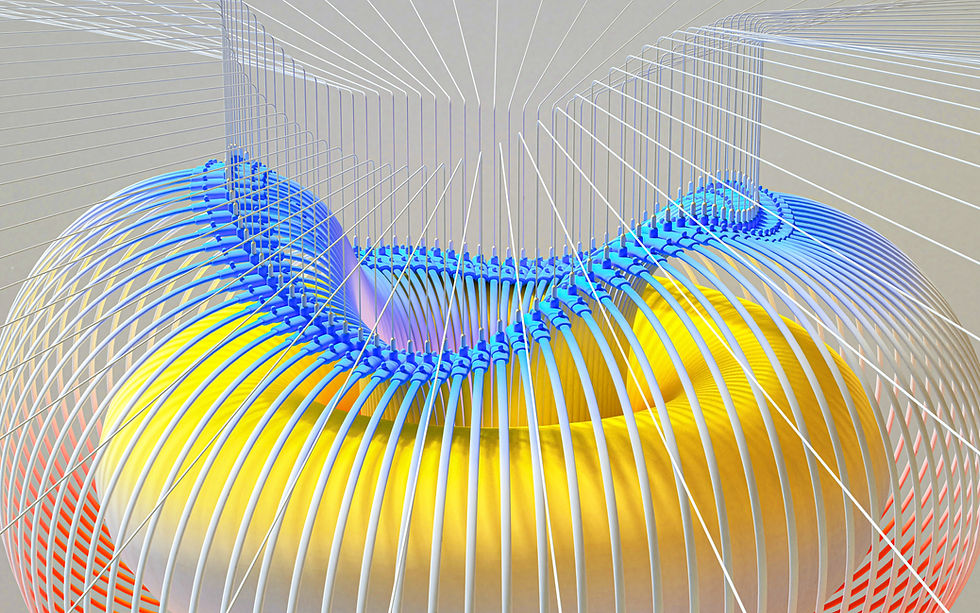
Understanding JSX in React
As a developer working with React, you may have heard of JSX, a syntax extension that allows developers to write HTML-like code in JavaScript. In this blog post, we'll explore what JSX is, why it's used in React, and provide a real-world example to help you understand how to use it in your own projects.
JSX is a syntax extension for JavaScript that allows developers to write HTML-like code in their JavaScript files. It was created by Facebook to help developers build user interfaces in React applications. With JSX, you can define the structure and content of user interfaces using a syntax that closely resembles HTML, but with the full power of JavaScript.
Why use JSX in React
React uses JSX as a way to define the structure and content of user interfaces in a concise and intuitive way. Without JSX, developers would need to use JavaScript functions to create and manipulate HTML elements, which can be more verbose and less intuitive. JSX makes it easier for developers to create and maintain React applications by providing a familiar syntax that can be quickly understood and modified.
import React from 'react';
function App() {
return (
<div className="App">
<h1>Hello, world!</h1>
<p>This is a React app using JSX.</p>
</div>
);
}
export default App;
In this example, we're defining a simple functional component called App. Instead of returning a string or a plain JavaScript object, we're returning JSX code.
The div element with the className attribute is a JSX representation of an HTML div element with a class name of App. Inside the div, we have an h1 element and a p element, each with some text content.
Note that we're also using curly braces {} to insert JavaScript expressions into our JSX code. In this case, we're using curly braces to insert the string "Hello, world!" as the content of the h1 element and the string "This is a React app using JSX." as the content of the p element.
When this component is rendered in the browser, the JSX code will be transformed into regular JavaScript code that creates and manipulates HTML elements, just like any other JavaScript code. JSX can also be used to render components dynamically based on data. Here's an example:
import React from 'react';
function App() {
const users = [
{ name: 'John', age: 25 },
{ name: 'Jane', age: 30 },
{ name: 'Bob', age: 40 }
];
return (
<div className="App">
<h1>User List</h1>
<ul>
{users.map(user => (
<li key={user.name}>
<p>Name: {user.name}</p>
<p>Age: {user.age}</p>
</li>
))}
</ul>
</div>
);
}
export default App;
In this example, we're using JSX to dynamically render a list of users. We have an array of users, each with a name and an age property. Inside the ul element, we're using the map method to loop through the users array and render a li element for each user.
Note that we're using the key attribute to provide a unique identifier for each li element. This is important for performance optimization and is required by React when rendering dynamic lists.
Inside each li element, we're using curly braces {} to insert JavaScript expressions that dynamically render the name and age properties of each user.
JSX provides a powerful and intuitive way to write dynamic and reusable UI components in React. By mastering JSX, you can create complex and sophisticated web applications with ease.
Another powerful feature of JSX is the ability to pass props to components. Here's an example:
import React from 'react';
function Greeting(props) {
return (
<div>
<h1>Hello, {props.name}!</h1>
<p>Your age is {props.age}.</p>
</div>
);
}
function App() {
return (
<div className="App">
<Greeting name="John" age="25" />
<Greeting name="Jane" age="30" />
<Greeting name="Bob" age="40" />
</div>
);
}
export default App;
In this example, we have a simple Greeting component that takes two props: name and age. Inside the component, we're using curly braces to dynamically render the values of the name and age props.
In the App component, we're using the Greeting component three times, each with different values for the name and age props. This demonstrates how easy it is to reuse components with different props to create dynamic and flexible UIs.
JSX is an essential part of React and is a powerful tool for creating complex and dynamic web applications. By mastering JSX, you can unlock the full potential of React and build amazing user interfaces with ease.
JSX also supports conditional rendering, which allows you to render different content based on certain conditions. Here's an example:
import React from 'react';
function App() {
const isLoggedIn = true;
return (
<div className="App">
{isLoggedIn ? (
<h1>Welcome back, User!</h1>
) : (
<h1>Please log in to continue.</h1>
)}
</div>
);
}
export default App;
In this example, we have a boolean variable called isLoggedIn that indicates whether the user is logged in or not. Inside the div element, we're using a ternary operator to conditionally render different content based on the value of isLoggedIn. If isLoggedIn is true, we render a h1 element that says "Welcome back, User!" Otherwise, we render a h1 element that says "Please log in to continue."
This is just a simple example of conditional rendering, but it can be used to create more complex UIs that adapt to the user's state and behavior.
In summary, JSX is a powerful tool that allows you to write HTML-like code in your JavaScript code, making it easy to create dynamic and reusable UI components in React. By mastering JSX, you can create sophisticated web applications with ease and unlock the full potential of React.
Another feature of JSX is the ability to define and use custom components. Custom components are essentially functions that return JSX, and they can be used just like built-in components in your React applications. Here's an example:
import React from 'react';
function Avatar(props) {
return (
<div className="avatar">
<img src={props.image} alt={props.alt} />
<p>{props.name}</p>
</div>
);
}
function App() {
return (
<div className="App">
<h1>User List</h1>
<Avatar name="John" image="https://example.com/john.jpg" alt="John's profile picture" />
<Avatar name="Jane" image="https://example.com/jane.jpg" alt="Jane's profile picture" />
<Avatar name="Bob" image="https://example.com/bob.jpg" alt="Bob's profile picture" />
</div>
);
}
export default App;
In this example, we're defining a custom component called Avatar that takes three props: name, image, and alt. Inside the component, we're rendering an img element with the src and alt props, as well as a p element with the name prop.
In the App component, we're using the Avatar component three times, each with different values for the name, image, and alt props. This demonstrates how easy it is to create reusable components with JSX and use them in your React applications.
Custom components are a powerful feature of JSX that allow you to create modular and reusable UI components in your React applications. By mastering custom components, you can create more complex and flexible UIs with ease.
Comments