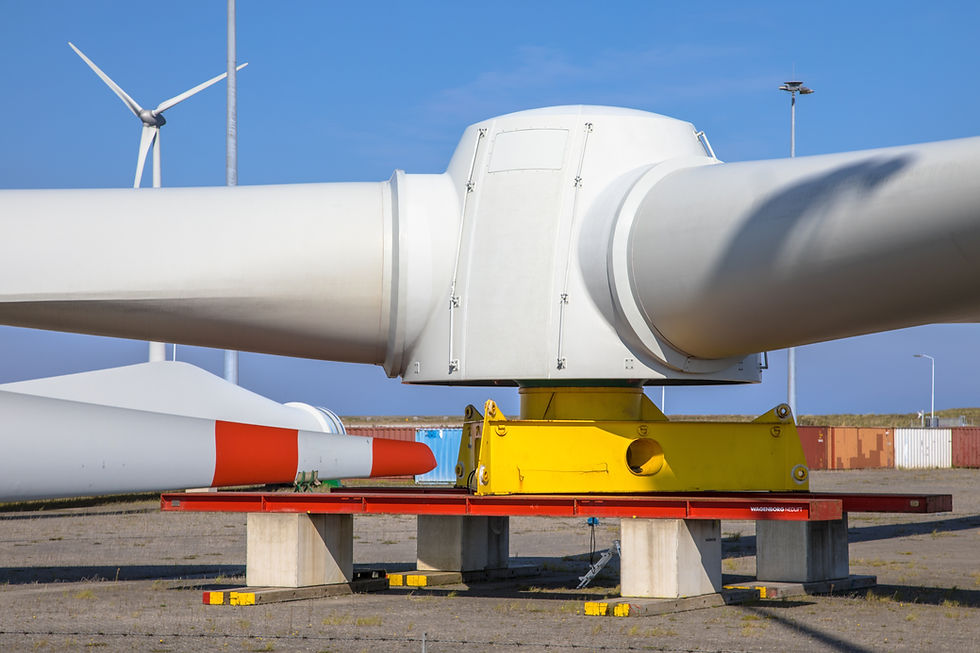
BeanUtils.copyProperties and Lombok's @Builder serve different purposes in Java and are used in different contexts.
BeanUtils.copyProperties:
BeanUtils.copyProperties is a method provided by Apache Commons BeanUtils library, and it is used for copying property values from one Java bean to another. This is typically done when you have two Java objects with similar properties, and you want to copy values from one object to another without writing boilerplate code for each property manually. It's often used for mapping DTOs (Data Transfer Objects) to entities or vice versa in data access layers.
Example: BeanUtils.copyProperties(sourceBean, targetBean);
Pros:
Helps reduce boilerplate code for copying properties.
Can be useful for mapping between different layers of an application, like DTOs and domain objects.
Cons:
It's not as type-safe as Lombok's @Builder.
Requires a third-party library (Apache Commons BeanUtils).
Lombok's @Builder:
Lombok is a Java library that helps reduce boilerplate code in Java classes by generating code at compile-time. @Builder is one of Lombok's annotations, and it generates a builder pattern for a class. The builder pattern is used to construct complex objects step by step with a fluent and readable API.
Example: @Builder
public class Person {
private String firstName;
private String lastName;
private int age;
}
Pros:
Provides a type-safe way to create complex objects with a fluent builder API.
Reduces the need for writing tedious constructor and setter methods.
Cons:
Primarily used for object creation and initialization, not for copying properties from one object to another.
What is creating objects with a fluent builder pattern using Lombok's @Builder?
Creating objects with a fluent builder pattern using Lombok's @Builder is a design pattern in Java that simplifies the process of constructing complex objects by providing a fluent and readable API. Lombok is a library that reduces boilerplate code in Java classes, and @Builder is one of its annotations that automatically generates the builder pattern code for a class.
Here's a step-by-step explanation of how to use Lombok's @Builder to create objects with a fluent builder pattern:
Annotate your class: Start by annotating your Java class with @Builder. This annotation tells Lombok to generate a builder class for your annotated class.
import lombok.Builder;
@Builder
public class Person {
private String firstName;
private String lastName;
private int age;
}
Create an instance using the builder: You can now use the generated builder to create instances of your class in a fluent and readable way. The builder provides methods to set the values of individual properties.
Person person = Person.builder()
.firstName("John")
.lastName("Doe")
.age(30)
.build();
In this example, you chain method calls to set the firstName, lastName, and age properties, and then call build() to create the Person object.
In summary, BeanUtils.copyProperties is used for copying property values between two Java beans, whereas Lombok's @Builder is used for creating objects with a builder pattern. The choice between them depends on your specific use case. If you need to copy properties, BeanUtils.copyProperties may be more suitable, while if you need to create objects with a fluent builder pattern, Lombok's @Builder is a better choice.
What is a Model Mapper in Java Spring Boot?
In Java Spring Boot applications, Model Mapper is a library or component that provides a convenient way to perform data mapping or transformation between Java objects, particularly domain entities, and data transfer objects (DTOs). In Spring Boot applications, it's common to have different representations of the same data for various purposes. For example, you might have a complex entity object representing a database record and a simpler DTO used for API responses or requests.
How does a Model Mapper work?
Model Mappers work by automatically copying data from one object (source) to another object (target) based on predefined rules or mappings. These mappings define how properties in the source object should be mapped to properties in the target object. The Model Mapper library handles the actual copying of data, reducing the need for manual and error-prone mapping code.
Here are some key aspects of how a Model Mapper typically works:
Mapping Configuration: Developers can define mapping rules in a configuration file or programmatically. These rules specify which fields in the source object correspond to fields in the target object.
Automatic Mapping: Once mappings are configured, the Model Mapper library can automatically map the source object's properties to the target object's properties. It can handle complex scenarios like nested objects or collections.
Type Conversion: Model Mappers often include type conversion capabilities, allowing them to handle data type differences between source and target objects.
Custom Mappings: Developers can override default mappings and provide custom mapping logic for specific scenarios when necessary.
When to Use a Model Mapper in Java Spring Boot:
DTO Transformation: Model Mappers are particularly useful when you need to transform domain entities into DTOs for API responses or vice versa. This helps in isolating the API layer from your domain model.
Database Entity Mapping: When working with JPA or other database technologies, Model Mappers can simplify the process of mapping database entities to DTOs or view models for efficient data retrieval and presentation.
Reducing Boilerplate Code: Model Mappers reduce the need for writing repetitive mapping code, resulting in cleaner and more maintainable code.
Complex Object Graphs: If your application deals with complex object graphs with nested objects or collections, a Model Mapper can streamline the mapping process.
Versioning and Data Transformation: When your application needs to support different versions of data models or data transformations, a Model Mapper can help manage these changes effectively.
In summary, a Model Mapper library is a valuable tool in Java Spring Boot applications, especially when dealing with data transformation between domain entities and DTOs or when simplifying the mapping of complex object structures. It helps reduce code duplication, improve maintainability, and ensure consistent data mapping throughout your application.
Key Differences BeanUtils.copyProperties, Lombok's @Builder & Model Mapper Model Mapper and BeanUtils.copyProperties are primarily focused on copying or mapping properties between objects, while Lombok's @Builder is focused on simplifying the instantiation of objects with specific properties.
Configuration: Model Mappers typically allow more extensive configuration and customization of mapping rules, whereas BeanUtils.copyProperties and @Builder offer simpler, less configurable property copying and object instantiation.
Use Cases:
Use Model Mapper or BeanUtils.copyProperties when you need to copy properties between objects with different structures or map between domain entities and DTOs.
Use Lombok's @Builder when you want to create instances of a class with a fluent and expressive syntax, especially in scenarios where there are many optional properties.
In summary, Model Mappers and BeanUtils.copyProperties are primarily used for copying or mapping properties between objects, while Lombok's @Builder focuses on simplifying object instantiation. The choice of which to use depends on your specific use case and the level of configurability and customization required.
Commenti