Test-Driven Development (TDD) in Node.js Express App
- CODING Z2M
- May 2, 2023
- 4 min read
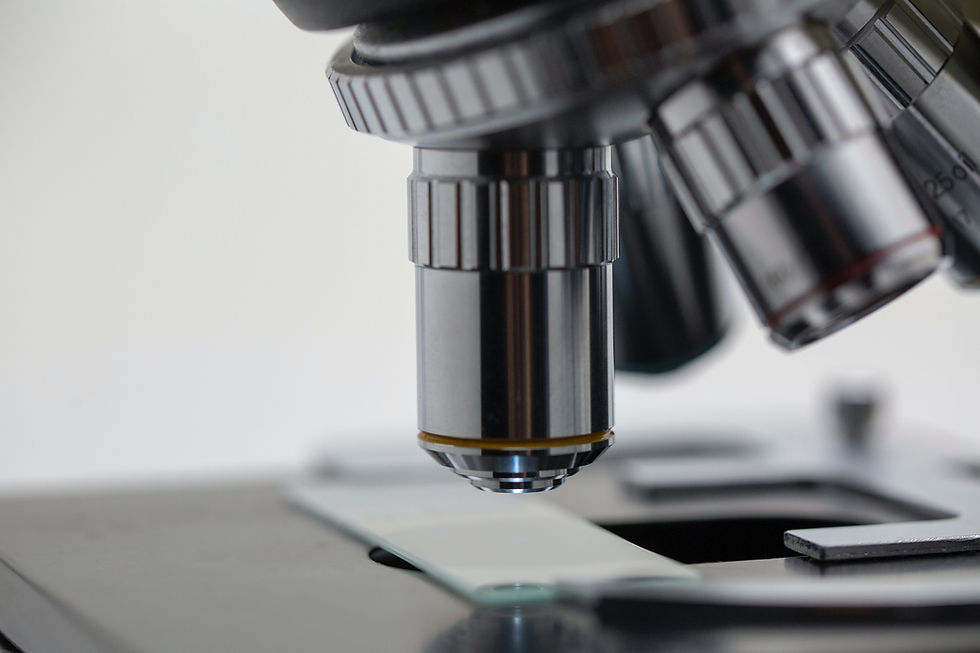
Test-driven development (TDD) is a software development approach that involves writing tests before writing the actual code. In TDD, tests are written to specify the expected behavior of a software system, and the code is then written to make the tests pass. TDD helps to ensure that the code is of high quality and that it meets the specified requirements. Note: Run the test cases, using the file names, for example > npx jest validatePassword.test.js, > npx jest array.test.js
Node.js is a popular platform for building server-side applications, and Express is a popular framework for building web applications with Node.js. When building a Node.js Express app using TDD, the following steps can be followed:
Write a failing test: The first step in TDD is to write a test that fails. This test should specify the desired behavior of the code. For example, if you are building an endpoint that returns a list of users, you can write a test that checks whether the endpoint returns a list of users.
Run the test: Once the test is written, you can run it to ensure that it fails. This step helps to ensure that the test is working as expected.
Write the code: After writing the failing test, the next step is to write the code that implements the desired behavior. For example, you can write code that retrieves a list of users from a database and returns them as a JSON object.
Run the test again: After writing the code, you should run the test again to ensure that it passes. If the test fails, you should go back and modify the code until the test passes.
Refactor the code: Once the test passes, you can refactor the code to improve its readability and maintainability. This step involves modifying the code without changing its behavior.
Repeat: You can repeat the above steps for other features and functionalities of your Node.js Express app.
In summary, TDD is a useful approach for building high-quality Node.js Express apps. By writing tests first, you can ensure that the code meets the specified requirements and is of high quality.
Here's an example of how TDD can be applied to test a function that adds two numbers in JavaScript: Write a failing test: The first step is to write a test that specifies the expected behavior of the function. For example, you can write a test that checks whether the function adds two numbers correctly: const { add } = require('./addition');
test('adds 2 + 3 to equal 5', () => {
expect(add(2, 3)).toBe(5);
});
Here, we're using Jest as the testing framework and assuming that the add function is defined in a separate module called addition.js.
Run the test: When you run the test, it should fail because the add function hasn't been implemented yet.
Write the code: Now that we have a failing test, we can write the code that implements the add function:
function add(a, b) {
return a + b;
}
module.exports = { add };
Run the test again: Once the code is implemented, we can run the test again to ensure that it passes:
PASS ./addition.test.js
✓ adds 2 + 3 to equal 5 (2ms)
Test Suites: 1 passed, 1 total
Tests: 1 passed, 1 total
Refactor the code: Finally, we can refactor the code if necessary. For example, we can rename the add function to a more descriptive name like sum.
By following this TDD approach, we have ensured that the add function works correctly and meets the specified requirements. This simple example demonstrates how TDD can be applied to any function or feature in a software project to ensure that it works as intended.
Here's another example of Test Driven Development (TDD) in Node.js using the Jest testing framework. we can add test cases to create a functions that calculates the average of an array of numbers, to check an empty array and an array with only one element:
//arrayFunctions.js
function average(numbers) { if (numbers.length === 0) { return 0; } const sum = numbers.reduce((acc, curr) => acc + curr, 0); return sum / numbers.length; } module.exports = average; function sumArray(arr) { if (arr.length === 0) return 0; if(arr.length >= 0) return sum = arr.reduce((acc, curr) => acc + curr, 0); } function getFirstElement(arr) { if (arr.length >= 1) { return arr[0]; } } module.exports= {average, sumArray, getFirstElement}
const { average, getFirstElement, sumArray } = require('./arrayFunctions'); describe('Average', () => { test('should calculate the average of an array of numbers', () => { const numbers = [2, 4, 6, 8]; expect(average(numbers)).toBe(5); }); test('should return 0 for an empty array', () => { const numbers = []; expect(average(numbers)).toBe(0); }); test('should return the same number for an array with only one element', () => { const numbers = [5]; expect(average(numbers)).toBe(5); }); }); describe('getFirstElement', () => { test('should return the first element of an array', () => { const numbers = [1, 2, 3]; expect(getFirstElement(numbers)).toBe(1); }); test('should return undefined for an empty array', () => { const numbers = []; expect(getFirstElement(numbers)).toBeUndefined(); }); }); describe('sumArray', () => { test('should return the sum of an array of numbers', () => { const numbers = [1, 2, 3]; expect(sumArray(numbers)).toBe(6); }); test('should return 0 for an empty array', () => { const numbers = []; expect(sumArray(numbers)).toBe(0); }); });
Comments