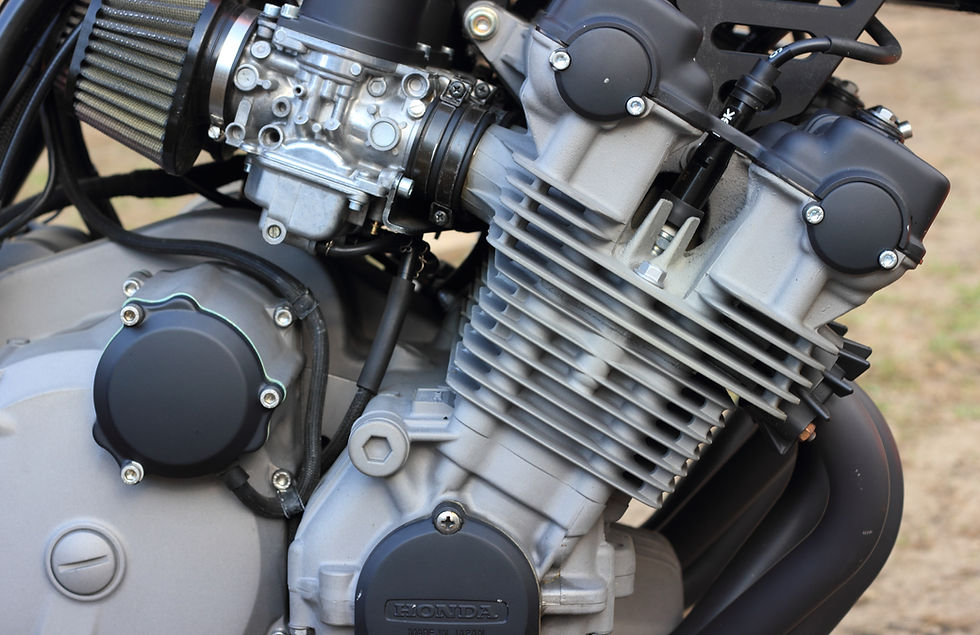
Welcome to the world of React, where building interactive and dynamic user interfaces is a breeze. One of the core concepts that sets React apart is its component-based architecture. In this comprehensive guide, we will delve into the fascinating world of React components, exploring their significance, benefits, and real-world applications. Whether you're a beginner or an experienced developer, this article will equip you with the knowledge and skills to harness the full potential of React components and create powerful web applications.
Understanding React.JS Components
React.JS Components React.js components are the building blocks of a React application. They are reusable, self-contained pieces of code that encapsulate the functionality and UI elements needed to create a specific part of a user interface. Components can be as small as a button or as complex as an entire page.
React follows a component-based architecture, which promotes modularity, reusability, and maintainability. Each component can have its own state, properties (props), and lifecycle methods. By breaking down a user interface into smaller, manageable components, developers can create a more organized and structured code base.
The Role of Components in React Applications React.js components play a crucial role in React applications. They enable developers to divide the UI into smaller, logical parts, making it easier to understand and maintain the code. Each component focuses on a specific functionality or UI element, allowing for easier debugging, testing, and reusability.
Components can be nested within one another, forming a hierarchical structure. This nesting allows for the composition of complex UIs by combining smaller, reusable components into larger ones. By breaking down the UI into components, developers can build scalable applications that are easier to extend and maintain over time.
Key Benefits of Using Components Using components in React applications brings several benefits:
a) Reusability: Components can be reused throughout an application, or even across multiple projects. This reusability saves development time and effort by eliminating the need to recreate similar functionality or UI elements.
b) Modularity: Components promote modularity, allowing developers to build a UI by combining and arranging smaller, self-contained components. This modularity enhances code organization and maintainability.
c) Encapsulation: Components encapsulate their own state and behavior, making them independent and isolated. This encapsulation prevents unintended interactions between components and helps in debugging and testing.
d) Scalability: By dividing the UI into components, React applications can easily scale as new features or UI elements are added. Components can be modified, replaced, or extended without affecting the rest of the application.
e) Maintainability: Components make code more readable and maintainable. With a modular structure, it becomes easier to locate and update specific parts of the application. This improves collaboration among team members and facilitates code maintenance.
In summary, understanding React.js components is essential for building efficient and maintainable applications. By breaking down the UI into reusable components, developers can take advantage of the benefits of reusability, modularity, encapsulation, scalability, and maintainability. In the following sections, we will explore how to create and use React components, harnessing their full potential in your applications.
Creating and Using React Components Functional Components: The Building Blocks of React
Functional components are the fundamental building blocks in React. They are simpler and easier to write compared to class components. Functional components are primarily used for rendering UI elements based on props. Let's explore functional components in more detail.
A functional component is essentially a JavaScript function that takes props as input and returns JSX (a syntax extension for JavaScript) to describe the UI. Functional components are stateless, meaning they don't have their own state. They focus on rendering UI based on the received props.
Here's an example of a simple functional component that displays a greeting message:
// Functional component example
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
In this example, the Greeting component takes a name prop as input and renders a greeting message with the provided name. The props object contains the data passed from the parent component.
Functional components are lightweight and perform well. They are especially useful for presentational or stateless components that focus on rendering UI based on props. Since functional components don't have their own state or lifecycle methods, they are easier to test and reason about.
Functional components can also be written using ES6 arrow function syntax:
const Greeting = (props) => {
return <h1>Hello, {props.name}!</h1>;
}
This syntax is more concise and often preferred for functional components.
To use a functional component, you simply include it in another component's JSX by invoking it as if it were an HTML tag:
function App() {
return (
<div>
<Greeting name="John" />
<Greeting name="Jane" />
</div>
);
}
In this example, the App component renders two instances of the Greeting component, passing different names as props.
Functional components can also receive multiple props, which can be accessed using dot notation (props.propName). This allows you to pass various data to the component, such as strings, numbers, or even objects.
Functional components offer simplicity, re-usability, and ease of testing. They are ideal for components that focus solely on rendering UI elements based on the received props. However, if you need to manage state or implement life-cycle methods, you would need to use class components, which we'll explore in the next section.
By understanding and utilizing functional components effectively, you can create modular and reusable UI elements in your React applications. In the following sections, we will dive deeper into React components and explore various concepts, such as managing state, passing data between components, and leveraging component life-cycle methods.
Comentários