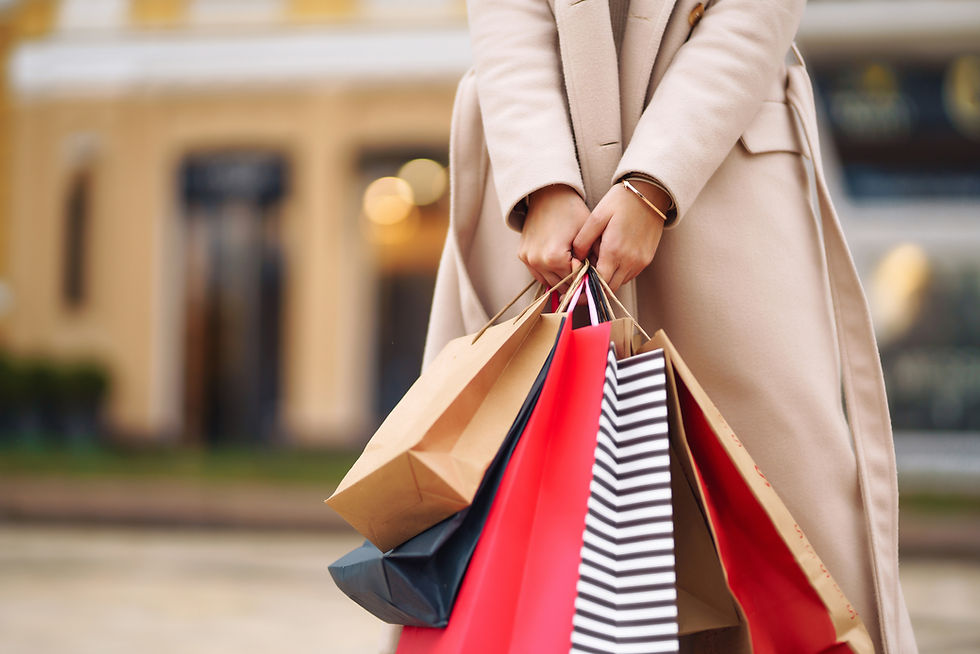
Learn how to build a RESTful API using Node.js and the Express web framework. Students will learn how to set up a Node.js development environment, configure an Express project, and create an Express server.
The course will cover using the Thunder Client extension for VS Code to test API endpoints, setting up an Express router to handle HTTP requests, and implementing error handling with the express-async-handler middleware. Students will also learn about using Express middleware for authentication and authorization, and how to hash passwords for secure storage.
The course will also cover using MongoDB Cloud as the database for the API, and how to use the Mongoose schema to interact with the database. Students will learn how to create CRUD (create, read, update, delete) operations for different schema types, and how to handle relationships between different schemas.
The course will also cover user authentication and authorization using JSON Web Tokens (JWTs), and how to implement protected routes that require user authentication. Students will learn how to register new users, log in existing users, and manage user sessions.
Throughout the course, students will also learn best practices for writing clean and maintainable code, and how to test their API using tools such as Thunder Client and Postman.
Basic steps to create a REST API in Node.js with Express
Install Node.JS and NPM (Node Package Manager) on your system.
Create a new directory for your project and navigate to it in the command line.
Initialize a new Node.js project using the following command: npm init.
Creating .gitignore, .env files and ignore '/node_modules' and '.env' in the .gitignore
Create a new file called server.js in your project directory.
Add our 'start' script in package.json file with the following code. "start": "node server.js", "dev": "nodemon server.js"
Running Server by running the following command: npm run dev
Installing 'Thunder Client' extension in VS Code
Installing packages locally npm install dotenv express mongoose express-async-handler bcrypt jsonwebtoken
Installing Dev Dependencies npm install -D nodemon
Mongo DB Setup & Creating Cluster
Connect MongoDB with VSCode Plugin - Download & Install MongoDB for VS Code
Connect Mongo DB Compass with Mongo DB Cluster:
Note: In your Mongo DB Cluster, choose "Connect with MongoDB Compass" option, copy the connection string and connect the database through MongoDB VSCode plugin inside VSCode
Connect App with MongoDB Cluster:
Note: In your Mongo DB Cluster, choose "Add your connection string into your application code" option copy the connection string and add it in the .env file Note: set 0.0.0.0/0 as IP address under network access in the MongoDB cluster.
mongoose - mongodb object modeling for node.js, schema-based solution to model your application data.
Create 'dbConnection.js' inside 'config' folder
Mongoose models provide several static helper functions for CRUD operations.
Defining data models using a schema with mongoose - Create 'models' folder and crate models
JSON Web Tokens (Only Authenticated Users can access the 'private' routes)
Obtaining Access Token Secret
Define unique Access Token Secret in '.env' file.
Note: Create a ValidateTokenHandler.js in the middleware to validate whether the token is associated with user.
Note: Payload (User Login Info) will be bound into the JSON Web Token
Finally, Use the custom middleware validateToken in the 'userRoutes' for Authorization
Comments