Benefits Defining Classes in a Node.js Express REST API
- CODING Z2M
- Apr 1, 2023
- 3 min read
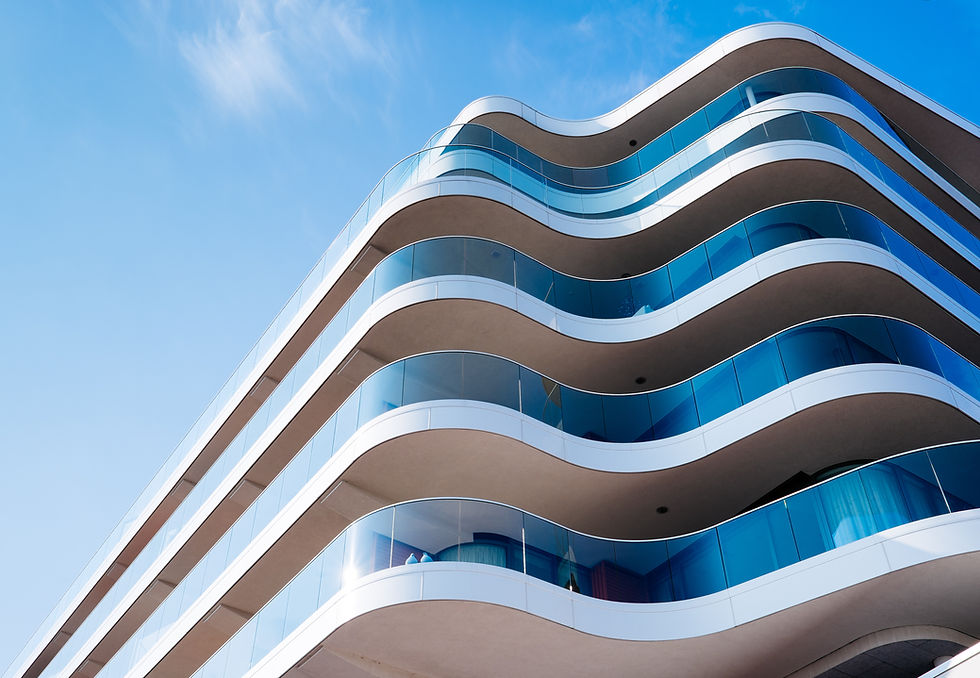
Defining classes and objects in a Node.js Express REST API can provide several benefits, including:
Modularity: Classes and objects provide a modular structure to your codebase by encapsulating related functionality together. This makes your code easier to understand, maintain, and test.
Reusability: Once you define a class or object, you can reuse it throughout your codebase. This can save you time and effort by reducing code duplication.
Abstraction: Classes and objects can abstract away complex logic and expose a simple interface for other parts of your code to interact with. This can make your codebase easier to work with and reduce the risk of bugs.
Encapsulation: Classes and objects can encapsulate data and behavior, protecting it from external interference. This can make your codebase more secure and prevent unintended side effects.
Polymorphism: Classes and objects can be used to implement polymorphic behavior, allowing you to write generic code that can work with objects of different types. This can make your code more flexible and adaptable to changing requirements.
In a Node.js Express REST API, you can define classes and objects to represent resources, middleware, controllers, models, and more. For example, you can define a User class to represent users in your system, a Product class to represent products in your inventory, or a Logger class to handle logging throughout your application.
Here's an example of how we can implement a model-controller-service architecture in a Node.js REST API using Mongoose:
Define the Mongoose schema for the model (in this example, we'll use a book model): const mongoose = require('mongoose'); const bookSchema = new mongoose.Schema ({ title: { type: String, required: true }, author: { type: String, required: true }, pages: { type: Number, required: true }, publishedDate: { type: Date, required: true }, });
const Book = mongoose.model('Book', bookSchema);
module.exports = Book;
2. Define the controller for the model: const Book = require('../models/book');
class BookController {
static async getAll(req, res, next) {
try {
const books = await Book.find();
res.status(200).json(books);
} catch (error) {
next(error);
}
}
static async getById(req, res, next) {
try {
const book = await Book.findById(req.params.id);
if (!book) throw new Error('Book not found');
res.status(200).json(book);
} catch (error) {
next(error);
}
}
static async create(req, res, next) {
try {
const newBook = new Book(req.body);
await newBook.save();
res.status(201).json(newBook);
} catch (error) {
next(error);
}
}
static async update(req, res, next) {
try {
const updatedBook = await Book.findByIdAndUpdate(req.params.id, req.body, { new: true });
if (!updatedBook) throw new Error('Book not found');
res.status(200).json(updatedBook);
} catch (error) {
next(error);
}
}
static async delete(req, res, next) {
try {
const deletedBook = await Book.findByIdAndDelete(req.params.id);
if (!deletedBook) throw new Error('Book not found');
res.status(200).json(deletedBook);
} catch (error) {
next(error);
}
}
}
module.exports = BookController;
In this example, the controller defines static methods for handling HTTP requests for the book model. Each method takes a request object (req), a response object (res), and a next function as arguments. The next function is used to pass errors to the next middleware function in the Express middleware stack. 3. Define the service for the model:
const Book = require('../models/book');
class BookService {
static async getAll() {
return await Book.find();
}
static async getById(id) {
return await Book.findById(id);
}
static async create(book) {
const newBook = new Book(book);
await newBook.save();
return newBook;
}
static async update(id, book) {
return await Book.findByIdAndUpdate(id, book, { new: true });
}
static async delete(id) {
return await Book.findByIdAndDelete(id);
}
}
module.exports = BookService; In this example, the service defines static methods for CRUD operations on the book model. Each method returns a Promise that resolves to the result of the operation. 4. Use the controller and service in the Express app:
const express = require('express');
const bodyParser = require('body-parser');
const BookController = require('./controllers/book-controller');
const BookService = require('./services/book-service');
const app = express();
app.use(bodyParser.json());
// Get all books
Comments