Skyrocket Your App's Security and User Engagement-Next.js OAuth authentication
- CODING Z2M
- Jul 2, 2023
- 5 min read
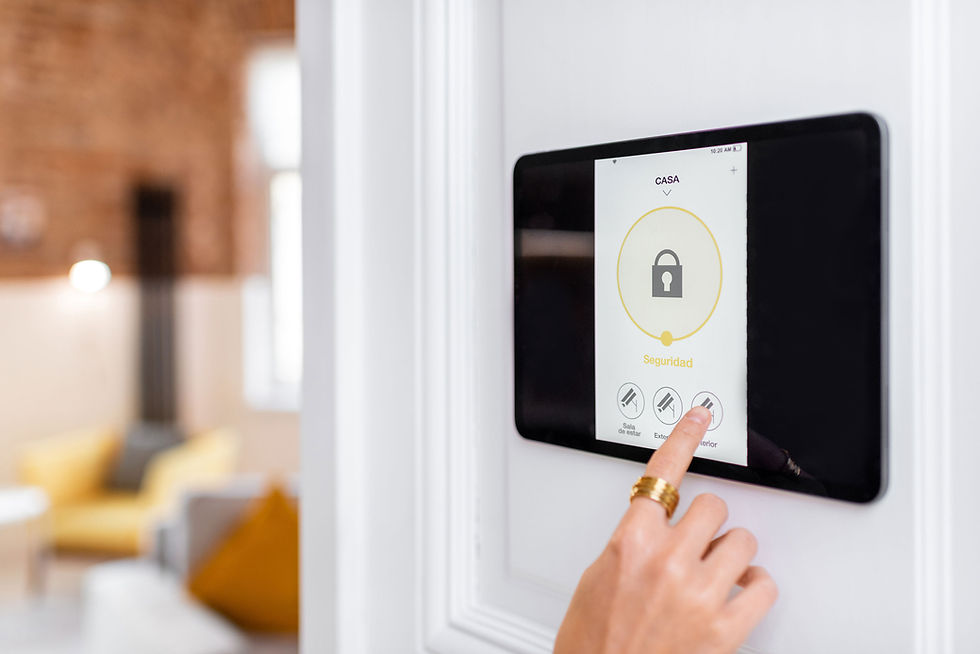
In today's digital landscape, implementing a robust authentication system is crucial for web applications. OAuth-based authentication offers a seamless and secure solution, allowing users to log in using their existing credentials from popular third-party services like Google. In this blog post, we will explore how to implement OAuth-based authentication in Next.js, leveraging the power of Google as the authentication provider.
Benefits of OAuth-Based Authentication
OAuth-based authentication provides several benefits for both developers and users. Let's take a closer look at some of these advantages:
Simplified User Experience: With OAuth, users can log in to your Next.js application using their Google accounts without the need to create a separate username and password. This eliminates the hassle of remembering yet another set of credentials, enhancing user convenience.
Enhanced Security: By leveraging OAuth, your application offloads the responsibility of password management and storage to the third-party authentication provider, such as Google. This reduces the risk of security vulnerabilities, such as password leaks or weak user passwords.
Increased Trust and User Adoption: Integrating OAuth with a trusted provider like Google instills confidence in users as they can rely on their existing Google credentials. This can lead to higher user adoption rates and increased trust in your application.
Step-by-Step Implementation Guide
Now, let's dive into the step-by-step process of implementing OAuth-based authentication in Next.js with Google as the authentication provider:
Step 1: Set Up a Google Developer Account
Create a project on the Google Developers Console.
Configure the project's OAuth consent screen and obtain the necessary credentials (Client ID and Client Secret).
Step 2: Install Required Packages
Use npm or yarn to install the required packages, such as next-auth and bcrypt.
Step 3: Create the Next.js Authentication Provider
Set up the authentication provider using next-auth by configuring the pages/api/auth/[...nextauth].js file.
Specify the Google OAuth provider details, including the Client ID and Client Secret obtained in Step 1.
Step 4: Create the Login Page
Build a login page where users can initiate the OAuth login process.
Add a button that triggers the authentication flow and redirects users to the Google login page.
Step 5: Handle the Callback
Create a callbacks to handle the callback after successful authentication.
Retrieve the user information and generate a session token using next-auth.
Example of an authentication API handler in Next.js:
// contains the logic for handling authentication-related API requests. import NextAuth from "next-auth/next"; import GoogleProvider from "next-auth/providers/google"; import { dbConnection } from "@/utility/mongodb"; import User from "@/models/user"; const authenticationHandler = NextAuth({ providers: [ GoogleProvider({ clientId: process.env.GOOGLE_ID, clientSecret: process.env.GOOGLE_CLIENT_SECRET, }) ], callbacks: { async session({session}) { const userSession = await User.findOne({ email: session.user.email }) session.user.id = userSession._id.toString(); return session; }, async signIn({profile}) { try{ await dbConnection(); // if the user already exists... const userExists = await User.findOne({ email: profile.email }) // if user is not existed... if(!userExists){ await User.create({ email: profile.email, username: profile.name.replace(" ", "").toLowerCase(), image: profile.picture }) } return true; } catch(error){ console.log(error); return false; } } } }) export {authenticationHandler as GET, authenticationHandler as POST}; The code snippet provided is an example of an authentication API handler in Next.js. It handles authentication-related API requests, such as signing in and managing sessions.
The code imports the necessary dependencies, including NextAuth, the GoogleProvider from next-auth/providers, and other utility files. It also imports the User model and establishes a database connection using dbConnection.
The authenticationHandler function is created using NextAuth, which takes an object as its argument. Within the object, the providers array specifies the authentication provider, in this case, GoogleProvider, and includes the client ID and client secret obtained from the environment variables.
The callbacks object defines two callback functions, session and signIn. The session callback is responsible for modifying the session object and adding the user ID from the database. It retrieves the user session based on the user's email and updates the session object with the corresponding user ID.
The signIn callback is executed when a user signs in. It first establishes a database connection using dbConnection. It checks if the user already exists in the database based on their email. If the user does not exist, it creates a new user entry using the profile information obtained from the authentication provider (in this case, Google). The user's email, username (derived from the profile name), and profile picture are stored in the User model.
The authenticationHandler function is exported as both GET and POST, indicating that this API route supports both HTTP methods.
Overall, this code snippet demonstrates how to configure authentication in Next.js using NextAuth and the GoogleProvider. It handles session management, user creation, and database interactions to enable OAuth-based authentication with Google.
Usage of the SessionProvider component from next-auth/react - Centralizing session management:
"use client"; import { SessionProvider } from 'next-auth/react' const UserSessionProvider = ({children, session}) => { return ( <SessionProvider session={session}> {children} </SessionProvider> ) } export default UserSessionProvider;
The code snippet provided demonstrates the usage of the SessionProvider component from next-auth/react and the creation of a custom UserSessionProvider component.
The UserSessionProvider component is defined as a functional component that takes two props: children and session. The children prop represents the child components that will be wrapped by the SessionProvider, and the session prop is the session object obtained from NextAuth.
Within the UserSessionProvider component, the SessionProvider component is used to wrap the children. The SessionProvider component receives the session prop, which contains the session object, and makes it accessible to all child components wrapped within it.
The UserSessionProvider component acts as a custom wrapper around the SessionProvider, providing a layer of abstraction and potential customization options if needed.
Finally, the UserSessionProvider component is exported as the default export, making it available for import and use in other parts of the application.
Overall, this code snippet demonstrates how to create a custom wrapper component, UserSessionProvider, that utilizes the SessionProvider from next-auth/react to provide the session object to its child components. This approach allows for centralized session management and simplifies the process of accessing and utilizing the session data within the application.
RootLayout component provides a common layout structure - layout.js:
import './globals.css' import { Inter } from 'next/font/google' const inter = Inter({ subsets: ['latin'] }) import UserSessionProvider from '@/components/UserSessionProvider' export const metadata = { title: 'Event Management App', description: 'Next.js MongoDB Event Management App', } // 'children' property is used to render the content that will be placed within the layout. export default function RootLayout({ children }) { return ( <html lang="en"> <body className={inter.className}> <UserSessionProvider> <main> <NavBar/> {children} </main> </UserSessionProvider> <Footer/> </body> </html> ) } The code snippet provided showcases a functional component called RootLayout that serves as a layout component in a React application. It wraps the main content with common elements such as an HTML structure, a navigation bar (NavBar), and a footer (Footer). Additionally, it includes the UserSessionProvider component, suggesting that it handles session-related functionality.
The RootLayout component takes a single prop, children, which represents the nested components or content that will be rendered within the layout.
Inside the <body> element, the UserSessionProvider component is used to wrap the main content. This suggests that the UserSessionProvider handles session-related functionality and provides the session object or context to the components nested within it. This can be helpful for managing user authentication and authorization throughout the application.
Within the main content section, the <NavBar/> component is rendered, representing a navigation bar that is likely shared across multiple pages or components. The children prop is rendered, which refers to the nested components or content passed to the RootLayout component.
Finally, after the UserSessionProvider and main content, the <Footer/> component is rendered, representing a footer section that might contain relevant information or links for the application.
Overall, the RootLayout component provides a common layout structure for the application, including session handling with the UserSessionProvider component, a navigation bar, the main content, and a footer. This helps maintain consistency and facilitates the organization and management of shared components and session-related functionality.
Conclusion:
Implementing OAuth-based authentication in Next.js with Google as the authentication provider offers numerous advantages for both developers and users. By following this step-by-step guide, you can seamlessly integrate third-party login capabilities, enhance security, and provide a friction-less user experience. Leverage the power of OAuth and Google to unlock the full potential of your Next.js application!
Remember to regularly update and review your implementation to stay up to date with the latest security best practices and OAuth specifications. Enjoy the benefits of OAuth-based authentication and offer your users a hassle-free login experience, powered by the trusted Google authentication provider.
Comments