Inversion of Control (IoC) and Dependency Injection (DI) & AOP in Spring | Spring Core & Spring MVC
- CODING Z2M
- Apr 19, 2023
- 5 min read
Updated: Jul 15, 2023
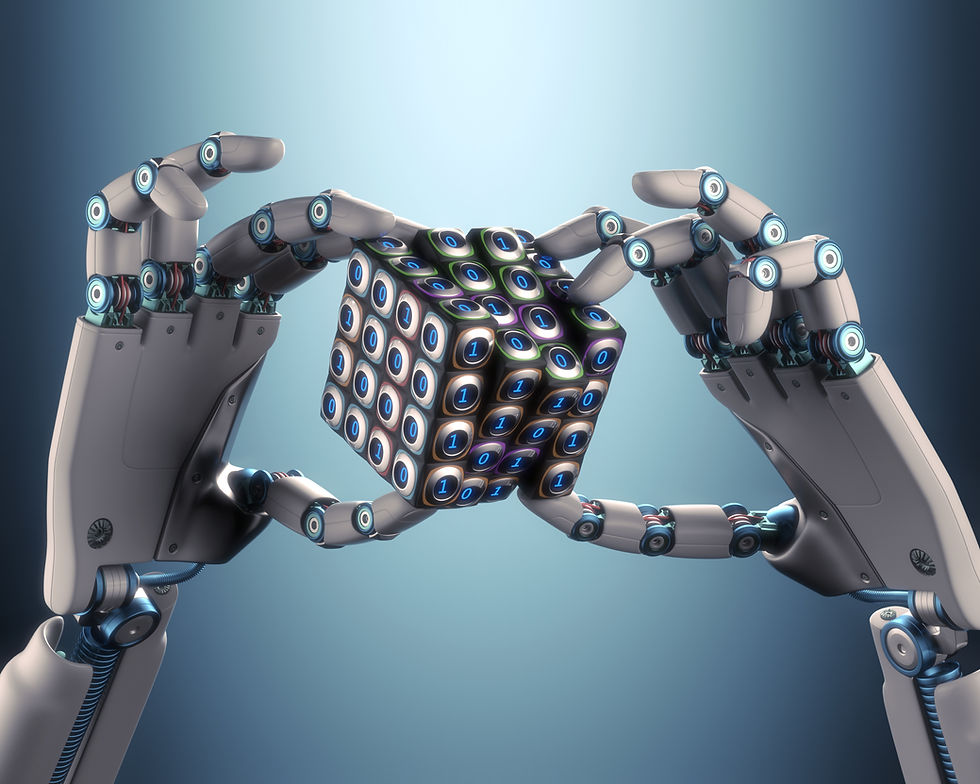
Inversion of Control (IoC) and Dependency Injection (DI) are two key concepts in the Spring Framework that help to reduce the complexity of Java-based applications. In this blog post, we will explore what IoC and DI are, how they work, and why they are important.
What is Inversion of Control (IoC)?
Inversion of Control (IoC) is a design pattern that allows the control flow of an application to be inverted. In traditional Java programming, the programmer would be responsible for creating and managing the objects in an application. However, with IoC, the responsibility for managing objects is shifted to a container, which is responsible for creating and managing the objects.
The container in IoC is responsible for creating and managing objects, and it injects dependencies into the objects it creates. This allows objects to be created and managed independently of the rest of the application, which makes them easier to test and maintain.
What is Dependency Injection (DI)?
Dependency Injection (DI) is a design pattern that allows objects to be created with their dependencies injected. With DI, the objects themselves don't create their dependencies; instead, they are created and managed by a container.
In DI, the container injects dependencies into objects, which makes it easier to manage the lifecycle of the objects. DI also helps to reduce the coupling between objects, which makes it easier to test and maintain the application.
How do IoC and DI work in Spring?
In Spring, IoC and DI are implemented using a container called the ApplicationContext. The ApplicationContext is responsible for creating and managing objects, and it injects dependencies into the objects it creates.
To use IoC and DI in Spring, you need to define the beans that you want to use in your application. A bean is simply an object that is managed by the ApplicationContext. When you define a bean in Spring, you can specify its dependencies using constructor injection or setter injection. With constructor injection, the dependencies are injected when the object is created, while with setter injection, the dependencies are injected after the object is created.
Why are IoC and DI important?
IoC and DI are important because they help to reduce the complexity of Java-based applications. By shifting the responsibility for managing objects to a container, you can reduce the amount of boilerplate code that you need to write.
IoC and DI also make it easier to test and maintain your applications. With IoC, objects are created and managed independently of the rest of the application, which makes them easier to test. With DI, objects are created with their dependencies injected, which makes it easier to test and maintain the application.
Conclusion
In conclusion, Inversion of Control (IoC) and Dependency Injection (DI) are two key concepts in the Spring Framework that help to reduce the complexity of Java-based applications. By shifting the responsibility for managing objects to a container, you can reduce the amount of boilerplate code that you need to write, and make your applications easier to test and maintain. If you're new to Spring, learning IoC and DI is a great place to start, and will help you to build better, more maintainable applications.
Spring Core & Spring MVC
Spring is a popular Java framework that offers several modules to develop robust, scalable, and high-performance Java applications. Spring Core and Spring MVC are two important modules of the Spring framework. In this blog post, we'll explore both of these modules in detail and see how they can be used to develop modern web applications.
Spring Core:
Spring Core is the foundation of the Spring framework. It provides the Inversion of Control (IoC) and Dependency Injection (DI) features that are at the heart of the Spring framework. IoC allows the objects to be loosely coupled, which makes them more reusable, maintainable, and testable. DI provides a way to inject dependencies into objects at runtime, which helps to reduce the tight coupling between objects.
Spring Core also provides several other features, such as AOP (Aspect-Oriented Programming), event handling, and resource management. AOP allows for modularization of cross-cutting concerns, such as logging, security, and transactions. Event handling provides a way to decouple components by allowing them to communicate through events. Resource management allows for easy integration with different types of resources, such as databases, messaging systems, and web services.
Spring MVC:
Spring MVC is a web framework that is built on top of the Spring Core module. It provides a Model-View-Controller (MVC) architecture that is used to develop web applications. The MVC architecture separates the application into three parts: the model (the data), the view (the user interface), and the controller (the business logic).
Spring MVC provides several features, such as request mapping, data binding, validation, and exception handling. Request mapping allows for mapping URLs to controller methods, which provides a way to handle HTTP requests. Data binding allows for converting HTTP request data into Java objects, which makes it easier to work with form data. Validation provides a way to validate user input, which helps to improve the quality of the data. Exception handling provides a way to handle exceptions in a consistent manner, which makes the application more robust.
Integration of Spring Core and Spring MVC:
Spring Core and Spring MVC can be integrated to develop modern web applications. Spring MVC uses the IoC and DI features provided by Spring Core to manage the application's components. The controllers, which are responsible for handling HTTP requests, are typically managed by Spring. This allows for loose coupling between the controllers and the rest of the application.
Spring Core and Spring MVC also provide several other features that make it easier to develop web applications. For example, Spring Security, which is built on top of Spring Core, provides a way to implement authentication and authorization in web applications. Spring Data, which is also built on top of Spring Core, provides a way to work with databases using a consistent and easy-to-use API.
Conclusion:
Spring Core and Spring MVC are two important modules of the Spring framework. Spring Core provides the foundation of the Spring framework, while Spring MVC provides a web framework for developing web applications. These two modules can be integrated to develop modern web applications that are scalable, maintainable, and high-performance. Spring also provides several other modules that can be used in conjunction with Spring Core and Spring MVC to develop more complex applications. Overall, Spring is a powerful framework that can help developers to be more productive and write better code. What is AOP (Aspect Oriented Programming)?
Aspect Oriented Programming (AOP) is a programming paradigm that allows developers to modularize cross-cutting concerns, which are concerns that span multiple parts of an application and are not related to the core business logic. In other words, AOP enables developers to separate out functionalities that cut across the different modules or components of an application and apply them consistently and transparently.
Traditional object-oriented programming (OOP) approaches typically result in tangled and complex code, with cross-cutting concerns scattered throughout the application. AOP provides a way to address this complexity by separating these concerns into reusable, modular units called aspects.
Aspects are essentially a set of behaviors that can be applied to multiple parts of an application. For example, logging is a cross-cutting concern that could be applied to various parts of an application, such as authentication, authorization, and validation. By separating out logging as an aspect, developers can ensure that it is consistently and transparently applied across the application, without having to write duplicate code or modify the core business logic.
AOP achieves this separation of concerns by introducing a new set of concepts and mechanisms, such as join points, pointcuts, advice, and weaving. Join points are specific points in an application where an aspect can be applied, such as method calls, field access, or exception handling. Pointcuts are expressions that define a set of join points that are to be intercepted by an aspect. Advice is the code that is executed at a join point. Weaving is the process of combining an aspect with the core application code to create a woven application.
Comments