Spring Security-OAuth 2.0 Login: Securely Accessing Your Favorite Apps and Service
- CODING Z2M
- Sep 10, 2023
- 5 min read
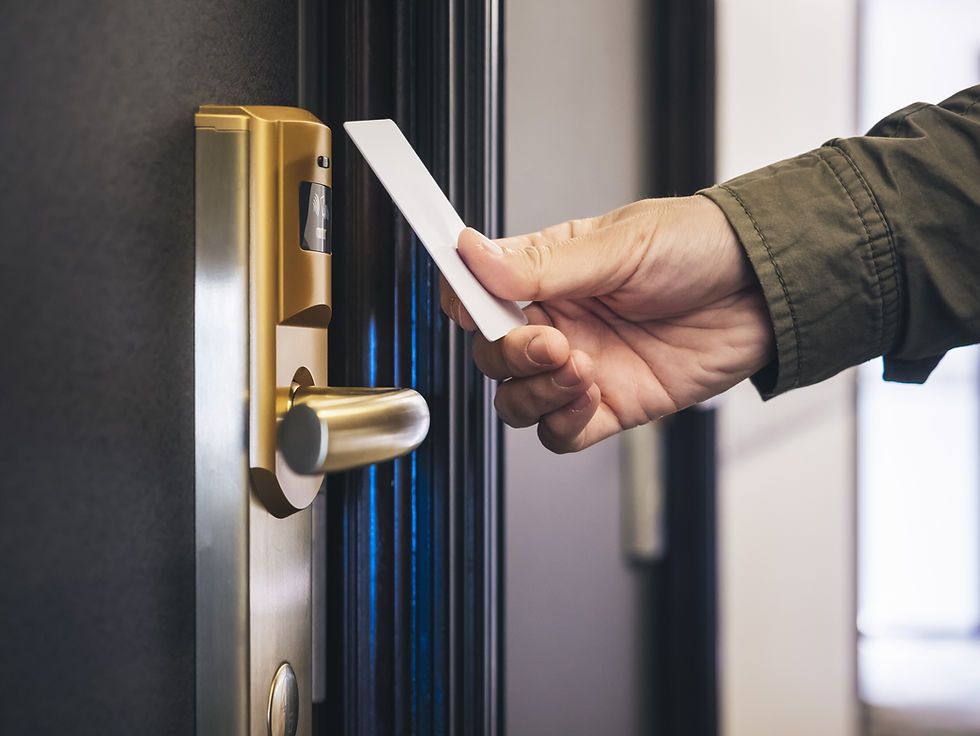
OAuth, which stands for "Open Authorization," is an open standard framework that allows third-party applications to access user data or perform actions on behalf of users without needing to know their credentials, such as usernames and passwords. It is commonly used for granting secure and limited access to resources on the web, especially in the context of APIs (Application Programming Interfaces).
OAuth is designed to enhance the security of user data and reduce the risk of exposing sensitive information. It does this by separating the authorization process from the actual sharing of user credentials. Instead of sharing a username and password directly with a third-party application, a user can grant limited access to their data or services through a process that involves authentication and authorization tokens. Refernces: Spring Security-OAuth2 Ref Video: OAuth2 & Spring boot 3 & Social login
OAuth2 providers:
Git & Setting up OAuth 2.0 on Google
Here's how OAuth typically works:
User Authorization: The user interacts with a client application (e.g., a mobile app or a website) and expresses the desire to access a resource protected by a service provider (e.g., social media data or cloud storage).
Request Token: The client application requests access to the user's data from the service provider. This request typically includes information such as the client's identity and the specific permissions it's requesting.
User Authentication: The user is redirected to the service provider's authentication system, where they log in or provide their consent for the requested access.
Authorization Grant: After successful authentication, the service provider issues an authorization grant (a temporary code) to the client application.
Token Exchange: The client application exchanges the authorization grant for an access token and, optionally, a refresh token. The access token is used to make authorized API requests on behalf of the user, and the refresh token is used to obtain a new access token when the current one expires.
Access Resources: The client application uses the access token to access the user's data or perform authorized actions on the service provider's platform.
OAuth is widely used by many online services, including social media platforms, cloud storage providers, and more, to enable third-party developers to create applications that interact with user accounts securely and without exposing sensitive login credentials.
Spring Boot makes it relatively easy to integrate OAuth2 providers into your application for authentication and authorization. Here are some popular OAuth2 providers you can integrate with Spring Boot:
Google: Google provides OAuth2 authentication services, which you can integrate into your Spring Boot application. Users can log in using their Google credentials.
GitHub: GitHub's OAuth2 authentication can be integrated into your Spring Boot application, enabling users to sign in with their GitHub accounts.
Facebook: Facebook offers OAuth2-based authentication. You can configure your Spring Boot application to allow users to sign in using their Facebook accounts.
Twitter: Twitter also supports OAuth2 authentication. You can integrate Twitter login functionality into your Spring Boot application.
LinkedIn: LinkedIn provides OAuth2-based authentication, allowing users to log in with their LinkedIn accounts.
Keycloak: Keycloak is an open-source identity and access management solution that provides OAuth2 support. You can integrate Keycloak with your Spring Boot application to manage user authentication and authorization.
Advantages of using OAuth2 in Spring Boot REST API OAuth2 is a widely used authentication and authorization framework that allows secure access to resources without revealing user credentials. In a Spring Boot application, OAuth2 can be used for various purposes, including:
User Authentication: OAuth2 can be used to enable user authentication in your Spring Boot application. Instead of managing user credentials and authentication logic in your application, you can delegate the authentication process to an external identity provider (IdP) like Google, Facebook, or GitHub. This allows users to log in using their existing accounts on these platforms.
Secure API Access: OAuth2 is commonly used to secure access to RESTful APIs. Your Spring Boot application can act as a resource server, and OAuth2 tokens are used to authorize client applications to access protected resources. This helps protect your APIs from unauthorized access.
Single Sign-On (SSO): OAuth2-based SSO allows users to log in once and access multiple applications without needing to log in again. Spring Boot applications can act as service providers that delegate authentication to an OAuth2-compatible identity provider (e.g., Keycloak, Okta) using OAuth2-based SSO protocols like OpenID Connect.
Third-Party Integration: If your Spring Boot application needs to interact with third-party services or APIs that require authentication, OAuth2 can be used to obtain access tokens and authenticate with those services securely.
Authorization: OAuth2 not only handles authentication but also provides mechanisms for fine-grained authorization. You can define access control policies based on scopes, roles, or permissions associated with OAuth2 tokens. This allows you to control what actions users or client applications can perform within your application or on protected resources.
Revoking Access: OAuth2 allows for token revocation, which is essential when you need to revoke access to resources or services. For example, if a user revokes access to their account on an OAuth2 identity provider, your Spring Boot application should also revoke the access tokens associated with that user.
Mobile and Single-Page Applications: OAuth2 is suitable for securing mobile apps and single-page applications (SPAs). These applications can obtain access tokens securely and use them to access protected resources, whether it's a REST API or a web service.
Security and Compliance: OAuth2 helps ensure that your Spring Boot application follows security best practices and compliance requirements. It provides mechanisms for token expiration, token refresh, and secure token transmission, enhancing the overall security of your application.
Scalability and Decoupling: By using OAuth2, your application can offload authentication and authorization to external identity providers. This decouples authentication from your application's core logic, making it more scalable and maintainable.
Logging and Auditing: OAuth2 transactions often generate logs and audit trails, which can be useful for monitoring and security analysis. You can leverage OAuth2 logs to track user and client application activity.
Defining OAuth2 in a Spring Boot application using Spring Security
@Configuration
@EnableWebSecurity
public class SecurityConfig {
@Bean
SecurityFilterChain securityFilterChain(HttpSecurity httpSecurity ) throws Exception {
return httpSecurity.authorizeHttpRequests(auth -> {
auth.anyRequest().authenticated();
})
.oauth2Login(oauth2 -> {
})
.build();
} }
@Configuration and @EnableWebSecurity annotations:
@Configuration indicates that this class is a Spring configuration class and can be used to configure beans and application settings.
@EnableWebSecurity is a Spring Security annotation that enables web-based security within your application.
SecurityConfig class:
This is the main configuration class for defining security-related settings in your Spring Boot application.
@Bean annotation:
This annotation is used to define a Spring bean within this configuration class.
SecurityFilterChain bean definition:
SecurityFilterChain is a construct used to define a chain of filters that Spring Security should apply to incoming HTTP requests to enforce security rules.
securityFilterChain method:
This method defines the SecurityFilterChain bean.
It takes an HttpSecurity object as a parameter. The HttpSecurity object is used to configure security rules for various aspects of your application.
httpSecurity.authorizeHttpRequests(auth -> { ... }):
This part of the code configures how incoming HTTP requests should be authorized.
authorizeHttpRequests is a method that takes an auth parameter, which is a lambda expression used to specify authorization rules.
In this case, it's using auth.anyRequest().authenticated(), which means that any HTTP request (anyRequest) must be authenticated. In other words, the user must be logged in to access any part of the application.
.oauth2Login(oauth2 -> { ... }):
This part of the code configures OAuth2 login support. It's empty in your code, so it doesn't specify any specific OAuth2 provider or settings. You would typically fill this in with OAuth2 configuration specific to your application.
.build():
Finally, .build() is called on the HttpSecurity object to build the configuration. This effectively finishes the security configuration.
In summary, this code configures Spring Security to enforce authentication for all incoming HTTP requests, meaning that users must be logged in to access any part of the application. Additionally, it sets up the infrastructure for OAuth2 login, but the specific OAuth2 configuration is missing and would need to be added separately to enable OAuth2-based authentication.
Comments