Using JWT in Node.js Express REST API
- CODING Z2M
- Apr 1, 2023
- 3 min read
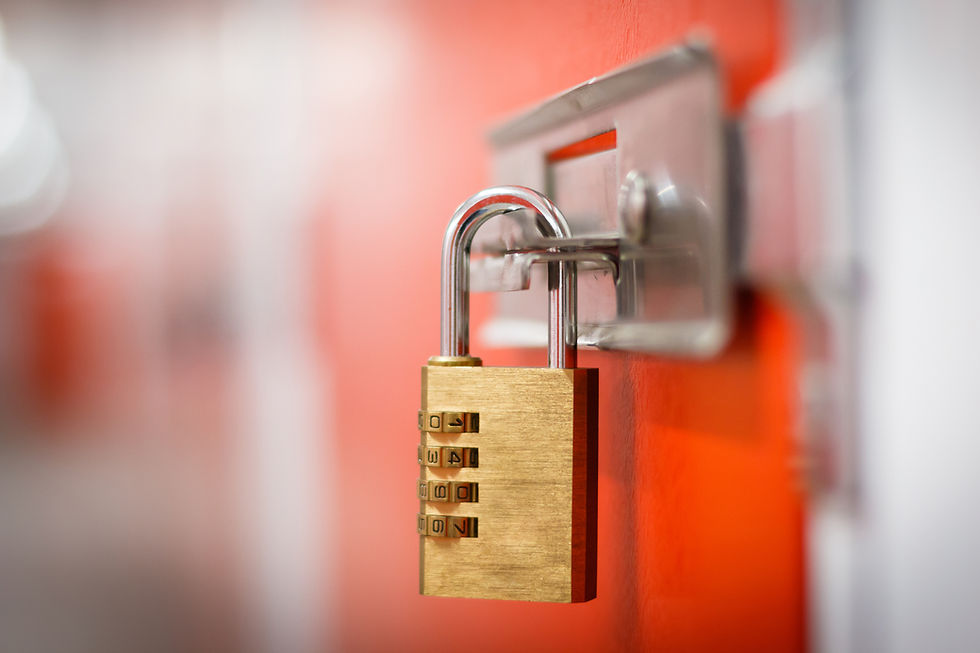
JWT (JSON Web Token) is a popular method for securely transmitting information between parties as a JSON object. It is often used as a way to authenticate and authorize users in web applications and APIs. A JWT consists of three parts: a header, a payload, and a signature. The header contains information about the type of token and the algorithm used to sign it. The payload contains the claims, or assertions, about the user being authenticated. The signature is used to verify the integrity of the token.
Example:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
This JWT consists of three parts separated by dots:
The first part is the header, which contains information about the type of token and the algorithm used to sign it. In this case, the algorithm used is HMAC-SHA256.
The second part is the payload, which contains the claims or assertions about the user being authenticated. In this example, the payload contains three claims: the user's ID (sub), their name (name), and the timestamp of when the token was issued (iat).
The third part is the signature, which is used to verify the integrity of the token. The signature is generated by hashing the header and payload with a secret key that is known only to the server.
This JWT can be decoded to reveal the claims in the payload, which can then be used to authenticate and authorize the user in subsequent requests.
In Node.js and Express, using JWTs is relatively easy with the help of the jsonwebtoken package. Here are the steps you can follow to use JWT in your Node.js Express REST API:
Install the jsonwebtoken package by running the following command in your terminal: npm install jsonwebtoken
Import the jsonwebtoken package into your Node.js file: const jwt = require('jsonwebtoken');
Create a secret key for signing and verifying the JWT: const secretKey = 'your-secret-key';
Create a function that generates a JWT. This function should take in a payload (the information you want to transmit) and sign it using the secret key. You can also set an expiration time for the token: function generateToken(payload) { const token = jwt.sign(payload, secretKey, { expiresIn: '1h' }); return token; }
Create a middleware function that verifies the JWT. This function should be added to any route that requires authentication. It should take in the token from the request headers and verify it using the secret key: function authenticateToken(req, res, next) { const authHeader = req.headers['authorization'];
const token = authHeader && authHeader.split(' ')[1];
if (!token) {
return res.sendStatus(401);
}
jwt.verify(token, secretKey, (err, user) => {
if (err) {
return res.sendStatus(403);
}
req.user = user;
next();
});
}
6. Add the authentication middleware to any route that requires authentication:
app.get('/protected-route', authenticateToken, (req, res) => {
// This route is protected and requires authentication
res.send('This route is protected');
});
7. Use the generateToken function to generate a token for a user and send it back as a response:
app.post('/login', (req, res) => {
const { username, password } = req.body;
// Check if the username and password are valid
// ...
const payload = { username: username };
const token = generateToken(payload);
res.json({ token: token });
});
In the above code, authenticateToken function uses authHeader.split(' ') to split the authorization header into an array with two elements: the authentication type (e.g. "Bearer") and the token itself.
The reason why we split the header string using a space character (' ') is that the Authorization header typically has the format Authorization: <type> <credentials>, where <type> is the authentication scheme (e.g. "Bearer" or "Basic") and <credentials> is the actual token or credentials.
For example, if the Authorization header value is "Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c", we want to extract the token part after the "Bearer " substring.
By splitting the header string using a space character (' '), we can separate the "Bearer" substring from the actual token and get an array like ["Bearer", "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c"]. Then, we can access the token by getting the second element of the array using authHeader.split(' ')[1].
Note that this assumes that the Authorization header always has the "Bearer" authentication type. If your API uses a different authentication scheme, you may need to modify the authenticateToken function to handle it correctly.
留言