Discover the Role of Hibernate, Spring Data JPA & JPA in Database Driven Spring Boot Applications
- CODING Z2M
- Apr 21, 2023
- 6 min read
Updated: Jul 16, 2023
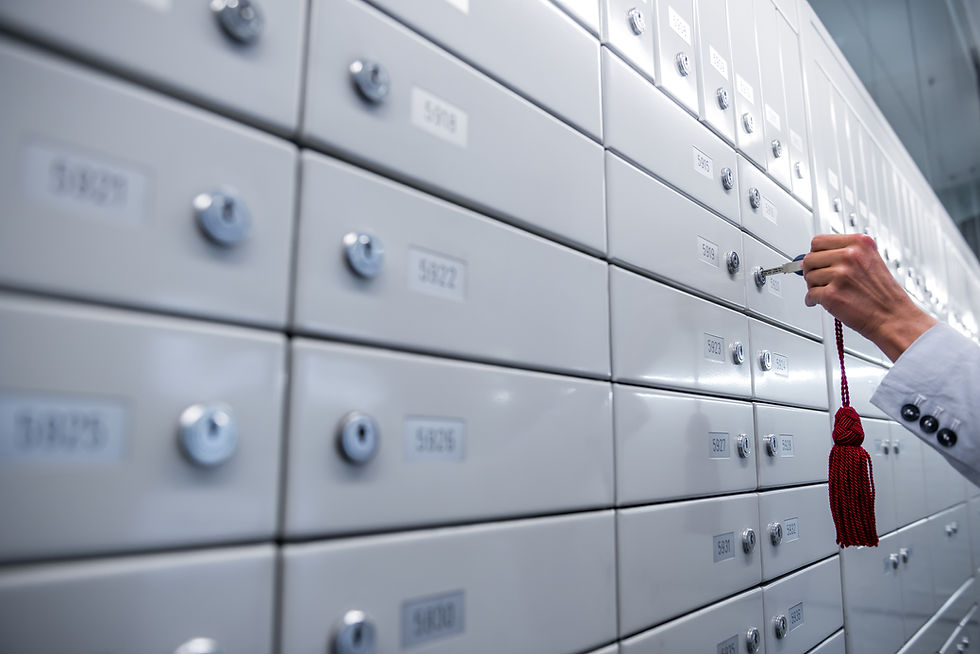
JPA (Java Persistence API) JPA is a specification that defines a set of interfaces and annotations for object-relational mapping (ORM) in Java applications. It provides a standardized way to map Java objects to relational database tables and perform CRUD (Create, Read, Update, Delete) operations on those objects. JPA itself is not an implementation; it's just a specification.
Hibernate Hibernate is one of the most popular implementations of the JPA specification. It is an open-source ORM framework that provides an object-oriented API to interact with the database. Hibernate handles the mapping between Java objects and relational database tables, and it also provides advanced features like lazy loading, caching, and transaction management. In a Spring Boot application, Hibernate is typically used as the JPA implementation.
Spring Data JPA
Spring Data JPA is a part of the Spring Data project and provides a higher-level abstraction on top of JPA. It aims to simplify the development of data access layers by reducing boilerplate code. Spring Data JPA provides a repository abstraction that allows you to define repositories interfaces with standard CRUD operations and custom queries. It also includes built-in support for pagination, sorting, and dynamic query generation based on method names.
How Spring Data JPA can be used in a Spring Boot REST API?
Spring Data JPA is a popular Java library that simplifies the development of data access layer in Spring applications. It provides a powerful set of abstractions that allow developers to work with relational databases in a more efficient and streamlined manner. Here's an example of how Spring Data JPA can be used in a Spring Boot REST API:
Let's say you are developing a REST API for a library management system. You want to allow users to create, read, update, and delete books in the library. To accomplish this, you decide to use Spring Boot to develop the API and Spring Data JPA to interact with the underlying database.
First, you create a Book entity class that represents a book in the library. You annotate the class with the @Entity annotation to indicate that it is a JPA entity and annotate the id field with the @Id annotation to indicate that it is the primary key of the entity. You also define the other fields of the entity, such as title, author, and publication date.
Next, you create a BookRepository interface that extends the JpaRepository interface provided by Spring Data JPA. This interface defines methods for common data access operations, such as finding books by title or author, updating a book's information, and deleting a book.
In your REST API controller class, you inject an instance of the BookRepository interface using the @Autowired annotation. You then define methods that handle HTTP requests, such as GET requests to retrieve a list of all books, POST requests to create a new book, PUT requests to update an existing book, and DELETE requests to delete a book.
When a request is received, the corresponding method in the controller class calls the appropriate method in the BookRepository interface to interact with the database. For example, to retrieve a list of all books, the controller method calls the findAll() method of the BookRepository interface.
Using Spring Data JPA in this way allows you to interact with the database in a more streamlined and efficient manner, without having to write complex SQL queries or handle low-level database operations. It also provides a consistent and standardized way of performing common data access operations, which can save development time and effort.
JPA with Spring - What is Java Persistence API (JPA) ?
JPA is used as data access layer in Java Spring Boot application. We can adopt Hibernate as a persistence provider of the standard Java Persistence API (JPA) and implement data access logic (JPA Repository) in Spring Framework application.
The Java Persistence API (JPA) provides a specification for persisting, reading, managing data from your Java object to relational tables in the database. Hibernate is a ORM (Object/Relational Mapping ) tool/solution for Java environments to map application domain model objects to the relational database tables. Hibernate provides a reference implementation of Java Persistence API (JPA).
JPA abstracts common patterns, define specifications and provides annotations, interfaces, other services, concepts such as PersistenceContext, EntityManager, and the Java Persistence Query Language (JPQL). We can perform various database operations by using JPA’s EntityManager interface and JPQL.
JPA persistence providers, such as Hibernate, EclipseLink, Oracle TopLink,( ORM tools ) are providing implementation.
Spring Framework bootstraps JPA Entity Manager by providing a number of EntityManagerFactoryBean implementations. The Spring Data JPA which is sub module/project of Spring Data project provides advanced support for using JPA in Spring Framework applications and JPA development.
Does Hibernate is used along with Spring Data JPA?
Hibernate is commonly used along with Spring Data JPA. Spring Data JPA is a framework that provides a high-level abstraction on top of JPA (Java Persistence API), which is a standard specification for ORM (Object-Relational Mapping) in Java. Hibernate is one of the most popular implementations of JPA and is commonly used as the underlying ORM provider for Spring Data JPA.
When using Spring Data JPA, you can configure Hibernate as the JPA provider by adding the appropriate dependencies and configuration to your project. Spring Data JPA provides a set of common APIs and abstractions that can be used to interact with the database, while Hibernate takes care of the implementation details of mapping Java objects to database tables and executing SQL queries.
In summary, Hibernate and Spring Data JPA work together to provide a powerful and easy-to-use solution for ORM in Java applications.
How Hibernate is used with Spring Data JPA as JPA provider in data driven spring boot REST API?
Hibernate is commonly used as the underlying JPA provider in Spring Data JPA, which is a framework that provides a high-level abstraction on top of JPA. Here are the general steps to configure Hibernate with Spring Data JPA in a data-driven Spring Boot REST API:
1. Add the necessary dependencies: You need to add the Spring Data JPA and Hibernate dependencies to your project's pom.xml file.
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
</dependencies>
2. Configure the data source: You need to configure the data source that Hibernate will use to connect to the database. You can do this by adding properties to your application.properties or application.yml file. For example:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=dbuser
spring.datasource.password=dbpass
3. Define your entities: You need to define the Java classes that represent your database tables. You can use JPA annotations to specify the mapping between the Java classes and the database tables.
4. Create a repository interface: You need to create a repository interface that extends the JpaRepository interface provided by Spring Data JPA. This interface will provide you with a set of methods for interacting with the database, such as findAll, save, findById, etc. You don't need to provide any implementation for these methods, as Spring Data JPA will generate the necessary queries at runtime based on the method names.
5. Use the repository in your service layer: You can use the repository interface in your service layer to interact with the database. For example, you can inject the repository into your service class using the @Autowired annotation, and then call its methods to perform CRUD operations.
6. Run your application: You can now run your Spring Boot application and test the REST endpoints that you've defined in your controllers. Spring Data JPA will automatically create the necessary database tables based on your entity classes, and Hibernate will handle the low-level details of mapping Java objects to database tables and executing SQL queries.
Does the spring data jpa would create an implementation class for repository interface?
Spring Data JPA generates an implementation class for your repository interface at runtime. This implementation class is created using Spring's dynamic proxy mechanism or bytecode instrumentation, depending on the configuration of your application.
By default, Spring Data JPA generates an implementation class for each repository interface you define. This implementation class provides the actual implementation of the methods declared in your repository interface, such as findAll, findById, save, etc.
The implementation class is generated at runtime based on the information provided in your repository interface. For example, if your repository interface extends JpaRepository, Spring Data JPA will generate an implementation class that provides the default implementation of the methods defined in JpaRepository.
You can also customize the behavior of the generated implementation class by adding custom methods to your repository interface and implementing them in your own code. These custom methods will be available alongside the default methods provided by Spring Data JPA.
In summary, Spring Data JPA generates an implementation class for your repository interface at runtime, which provides the actual implementation of the methods declared in your repository interface. This implementation class is customizable, and you can add custom methods to your repository interface and implement them in your own code.
Comments