Next.js MongoDB API Routes - Build Robust Back-End Functionality in Minutes
- CODING Z2M
- Jul 3, 2023
- 5 min read
Updated: Sep 7, 2023
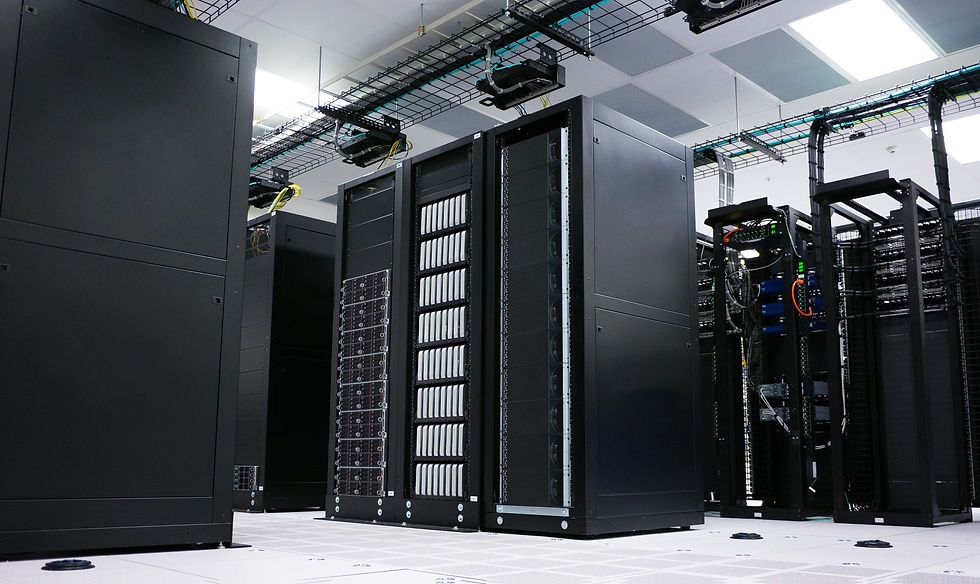
In the world of web development, Next.js and MongoDB are two powerful technologies that, when combined, offer tremendous potential for building scalable and feature-rich applications. In this article, we will explore the integration of Next.js with MongoDB API routes, and how this combination can empower developers to create robust backend functionality. Let's dive in!
Understanding Next.js API Routes:
Next.js API routes provide a serverless architecture for handling API requests. With Next.js, you can effortlessly define custom API endpoints that can be accessed directly from the client-side code. This eliminates the need for setting up a separate backend server and simplifies the development process.
Introducing MongoDB:
MongoDB is a popular NoSQL database known for its flexibility and scalability. It stores data in a JSON-like format, making it easy to work with in modern JavaScript applications. MongoDB's document-oriented nature and powerful querying capabilities make it an excellent choice for storing and retrieving data in Next.js applications.
Integrating Next.js with MongoDB API Routes:
By combining Next.js API routes with MongoDB, developers can create a seamless connection between their frontend and backend. This integration allows you to perform CRUD (Create, Read, Update, Delete) operations on your MongoDB data using the API endpoints defined in Next.js.
Setting up MongoDB Connection:
To get started, establish a connection to your MongoDB database within the Next.js API routes. Utilize the MongoDB Node.js driver or an ORM like Mongoose to handle the connection and interact with the database. Ensure you securely manage credentials and handle error scenarios effectively.
Implementing CRUD Operations:
With the connection established, you can proceed to implement CRUD operations using the API routes. Create endpoints for fetching data, adding new records, updating existing documents, and deleting data from MongoDB. Leverage the expressive querying capabilities of MongoDB to filter and sort data based on your application's requirements.
Establishing a MongoDB connection using Mongoose:
import mongoose from "mongoose"; let isDbConnected = false; export const dbConnection = async () =>{ mongoose.set('strictQuery', true); if(isDbConnected) { console.log("Mongo database is connected!"); return; } try { await mongoose.connect(process.env.MONGODB_URI, { dbName: "nextjs13_event_management", useNewUrlParser: true, useUnifiedTopology: true, }) isDbConnected= true; console.log("MongoDB Connected!") } catch(error) { console.log("MongoDb Connection Error!"); } }
The provided code snippet demonstrates a function for establishing a MongoDB connection using Mongoose, a popular MongoDB object modeling tool for Node.js. Here's a breakdown of how it works:
Importing the necessary dependencies: import mongoose from "mongoose"; The code begins by importing the mongoose library, which provides the necessary tools for interacting with MongoDB in a Node.js environment.
Defining a variable to track the connection status: let isDbConnected = false; Here, a boolean variable isDbConnected is initialized as false to keep track of whether the MongoDB database connection has been established.
Exporting the database connection function: export const dbConnection = async () => { // Function code } The dbConnection function is exported as a named export, making it accessible for other modules to use.
Setting strict query mode for Mongoose: mongoose.set('strictQuery', true); This line configures Mongoose to use strict query mode, which helps in detecting and reporting any potential issues with query syntax or data validation.
Checking if the database is already connected: if (isDbConnected) { console.log("Mongo database is connected!"); return; } Before establishing a new database connection, the function checks if the isDbConnected flag is already set to true. If it is, the function logs a message stating that the MongoDB database is already connected and returns, skipping the connection logic.
Establishing the database connection: try { await mongoose.connect(process.env.MONGODB_URI, { dbName: "nextjs13_event_management", useNewUrlParser: true, useUnifiedTopology: true, }); isDbConnected = true; console.log("MongoDB Connected!"); } catch (error) { console.log("MongoDb Connection Error!"); }
If the isDbConnected flag is false, the function attempts to connect to the MongoDB database using the mongoose.connect method. It uses the value of the MONGODB_URI environment variable to specify the MongoDB connection URL. Additional options, such as the database name, dbName, and connection settings, useNewUrlParser and useUnifiedTopology, are provided to ensure proper connection establishment. If the connection is successful, the isDbConnected flag is set to true, indicating that the database is connected. A success message is logged to the console. If an error occurs during the connection attempt, an error message is logged instead.
Overall, this code snippet establishes a connection to a MongoDB database using Mongoose and ensures that the connection is only established once, preventing unnecessary connection attempts.
Creation of a Mongoose model Example: The provided code snippet showcases the creation of a Mongoose model for a "User" schema in a MongoDB database. Let's break down how it works:
Importing the necessary dependencies:
import { Schema, model, models } from "mongoose";
The code imports the required modules from the mongoose library, including Schema, model, and models. These modules provide the tools necessary to define and work with MongoDB schemas and models.
Defining the User schema:
const UserSchema = new Schema({
email: {
type: String,
unique: [true, "Email is already registered!"],
required: [true, "Email is required!"],
},
username: {
type: String,
required: [true, "Username is required!"],
match: /^[a-zA-Z0-9_]+$/,
},
image: {
type: String,
}
})
The code defines a UserSchema using the Schema class provided by Mongoose. It specifies the structure and validation rules for the User schema. In this case, the schema includes fields for email, username, and image.
The email field is of type String and is required (required: true). It also has a unique constraint, ensuring that no two users can have the same email address. If a duplicate email is provided, an error message "Email is already registered!" will be shown.
The username field is of type String and is required. It also includes a regular expression (match) pattern, allowing only alphanumeric characters and underscores.
The image field is of type String and does not have any validation constraints.
Creating the User model:
const User = models.User || model("User", UserSchema);
Here, the code defines the User model using the model function provided by Mongoose. It checks if the User model already exists in the models object. If it does, it retrieves the existing model. Otherwise, it creates a new model named "User" using the UserSchema defined earlier.
Exporting the User model: export default User; Finally, the User model is exported as the default export of the module, making it accessible for other parts of the application to use.
In summary, this code creates a Mongoose model for the "User" schema, defining the structure and validation rules for the User object in a MongoDB database. It allows for easy interaction with user data, such as creating, reading, updating, and deleting user documents within the MongoDB collection associated with the User model. Conclusion:
Next.js MongoDB API routes offer an exceptional combination for building powerful and scalable web applications. By seamlessly integrating Next.js with MongoDB, developers can create robust backend functionality, perform CRUD operations, and harness real-time capabilities. The flexibility and scalability of these technologies enable the creation of feature-rich applications that deliver exceptional user experiences. So, why not dive into the world of Next.js MongoDB API routes and unlock the true potential of your web development projects?
Comments