Unlocking the Hidden Superpowers of Props in React: Passing props in React
- CODING Z2M
- May 7, 2023
- 5 min read
Updated: Jun 19, 2023
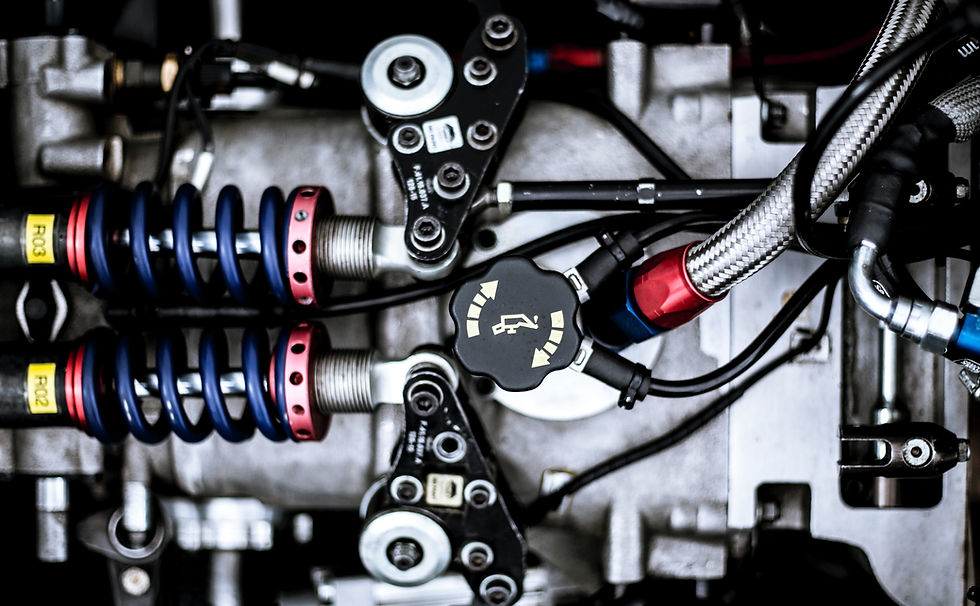
Passing data between components is a fundamental concept in React development. It allows you to create dynamic and interactive user interfaces by sharing information across different parts of your application. In React, data is passed through props (short for properties), which serve as a communication channel between components.
In this comprehensive guide, we'll explore everything you need to know about passing data to React components using props. We'll discuss the basics of props, different ways to pass data, and provide real-world examples to solidify your understanding. So let's dive in and discover the power of props in React!
In React, props are a mechanism for passing data from a parent component to its child components. They allow you to customize and configure child components based on the specific needs of the parent component. Props can be any type of data, such as strings, numbers, booleans, objects, or even functions.
By passing props, you establish a unidirectional data flow in your React application. Data flows from the parent component down to its child components, ensuring that changes in the parent component can be reflected in the child components.
Passing Data from Parent to Child Components
Passing data from a parent component to its child components is a common scenario in React development. There are multiple ways to achieve this, depending on the complexity and relationship between components.
Passing Props through Component Attributes
The simplest and most common way to pass data to a child component is by using component attributes or "props." When rendering a child component, you can provide props by passing them as attributes within the component's opening tag.
Let's consider an example where we have a parent component called App and a child component called Greeting. We want to pass a name prop from the parent to the child, so the child component can display a personalized greeting.
// Parent Component: App.js
import React from 'react';
import Greeting from './Greeting';
const App = () => {
const name = 'John';
return (
<div>
<Greeting name={name} />
</div>
);
};
export default App;
// Child Component: Greeting.js
import React from 'react';
const Greeting = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
export default Greeting;
In the above example, the name prop is passed from the parent App component to the child Greeting component. The child component can access and display the value of the prop using props.name.
Passing Props through the Children of a Component
In some cases, you may need to pass data to a child component by using the children of a component rather than using component attributes directly. This approach is useful when you want to pass complex or nested data structures.
Let's see an example where we have a parent component called List and multiple child components called ListItem. We want to pass an array of items to the List component, and each ListItem component will render a specific item. // Parent Component: List.js
import React from 'react';
import ListItem from './ListItem';
const List = ({ items }) => {
return (
<div>
{items.map((item, index) => (
<ListItem key={index}>{item}</ListItem>
))}
</div>
);
};
export default List;
// Child Component: ListItem.js
import React from 'react';
const ListItem = ({ children }) => {
return <div>{children}</div>;
};
export default ListItem;
In the above example, the List component receives an items prop, which is an array of items. The component iterates over the array using map and renders multiple ListItem components. Each ListItem component receives the specific item as its children and renders it accordingly.
Accessing and Using Props in Child Components
Once props are passed to a child component, they can be accessed and used within the component. Props are accessed by using the props object, which is automatically available within the component.
Let's explore how to access and utilize props in a child component: // Parent Component: App.js
import React from 'react';
import Greeting from './Greeting';
const App = () => {
const name = 'John';
return (
<div>
<Greeting name={name} />
</div>
);
};
export default App;
// Child Component: Greeting.js
import React from 'react';
const Greeting = (props) => {
const { name } = props;
return <h1>Hello, {name}!</h1>;
};
export default Greeting;
In the above example, the name prop is accessed within the Greeting component by destructuring props and extracting the name property. This allows us to use name directly in the component's JSX and display the personalized greeting.
Handling Events and Updating Props
In addition to passing data through props, you can also handle events and update props to enable interactivity within your components. By modifying the props, you can trigger changes in the component's state and re-render the component.
Let's explore an example where we have a parent component called Counter and a child component called Button. The Counter component maintains a count state, and the Button component updates the count when clicked. // Parent Component: Counter.js
import React, { useState } from 'react';
import Button from './Button';
const Counter = () => {
const [count, setCount] = useState(0);
const incrementCount = () => {
setCount(count + 1);
};
return (
<div>
<h1>Count: {count}</h1>
<Button onClick={incrementCount} />
</div>
);
};
export default Counter;
// Child Component: Button.js
import React from 'react';
import PropTypes from 'prop-types';
const Button = ({ onClick }) => {
return <button onClick={onClick}>Increment</button>;
};
Button.propTypes = {
onClick: PropTypes.func.isRequired,
};
export default Button;
In the above example, the Counter component uses the useState hook to define a count state variable and an incrementCount function to update the count. The Button component receives the onClick prop, which is a function, and triggers the incrementCount function when clicked.
By updating the count state in the parent component, the UI is re-rendered with the updated count value, creating an interactive counter.
Passing Data between Sibling Components In some cases, you may need to pass data between sibling components, bypassing the parent component. This can be achieved by utilizing a technique called "lifting state up" or by using libraries such as Redux or React Context.
Let's consider an example where we have a parent component called App, and two sibling components called Sender and Receiver. We want to pass a message from the Sender component to the Receiver component without involving the parent component. // Parent Component: App.js
import React from 'react';
import Sender from './Sender';
import Receiver from './Receiver';
const App = () => {
return (
<div>
<Sender />
<Receiver />
</div>
);
};
export default App;
// Sender Component: Sender.js
import React, { useState } from 'react';
const Sender = () => {
const [message, setMessage] = useState('');
const handleMessageChange = (event) => {
setMessage(event.target.value);
};
return (
<div>
<input type="text" value={message} onChange={handleMessageChange} />
</div>
);
};
export default Sender;
// Receiver Component: Receiver.js
import React, { useState } from 'react';
const Receiver = () => {
const [receivedMessage, setReceivedMessage] = useState('');
const handleReceiveMessage = (message) => {
setReceivedMessage(message);
};
return (
<div>
<h1>Received Message: {receivedMessage}</h1>
</div>
);
};
export default Receiver;
In the above example, the Sender component maintains a message state using the useState hook. It provides an input field where the user can enter a message. The Receiver component also maintains a receivedMessage state and has a handleReceiveMessage function that updates the state when a message is received.
To pass the message from the Sender to the Receiver, we would need to lift the state up to the common parent component (App) and pass the message as props to both the Sender and Receiver components. This way, both components can access and update the shared message state.
Comments