Unleash Lightning-Fast Speeds: Next.js Performance Optimization
- CODING Z2M
- Jun 22, 2023
- 4 min read
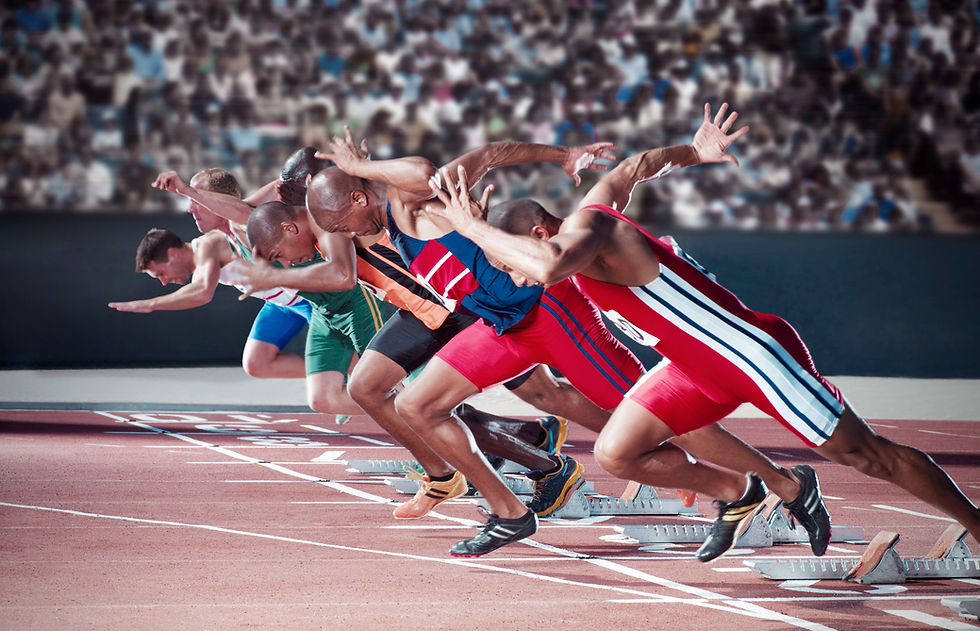
Next.js Performance Optimization: In today's fast-paced digital world, website and application performance is crucial for delivering an exceptional user experience. With Next.js, a powerful React-based framework, developers have access to a range of optimization techniques to ensure lightning-fast applications. In this comprehensive guide, we will explore the best practices and tips for optimizing performance in Next.js. Let's dive in and unlock the potential of a high-performing Next.js application.
1. Use Server-Side Rendering (SSR) Wisely:
Next.js offers built-in server-side rendering capabilities, which greatly enhance initial load times and SEO performance. However, it's essential to use SSR judiciously to strike a balance between performance and functionality. Consider the following:
a) Identify Critical Content: Render only the most critical content on the server to reduce the initial payload. Fetch non-critical content asynchronously on the client-side to improve performance.
b) Implement Incremental Static Regeneration (ISR): For pages with frequently changing data, use ISR to update the content at predetermined intervals. This approach ensures a balance between fresh content and optimal performance.
2. Leverage Code Splitting:
Next.js provides automatic code splitting, which allows you to split your JavaScript bundles into smaller, more manageable chunks. This technique enhances performance by loading only the required code for each specific page or component. Follow these practices:
a) Identify Code Dependencies: Analyze your application to identify code dependencies that can be split into separate chunks. This enables lazy loading and improves initial load times.
b) Use Dynamic Imports: Utilize Next.js's dynamic imports feature to load components and libraries on demand. This approach significantly reduces the initial bundle size and improves performance.
3. Optimize Image Loading:
Images can significantly impact page load times. Next.js offers built-in optimizations to ensure efficient image loading:
a) Use the Next.js Image Component: Replace traditional HTML image tags with Next.js's Image component. It automatically optimizes and resizes images based on the device and screen size, resulting in faster load times.
b) Enable Image Optimization: Configure Next.js to automatically optimize and compress images during the build process. This feature reduces image file sizes without compromising quality.
c) Lazy Load Images: Implement lazy loading for images that appear below the fold. By loading images only when they enter the view-port, you reduce the initial page load and improve perceived performance.
Follow these steps to enable automatic image optimization & implement lazy loading in your Next.js app:
Inside the next.config.js file, add the following code snippet to configure image optimization:
const withImages = require('next-images');
module.exports = withImages({
// Other Next.js configuration options...
// Image Optimization Configuration
images: {
domains: ['example.com'], // Add your domain(s) here
deviceSizes: [320, 640, 750, 828, 1080, 1200, 1920, 2048], // Adjust according to your project's requirements
},
// Lazy Loading Configuration reactStrictMode: true, // Enable strict mode
});
In the images configuration section, you can customize the optimization settings:
domains: Specify the domains from which your app will fetch images. Add the relevant domain(s) inside the array. This ensures that Next.js properly optimizes and serves images from these domains.
deviceSizes: Define an array of device widths (in pixels) for which Next.js will automatically generate optimized images. Adjust the sizes based on your project's needs.
4. Implement Client-Side Data Fetching:
Next.js enables client-side data fetching through its powerful APIs like getStaticProps, getServerSideProps, and useSWR. Utilize these capabilities to enhance performance:
a) Caching and Prefetching: Implement caching mechanisms using Next.js's data fetching APIs to avoid unnecessary network requests. Use prefetching techniques to fetch data in advance based on user interactions.
b) Optimize Data Fetching Strategies: Choose the appropriate data fetching strategy based on your application's requirements. Use static generation (getStaticProps) for content that rarely changes, server-side rendering (getServerSideProps) for dynamic data, and client-side rendering (useSWR) for real-time data updates.
5 .Minify and Compress Assets:
Reducing the size of your JavaScript, CSS, and other assets is crucial for optimizing performance. Follow these practices:
a) Minify JavaScript and CSS: Utilize Next.js's built-in minification capabilities to remove unnecessary characters, comments, and whitespace from your code. This reduces file sizes and improves load times. Inside the next.config.js file, you can configure the built-in minification capabilities for JavaScript and CSS using the minify property. Add the following code snippet:
module.exports = {
// Other Next.js configuration options...
minify: {
minifyJS: true,
minifyCSS: true,
},
};
By setting minifyJS and minifyCSS to true, you enable the minification of JavaScript and CSS files, respectively.
6. Implement Client-Side Rendering (CSR) for Interactivity (continued): While SSR is beneficial for initial page loads and SEO, certain interactive elements can be rendered on the client-side for a smoother user experience. Consider the following:
a) Identify Interactive Components: Determine which components require real-time updates or user interactions. These components can be rendered on the client-side using Next.js's client-side rendering capabilities.
b) Utilize React's useEffect Hook: Leverage React's useEffect hook to initiate client-side rendering and handle component updates. This allows you to fetch data or perform actions asynchronously on the client-side.
Conclusion:
Optimizing performance in Next.js applications is crucial for delivering fast, responsive, and user-friendly experiences. By following the best practices and tips outlined in this guide, you can ensure that your Next.js applications are finely tuned for optimal performance. From leveraging server-side rendering to implementing code splitting, image optimization, and client-side data fetching, each technique plays a vital role in enhancing performance. Regularly monitor and optimize your application's performance to maintain a high-performance standard. With these practices in mind, you'll be well-equipped to create high-performing Next.js applications that leave users impressed.
Comments