Spring Boot Questions & Answers
- CODING Z2M
- Apr 19, 2023
- 6 min read
Updated: Jul 15, 2023
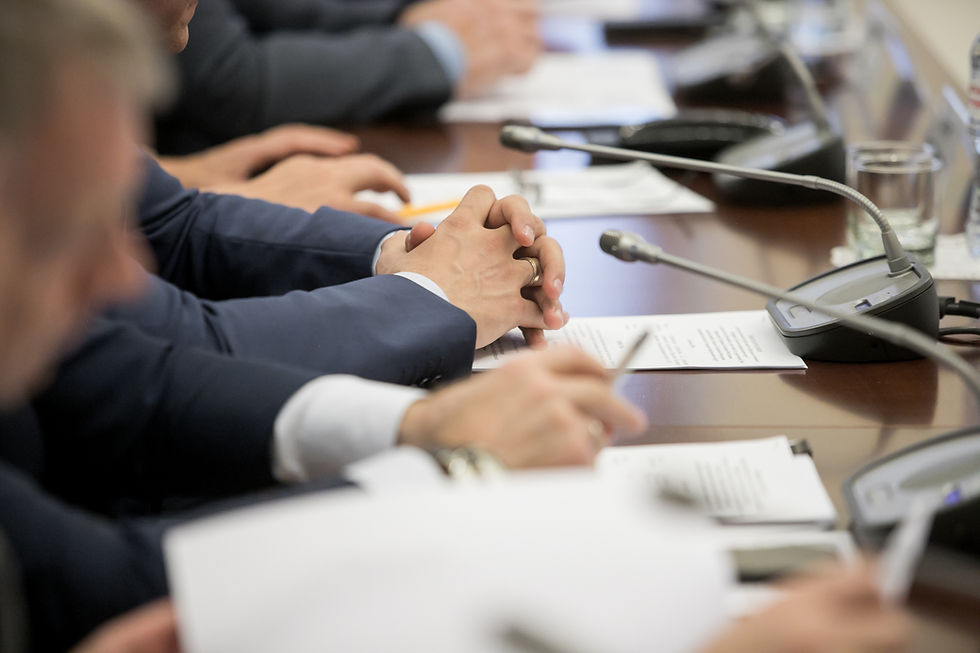
1. Why do we use @Autowired at the constructor level?
Answer: We use @Autowired at the constructor level in Spring to inject dependencies into a class through its constructor. This approach is also known as constructor injection.
Constructor injection has several advantages over other forms of dependency injection, such as field or setter injection. First, it ensures that all required dependencies are provided when the object is created, which can help prevent runtime errors due to missing dependencies. Second, it makes the class more testable by allowing dependencies to be easily swapped out with mock objects during testing. Finally, it makes the code more readable by making the dependencies explicit in the class's constructor signature.
Here is an example of using @Autowired at the constructor level: @Service
public class MyService {
private final MyRepository repository;
@Autowired
public MyService(MyRepository repository) {
this.repository = repository;
}
public void doSomething() {
// use the injected repository
}
}
In this example, the MyRepository dependency is injected into the constructor of MyService using @Autowired. This ensures that the MyRepository instance is available when the MyService instance is created, and that it is not null when the doSomething() method is called.
Note that when using constructor injection, the @Autowired annotation is required on the constructor parameter(s) to indicate to Spring which dependencies should be provided. Also, if there is only one constructor in the class, the @Autowired annotation can be omitted, as Spring will automatically use it for dependency injection.
2. Why do we use @Autowired at the variable level?
Answer: The @Autowired annotation is commonly used in Spring to inject dependencies into a class. It is typically used at the class level to mark fields, methods or constructors that need to be injected with dependencies.
However, it is also possible to use the @Autowired annotation at the variable level. When used at the variable level, the @Autowired annotation injects the dependency directly into the variable, without the need for a setter method.
Using @Autowired at the variable level is particularly useful when you have a class with many dependencies, and you want to avoid writing multiple setter methods or a long constructor with many parameters.
Here is an example of using @Autowired at the variable level:
@Component
public class MyService {
@Autowired
private MyRepository repository;
public void doSomething() {
// use the injected repository
}
}
In this example, the MyRepository dependency is injected directly into the repository field using @Autowired. This makes the code more concise and easier to read.
However, it's worth noting that using @Autowired at the variable level can make the code less testable because you cannot easily swap out the dependency with a mock object for testing purposes. For this reason, it's generally recommended to use constructor injection instead of field injection, and only use field injection sparingly when necessary.
3. What is Spring Boot?
Answer: Spring Boot is an open-source Java framework used to build standalone, production-ready Spring-based applications. It is built on top of the Spring Framework and provides several features, such as auto-configuration, embedded servers, and metrics, which make it easier to develop and deploy Spring-based applications.
4. What are some of the advantages of using Spring Boot? Answer: Some of the advantages of using Spring Boot include:
Simplified configuration: Spring Boot provides auto-configuration, which automatically configures the application based on its dependencies, making it easier to get started.
Embedded servers: Spring Boot includes several embedded servers, such as Tomcat and Jetty, which make it easier to develop and deploy web applications.
Dependency management: Spring Boot manages dependencies through its built-in dependency management system, which ensures that all dependencies are compatible and up-to-date.
Production-ready: Spring Boot is designed to be production-ready, with features such as health checks, metrics, and externalized configuration.
5. What is auto-configuration in Spring Boot?
Answer: Auto-configuration is a feature in Spring Boot that automatically configures the application based on its dependencies. Spring Boot includes several auto-configuration classes, which provide sensible defaults for various Spring components, such as data sources, JPA, and security. Auto-configuration can be customized or disabled by providing a custom configuration class.
6. What is Spring Boot Actuator? Answer: Spring Boot Actuator is a sub-project of Spring Boot that provides several production-ready features, such as health checks, metrics, and monitoring, for Spring-based applications. It includes several endpoints, which provide information about the application's health, metrics, and other operational aspects. Actuator can be configured and customized to suit the application's needs.
7. What is the difference between @Component, @Service, and @Repository annotations in Spring Boot? Answer: All three annotations are used to indicate that a class is a Spring-managed component, but they have slightly different semantic meanings.
@Component: This is a general-purpose annotation used to indicate that a class is a Spring-managed component.
@Service: This annotation is used to indicate that a class is a Spring-managed service component, typically used for business logic.
@Repository: This annotation is used to indicate that a class is a Spring-managed repository component, typically used for data access.
8. What is Spring Boot Starter? Answer: A Spring Boot starter is a set of pre-configured dependencies that provide a specific functionality, such as database access or web development. Starters include a set of transitive dependencies and a default configuration, making it easy to get started with a specific functionality. Starters can be included in the application's dependencies, or custom starters can be created for specific use cases. 9. Give one example for auto-configuration in Spring Boot? Answer: Suppose you want to use Spring Data JPA in your Spring Boot application to access a database. Normally, you would need to configure several Spring components, such as a data source, a transaction manager, and an entity manager factory, in order to use Spring Data JPA.
However, with Spring Boot's auto-configuration, you can simply add the following dependency to your pom.xml file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency> This dependency includes several transitive dependencies, including a JDBC driver, a Hibernate implementation, and a connection pool. It also provides a default configuration for the required Spring components, based on sensible defaults and best practices.For example, the spring-boot-starter-data-jpa dependency includes a default configuration for a data source, which is typically sufficient for most use cases. In summary, with Spring Boot's auto-configuration, you can easily get started with Spring Data JPA without needing to manually configure all the required Spring components. This saves time and reduces the chances of errors in your configuration
10. Difference between spring framework and spring boot?
Answer: The Spring Framework and Spring Boot are two related technologies that are widely used in Java web application development. Here are some key differences between the two:
Configuration: The Spring Framework requires explicit configuration of all components, whereas Spring Boot provides auto-configuration and sensible defaults that can reduce the amount of manual configuration required.
Dependencies: The Spring Framework relies on external dependencies to provide functionality, whereas Spring Boot includes a set of pre-configured dependencies that provide a jump start for common use cases.
Packaging and Deployment: The Spring Framework applications typically need to be packaged as WAR or JAR files and deployed to a web container, whereas Spring Boot applications can be packaged as standalone JAR files and run as executable Java applications.
Convention over Configuration: Spring Boot emphasizes convention over configuration, which means that it provides sensible defaults that allow developers to focus on writing business logic, rather than worrying about configuring the application.
Rapid Development: Spring Boot is designed for rapid development, with features such as hot-reloading and the ability to run the application directly from an IDE, making it easier to iterate quickly.
Overall, Spring Boot is built on top of the Spring Framework, and provides a simpler and more opinionated way of building Spring applications, with a focus on rapid development and ease of deployment.
11. How incoming HTTP requests in spring are mapped to specific methods and return the response directly as JSON or XML data?
Answer: In Spring, incoming HTTP requests are mapped to specific methods using a combination of annotations and configuration. The most commonly used annotations for mapping requests to methods are @RequestMapping, @GetMapping, @PostMapping, @PutMapping, @DeleteMapping, and @PatchMapping.
The @RequestMapping annotation is used to map a URI path and HTTP method to a specific method in a Spring controller. For example, the following code maps a GET request to the /users path to the getUser method in a controller: @RequestMapping(method = RequestMethod.GET, value = "/users")
@ResponseBody
public List<User> getUsers() {
// logic to retrieve list of users
return userList;
} The @GetMapping annotation is a shortcut for mapping a GET request to a specific URI path. For example, the following code is equivalent to the previous example: @GetMapping("/users")
@ResponseBody
public List<User> getUsers() {
// logic to retrieve list of users
return userList;
}
Similarly, @PostMapping, @PutMapping, @DeleteMapping, and @PatchMapping are shortcuts for mapping POST, PUT, DELETE, and PATCH requests to specific URI paths.
When a method is called to handle an incoming request, Spring will automatically convert the return value to JSON or XML data if the @ResponseBody annotation is present. This annotation indicates that the return value should be serialized and returned directly as the response body.
For example, in the previous code examples, the @ResponseBody annotation is used to indicate that the List<User> should be returned as the response body in JSON or XML format.
Spring also provides a variety of tools and libraries for customizing the serialization and deserialization of data, such as the Jackson library for JSON serialization and the JAXB library for XML serialization.
Overall, Spring's mapping and serialization capabilities provide a powerful and flexible platform for developing RESTful web services that can easily handle incoming HTTP requests and return the response directly as JSON or XML data.
Comments