Component Rendering Revolution: Next.js CSR vs SSR at the Component Level
- CODING Z2M
- Jun 14, 2023
- 5 min read
Updated: Jun 21, 2023
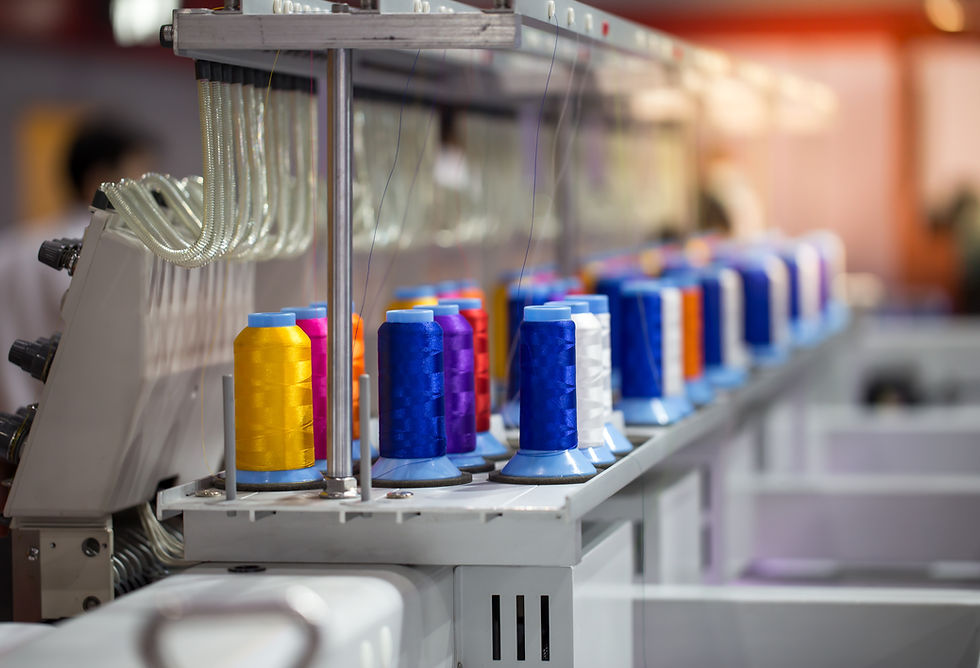
Next.js CSR vs Next.js SSR: Next.js, a popular React framework, offers developers the ability to leverage both client-side rendering (CSR) and server-side rendering (SSR) at the component level. This powerful feature allows for fine-grained control over rendering methods, optimizing performance, and delivering dynamic user experiences. In this article, we will explore the concept of component-level rendering in Next.js, its benefits, and how to effectively utilize it in your projects.
Understanding Component-Level Rendering: Component-level rendering refers to the capability of Next.js to choose the appropriate rendering method on a per-component basis. This means that certain components within a Next.js application can be rendered on the server while others can be rendered on the client-side, providing flexibility and optimization opportunities.
Leveraging Client-Side Rendering (CSR) at the Component Level: By opting for client-side rendering at the component level, developers can achieve highly interactive and dynamic experiences. Components rendered on the client-side can fetch data from APIs, respond to user interactions in real-time, and update without full page reloads. This approach is suitable for components that require frequent updates, real-time data, or user-driven interactions.
Harnessing Server-Side Rendering (SSR) at the Component Level: Server-side rendering at the component level is valuable when search engine optimization (SEO) and initial load performance are crucial. By rendering specific components on the server, Next.js can generate pre-rendered HTML content that is readily available to search engine crawlers and improves the overall performance of the application. Components benefiting from SSR include static content, landing pages, and frequently accessed information.
Choosing the Right Rendering Strategy for Components: To determine the appropriate rendering strategy for each component, consider the specific requirements and characteristics of the component. Ask yourself the following questions:
Does the component require real-time data or frequent updates? If yes, consider CSR.
Is search engine visibility important for the component? If so, prioritize SSR.
Are there performance constraints or content that rarely changes? In such cases, SSR might be the preferred option.
Example of Client-Side Rendering (CSR) at the component level in Next.js is a social media feed component:
The social media feed component contains posts from various users with associated likes, comments, and interactions. Instead of pre-rendering the entire feed on the server-side, Next.js can fetch the initial data (e.g., the latest posts) from an API endpoint and render the component on the client-side.
With CSR, the feed component is initially displayed with placeholder content while the client-side JavaScript code retrieves the actual post data asynchronously. Once the data is fetched, the component dynamically updates with the real-time content, including post text, user avatars, likes, and comments. Users can interact with the posts, such as adding comments or liking them, without triggering a full page reload.
import { useEffect, useState } from 'react';
const SocialMediaFeed = () => {
const [posts, setPosts] = useState([]);
useEffect(() => {
const fetchPosts = async () => {
const response = await fetch('https://api.example.com/posts');
const data = await response.json();
setPosts(data);
};
fetchPosts();
}, []);
const handleLike = (postId) => {
// Simulating a client-side like action
setPosts((prevPosts) =>
prevPosts.map((post) =>
post.id === postId ? { ...post, likes: post.likes + 1 } : post
)
);
};
return (
<div>
<h1>Social Media Feed</h1>
{posts.length > 0 ? (
<ul>
{posts.map((post) => (
<li key={post.id}>
<h3>{post.title}</h3>
<p>{post.body}</p>
<div>
Likes: {post.likes}
<button onClick={() => handleLike(post.id)}>Like</button>
</div>
<div>Comments: {post.comments}</div>
</li>
))}
</ul>
) : (
<p>Loading posts...</p>
)}
</div>
);
};
export default SocialMediaFeed;
In the above code, we create a SocialMediaFeed component responsible for rendering a social media feed. Inside the component, we utilize React's useEffect and useState hooks.
The useEffect hook is used to perform an asynchronous API call to fetch the posts data from the server. In this example, we simulate the API call using the fetch function. Once the data is retrieved, it is stored in the component's state using the setPosts function from the useState hook.
The component's JSX code renders a title heading, followed by a conditional rendering based on the state of the posts array. If the posts array has data, it iterates through each post using the map function and renders the post's title, body, likes, and comments. If the posts array is empty (initially), it displays a loading message.
By utilizing CSR at the component level, the social media feed component initially renders with a loading message and asynchronously fetches the actual post data from the API. Once the data is retrieved, the component updates to display the posts dynamically.
This approach allows for a faster initial page load, as only the necessary HTML structure is initially sent from the server. The client-side JavaScript then handles the fetching and rendering of the actual post content, resulting in a more interactive and responsive user experience.
This example has a client-side action of liking a post. When the user clicks the "Like" button, the handleLike function is triggered.
The handleLike function takes the postId as an argument and updates the likes count for the respective post. In this simulated scenario, we update the likes count by incrementing it by one. We achieve this by using the setPosts function with the map method to update the specific post in the posts state array.
By adding this client-side interactivity, the user can click the "Like" button on a post, and the component updates immediately to reflect the increased number of likes without triggering a full page reload. This showcases the benefits of Client-Side Rendering (CSR) at the component level in Next.js, enabling dynamic and real-time user interactions.\
Real-word Example for server-Side Rendering (SSR) at the component level in Next.js:
One real-world example of server-side rendering (SSR) at the component level in Next.js is when you have a dynamic webpage that needs to fetch data from an external API and render it on the server before sending the fully rendered page to the client.
For instance, let's say you have a Next.js application that displays a list of blog posts. Each blog post is fetched from a backend API. In this case, you can use SSR at the component level to fetch the blog post data on the server and render it before sending the HTML response to the client.
Here's an example code snippet showcasing SSR at the component level in Next.js:
import React from 'react';
import axios from 'axios';
const BlogPost = ({ post }) => {
return (
<div>
<h2>{post.title}</h2>
<p>{post.content}</p>
</div>
);
};
export async function getServerSideProps() {
const response = await axios.get('https://api.example.com/posts/123');
const post = response.data;
return {
props: {
post,
},
};
}
export default BlogPost;
In this example, the getServerSideProps function is used to fetch the specific blog post data from the API on the server side. The fetched data is then passed as props to the BlogPost component, which renders the blog post title and content. When a user requests this page, Next.js will execute the getServerSideProps function on the server, fetch the data, and pass it to the component for rendering. The server then sends the fully rendered HTML to the client.
This way, the client receives a pre-rendered HTML page with the blog post data already populated, which improves performance and SEO while providing a dynamic and interactive experience for the user.
Comments