Harnessing the Power of React Context API for Effortless State Management
- CODING Z2M
- Aug 28, 2023
- 5 min read
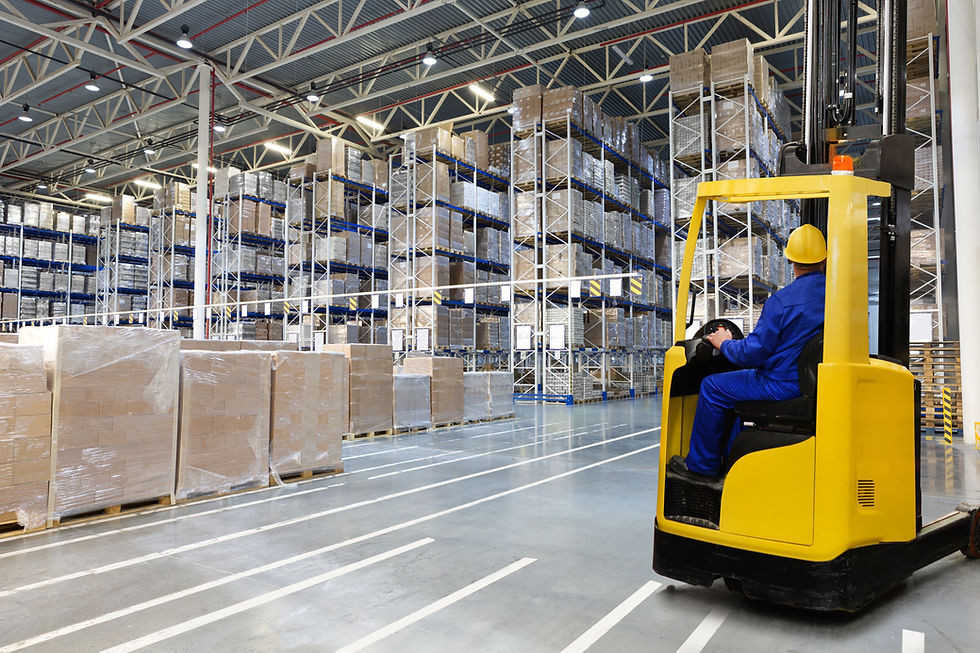
In the fast-paced world of web development, React has solidified its position as one of the most popular JavaScript libraries for building dynamic and interactive user interfaces. With React's component-based architecture, creating reusable and maintainable UI elements is a breeze. However, as your application grows, managing state efficiently across components can become a challenge. This is where the React Context API steps in, offering an elegant solution to streamline state management and make your code more maintainable and readable.
Understanding the Need for State Management
Before diving into the React Context API, let's understand why state management is crucial. In a React application, state represents the data that can change over time and affect the behavior and appearance of components. As your app scales, passing state down through the component tree using props can become cumbersome and error-prone. This is especially true when you need to share state between deeply nested components or when different parts of your application need access to the same data.
Enter React Context API
The React Context API is a built-in feature that simplifies state management by providing a way to share data and functions between components without having to pass props manually. It essentially creates a global data store that any component within your app can tap into.
Here's how it works:
Create a Context: First, you define a context using the createContext function. This context acts as a container for your shared data.
Provide Data: Wrap your application (or a part of it) with a <Context.Provider> component. This provider supplies the data that you want to share to all components within its subtree.
Consume Data: Any component within the provider's subtree can access this shared data by using the useContext hook or the Context.Consumer component. This eliminates the need to pass props down through multiple levels of components.
Benefits of Using React Context API
Simplifies Prop Drilling: Say goodbye to the hassle of passing props down the component tree just to share data. With React Context, your data is available where you need it.
Enhances Code Maintainability: Context encourages cleaner and more maintainable code. Since data is centralized, it's easier to make changes or additions without touching multiple components.
Global Data Management: Ideal for managing global application state, such as user authentication, theme preferences, or language settings.
Reduces Component Coupling: Components become more independent as they don't need to know where their data is coming from. This reduces coupling and makes your codebase more flexible and easier to test.
Best Practices
While React Context API is a powerful tool, it's essential to follow some best practices:
Avoid Overusing Context: Reserve Context for data that genuinely needs to be shared across many components. Overusing it can lead to unnecessary complexity.
Opt for a State Management Library: For complex state management needs (e.g., large-scale applications), consider using state management libraries like Redux or Mobx alongside Context.
Keep Context Small: Divide your context into smaller, more manageable contexts rather than creating a monolithic context for your entire app.
Shopping Cart Example Using React Context API
CartContext.js:
"use client"
// components/CartContext.js
import { createContext, useContext, useReducer } from 'react';
// Define the initial state for the cart
const initialState = {
cart: [],
};
// Create a context for the cart
const CartContext = createContext();
// Create a reducer to manage cart actions
const cartReducer = (state, action) => {
switch (action.type) {
case 'ADD_TO_CART':
// Check if the product is already in the cart
const existingProductIndex = state.cart.findIndex(
(item) => item.id === action.payload.id
);
if (existingProductIndex !== -1) {
// If the product is already in the cart, increment its quantity
const updatedCart = [...state.cart];
updatedCart[existingProductIndex].quantity += action.payload.quantity;
return { ...state, cart: updatedCart };
} else {
// If the product is not in the cart, add it
return { ...state, cart: [...state.cart, action.payload] };
}
case 'INCREMENT_QUANTITY':
// Increment the quantity of a product in the cart
const incrementCart = state.cart.map((item) => {
if (item.id === action.payload.id) {
return { ...item, quantity: item.quantity + 1 };
}
return item;
});
return { ...state, cart: incrementCart };
case 'DECREMENT_QUANTITY':
// Decrement the quantity of a product in the cart
const decrementCart = state.cart.map((item) => {
if (item.id === action.payload.id) {
return { ...item, quantity: item.quantity - 1 };
}
return item;
});
return { ...state, cart: decrementCart };
case 'REMOVE_FROM_CART':
// Remove a product from the cart
const updatedCart = state.cart.filter(
(item) => item.id !== action.payload.id
);
return { ...state, cart: updatedCart };
default:
return state;
}
};
// Create a CartProvider component
export const CartProvider = ({ children }) => {
const [state, dispatch] = useReducer(cartReducer, initialState);
return (
<CartContext.Provider value={{ state, dispatch }}>
{children}
</CartContext.Provider>
);
};
// Custom hook for accessing the cart context
export const useCart = () => {
return useContext(CartContext);
};
Let's break it down step by step:
Importing Dependencies:
createContext, useContext, and useReducer are imported from the React library. These are essential for creating and managing the context and state.
Initial State:
initialState is defined to represent the initial state of the cart. It contains a single property cart, which is an array that will hold the items in the cart.
Creating a Context
CartContext is created using createContext(). This context will be used to provide the cart state and dispatcher to child components.
Reducer Function:
cartReducer is a function that takes two arguments: state and action. It is used to manage changes to the cart state based on different action types.
The reducer handles actions like adding items to the cart, incrementing/decrementing item quantities, and removing items from the cart. It returns a new state based on the action type and payload.
CartProvider Component:
CartProvider is a React component that wraps its children with the CartContext.Provider.
Inside CartProvider, the useReducer hook is used to manage the cart state. It takes the cartReducer function and the initialState as arguments.
The CartProvider component provides the cart state and the dispatch function to its children via the CartContext.Provider value prop.
Now, let's look at how you can use this context in your application:
Consuming the Cart Context:
In any component where you want to access the cart state or dispatch actions, you can use the useContext hook to access the context.
For example, to access the cart state and dispatch function in a component, you would do something like this:
import React, { useContext } from 'react';
import { CartContext } from './CartProvider'; // Import the CartProvider component
function ShoppingCart() {
const { state, dispatch } = useContext(CartContext);
// You can now access state.cart (the cart items) and dispatch actions to modify the cart.
// For example, dispatch({ type: 'ADD_TO_CART', payload: { id: 1, quantity: 2 } });
return (
// Render your shopping cart component using the state data
);
}
This code sets up a centralized cart state management system using React Context and a reducer. Components throughout your application can access and modify the shopping cart's state and perform actions like adding items, changing quantities, and removing items with ease.
Conclusion
The React Context API is a valuable addition to your toolkit as a React developer. It simplifies state management, improves code maintainability, and enhances the overall structure of your applications. By embracing the power of context, you can take your React projects to new heights, building more scalable and efficient user interfaces. So go ahead, harness the potential of React Context API and watch your development process become more seamless and enjoyable.
Comments