Unveiling the Power of Server Side & Client Side Components in Next.js
- CODING Z2M
- Jun 20, 2023
- 3 min read
Updated: Jun 21, 2023
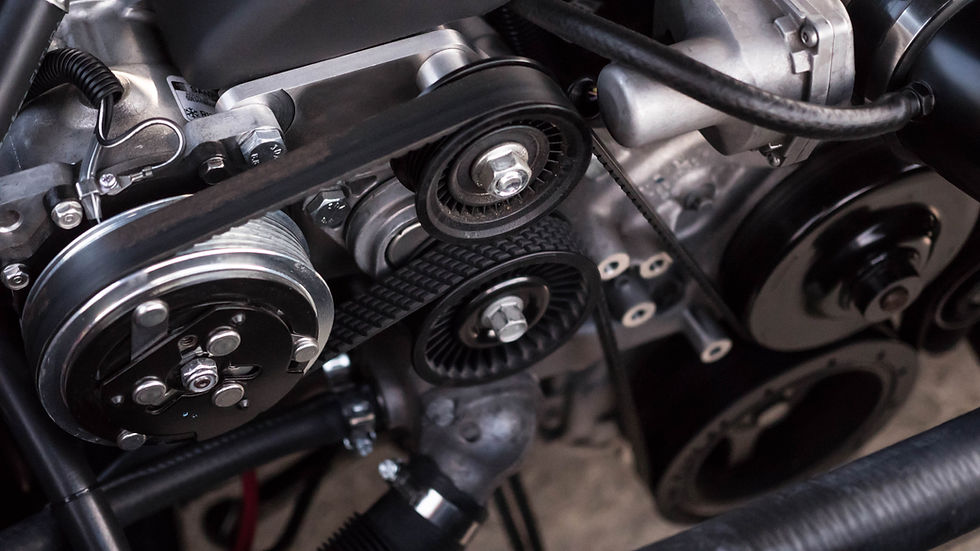
In the world of web development, optimizing performance and delivering exceptional user experiences are top priorities. Next.js, a popular React framework, offers a powerful solution with its server side and client side components. In this blog post, we will explore the benefits, use cases, and implementation of server side and client side components in Next.js, enabling you to take your web development skills to new heights.
Leveraging Server Side Components in Next.js
Server Side Components take SSR a step further by enabling you to selectively render components on the server. With Next.js Server Side Components, you can define parts of your application that require server rendering and load them dynamically. This allows for optimized performance by rendering only the necessary parts on the server, while still benefiting from the reactivity and interactivity of client-side rendering.
Server side components in Next.js, allow you to fetch data and perform server-side rendering for specific parts of a page. You can create server side components by exporting a function from a file in the pages/api directory.
Benefits of Server Side Components:
Server Side Components bring several advantages to your Next.js applications. Firstly, they enable faster time to interactive (TTI) by rendering critical components on the server and sending them to the client for immediate interaction. Additionally, they provide improved accessibility and SEO, as all relevant content is available in the initial HTML response. Server Side Components also facilitate code reusability, as you can encapsulate complex server-rendered logic within components.
Real-World Use Cases: you can use them to render personalized user interfaces, fetch and display server-side data, or handle complex server-side logic that requires pre-rendering. // pages/api/server-side-component.js
export default function ServerSideComponent() {
// Perform server-side logic and return the data
const serverData = fetchData();
return (
<div>
<h1>{serverData.title}</h1>
<p>{serverData.description}</p>
</div>
);
}
Conclusion: Server Side Components in Next.js provide an effective approach to achieve a balance between server-side rendering and client-side interactivity. By leveraging the power of Next.js and selectively rendering components on the server, you can deliver blazing-fast and highly interactive web experiences.
Enhancing Interactivity with Client Side Components Client Side Components in Next.js enable you to create interactive elements that are rendered and managed on the client-side. These components can handle dynamic content, user interactions, and state changes without requiring a full page reload. These components are bundled with the initial page load and can be updated without full page refresh.
Benefits of Client Side Components: Client Side Components offer numerous advantages for Next.js applications. They enhance user interactivity by enabling real-time updates and responsiveness. They also improve performance by reducing the amount of data sent between the server and client, resulting in faster page rendering. Additionally, Client Side Components facilitate a seamless user experience by providing smooth transitions and dynamic content loading.
Implementing Client Side Components in Next.js:
To leverage Client Side Components in Next.js, you can utilize the built-in features of React, such as hooks and context, to manage component state and handle user interactions.
Real-World Use Cases:
You can use them to create interactive forms, implement live chat functionality, build real-time data visualization components, or create interactive maps with dynamic markers.
// components/ClientSideComponent.js
import { useState } from 'react';
export default function ClientSideComponent() {
const [count, setCount] = useState(0);
const incrementCount = () => {
setCount(count + 1);
};
return (
<div>
<h2>Client Side Component</h2>
<p>Count: {count}</p>
<button onClick={incrementCount}>Increment</button>
</div>
);
}
Conclusion: Client Side Components in Next.js empower developers to create rich and interactive web applications that engage users with dynamic content and real-time updates. By harnessing the power of React and Next.js, you can build responsive and highly interactive UI components that provide a seamless user experience.
Combining server side and client side components for optimal performance and flexibility. This approach allows you to pre-render content on the server and then hydrate it with client-side interactivity.
Comments