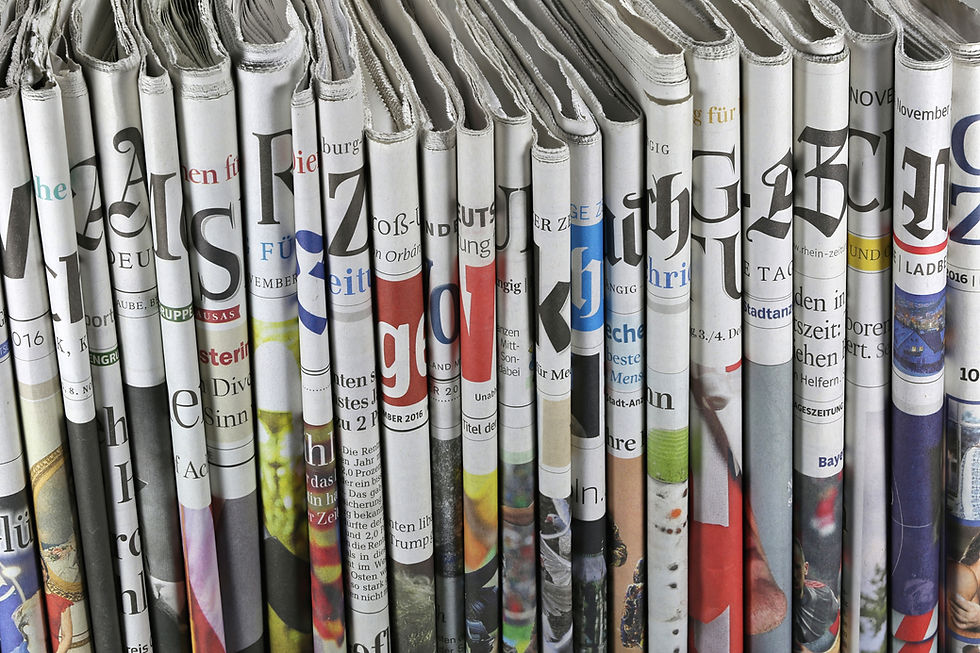
This app is designed to teach students how to build a REST API for a Blogging Platform with Node.js, Express, MongoDB, Mongoose, JWT Authentication, Jest, Middleware, Pagination, Model-Controller-Service architecture can be a great way to create a secure and scalable back-end for your blogging platform.
Node.js and Express provide a robust foundation for building REST APIs, with a powerful and flexible routing system that can handle HTTP requests and responses. MongoDB, a NoSQL database, can be used as the data store for the blog platform, providing fast and scalable data storage.
Mongoose, a popular Object Document Mapper (ODM) for MongoDB, can be used to define and interact with the database schema, making it easy to manage complex data structures and relationships.
JWT Authentication can be used to secure the API endpoints and ensure that only authorized users can access the blog platform. By using JWTs, you can create a stateless authentication system that can scale easily and integrate with other systems.
Using Jest testing framework to write more robust and reliable tests for your Node.js REST API, leading to a more stable and maintainable codebase.
Limiting the number of records returned by a Node.js Express REST API and implementing pagination that can be used to enhance the functionality and performance of the API endpoints and improve performance by reducing the amount of data transferred over the network.
Creating an organized structure for your application by defining classes can help you organize the code, make it more modular and reusable. By encapsulating related functions and data into a single class, you can make your code more maintainable, scalable, and robust. Additionally, this approach can help separate concerns and improve the testability of your code.
By using these technologies and techniques, you can create a robust and scalable backend for your blogging platform
Node.js Resources Basic steps to create a REST API in Node.js with Express
Install Node.JS and NPM (Node Package Manager) on your system.
Create a new directory for your project and navigate to it in the command line.
Initialize a new Node.js project using the following command: npm init.
Creating .gitignore, .env files and ignore '/node_modules' and '.env' in the .gitignore
Create a new file called server.js in your project directory.
Add our 'start' script in package.json file with the following code. "start": "node server.js", "dev": "nodemon server.js"
Running Server by running the following command: npm run dev
Installing 'Thunder Client' extension in VS Code
Installing packages locally npm install dotenv express mongoose express-async-handler bcrypt jsonwebtoken Installing Dev Dependencies npm install -D nodemon Mongo DB Setup & Creating Cluster Connect MongoDB with VSCode Plugin - Download & Install MongoDB for VS Code Note: set 0.0.0.0/0 as IP address under network access in the MongoDB cluster.
Connect Mongo DB Compass with Mongo DB Cluster: Note: In your Mongo DB Cluster, choose "Connect with MongoDB Compass" option, copy the connection string and connect the database through MongoDB VSCode plugin inside VSCode Connect App with MongoDB Cluster: Note: In your Mongo DB Cluster, choose "Add your connection string into your application code" option copy the connection string and add it in the .env file mongoose - mongodb object modeling for node.js, schema-based solution to model your application data. mongoose - Getting Started Create 'db.js' inside 'config' folder Mongoose models provide several static helper functions for CRUD operations. Defining data models using a schema with mongoose - Create 'models' folder and crate models. JSON Web Tokens (Only Authenticated Users can access the 'private' routes) Obtaining Access Token Secret Define unique Access Token Secret in '.env' file. Note: Create a ValidateTokenHandler.js in the middleware to validate whether the token is associated with user. Note: Payload (User Login Info) will be bound into the JSON Web Token Finally, Use the custom middleware validateToken in the 'userRoutes' for Authorization
Comments