Building Blocks of Node.js Express REST API
- CODING Z2M
- Mar 30, 2023
- 7 min read
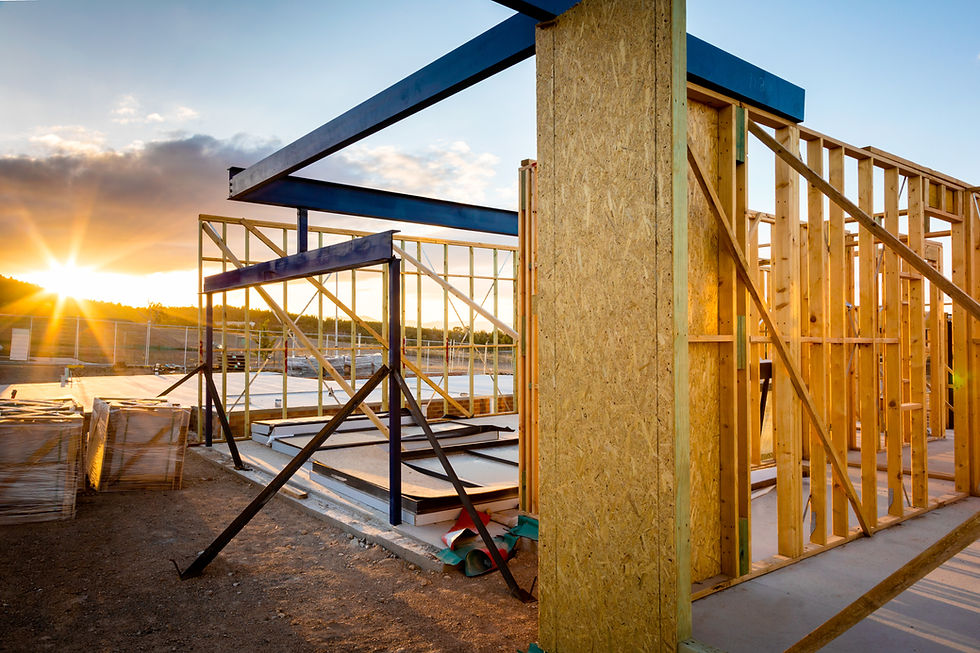
What is Node.js?
Node.js is an open-source, cross-platform, back-end JavaScript runtime environment that is built on the Chrome V8 JavaScript engine. It was created by Ryan Dahl in 2009 and has since become one of the most popular platforms for building server-side applications, including Real-time applications, web servers, RESTful APIs, and microservices. Node.js also has a large ecosystem of third-party modules and packages available through the npm (Node Package Manager) registry, which makes it easy to add functionality to your Node.js applications
Some of the key features of Node.js include:
Asynchronous I/O - Node.js uses an event-driven, non-blocking I/O model that allows it to handle a large number of connections and requests simultaneously without blocking the execution of other operations.
NPM - Node.js has a built-in package manager called NPM that provides access to a vast library of reusable modules and tools for building applications.
Cross-platform - Node.js can run on a variety of operating systems, including Windows, macOS, and Linux, making it highly portable and flexible.
Scalability - Node.js is highly scalable, meaning that it can handle a large volume of traffic and requests without sacrificing performance or stability.
Fast and efficient - Node.js is known for its speed and efficiency, thanks to the Chrome V8 engine, which is designed to execute JavaScript code quickly.
Modular - Node.js allows developers to build applications using modular code and components, which can be easily reused and shared across projects.
Real-time applications - Node.js is well-suited for building real-time applications, such as chat applications and online gaming platforms, because it can handle a large number of simultaneous connections and events.
key Features of Express
Express.js is a popular open-source web framework for Node.js, which allows developers to build web applications and APIs easily and quickly. It provides a set of features and tools that simplify the process of building web applications and APIs.
Routing: Express.js provides a simple and flexible mechanism for defining routes in your application. You can define routes for different HTTP methods (GET, POST, PUT, DELETE, etc.) and specify the handling function for each route.
Middleware: Express.js middleware functions are functions that have access to the request and response objects, and the next middleware function in the application's request-response cycle. They can be used to perform various tasks such as logging, authentication, validation, and more.
Templating: Express.js supports a variety of templating engines (such as EJS, Pug, and Handlebars) that allow you to generate HTML pages dynamically based on data.
Error handling: Express.js provides a robust error handling mechanism that helps you handle errors in a consistent and structured way.
Static file serving: With Express.js, you can serve static files (such as images, CSS, and JavaScript files) directly from your application's file system.
Security: Express.js provides built-in security features such as CSRF protection, XSS prevention, and more.
Database integration: Express.js can be easily integrated with popular databases such as MongoDB, MySQL, and PostgreSQL.
Building blocks of a Node.js Express REST API to create a powerful and scalable API The following are the main building blocks of a Node.js Express REST API. By combining these building blocks, you can create a powerful and scalable API that can be used to build web and mobile applications.
Express: Express is a fast, minimal, and flexible web framework for Node.js. It provides a robust set of features for web and mobile applications.
Routing: In Express, routes are used to define how the application responds to client requests. Routes are defined by using the app.get(), app.post(), app.put(), and app.delete() methods.
Middleware: Middleware functions are functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle. They are used to modify the request and response objects, perform authentication, and handle errors.
Controllers: Controllers are used to handle the business logic of the application. They receive data from the client, perform operations on it, and send back a response to the client.
Mongoose: Mongoose is an Object Data Modeling (ODM) library for MongoDB and Node.js. It provides a schema-based solution to model data and includes features like validation, middleware, and querying. You should learn how to use Mongoose to create models and interact with MongoDB.
Data modeling: Models are used to represent the data that is being manipulated by the application. They provide a way to interact with a database or other data source. A REST API typically needs to interact with a database to store and retrieve data. You should learn how to use a data modeling library such as Mongoose to define the schema for your data and interact with the database.
Authentication and Authorization: Authentication and authorization are important aspects of building web applications. You should learn how to use JWTs to authenticate and authorize users for your Node.js REST API.
Error handling: Error handling is an important part of any application. In a Node.js Express REST API, error handling can be performed using middleware or a third-party library such as Boom or Sentry.
TypeScript: TypeScript is a typed superset of JavaScript that compiles to plain JavaScript. It provides a more structured approach to writing JavaScript and helps to catch errors before runtime. You should learn how to use TypeScript to write type-safe code for your Node.js REST API.
Testing: Testing is an essential part of building web applications. You should learn how to use testing frameworks like Jest and Supertest to write unit and integration tests for your Node.js REST API.
Deployment: Deployment is the process of making your Node.js REST API available to users. You should learn how to deploy your application to a production environment using tools like Docker and Kubernetes.
What is REST API & Its Principles The following principles of REST APIs provide a simple and flexible way to build web services that can be easily consumed by clients on any platform.
Resources: REST APIs work with resources, which are the things that you want to manage, such as users, products, or orders. Each resource is identified by a unique URI (Uniform Resource Identifier).
HTTP Methods: REST APIs use HTTP methods (also called verbs) to specify the type of operation to be performed on a resource. The most common HTTP methods are GET, POST, PUT, PATCH, and DELETE. For example, GET is used to retrieve a resource, POST is used to create a new resource, PUT is used to update an existing resource, PATCH is used to partially update an existing resource, and DELETE is used to delete a resource.
Representations: A resource can have different representations, such as JSON, XML, or HTML. A client can request a specific representation of a resource using the Accept header in the HTTP request.
Stateless: REST APIs are stateless, which means that each request from a client to a server contains all the necessary information to complete the request. The server does not store any client context between requests.
Uniform Interface: REST APIs have a uniform interface, which means that they use a common set of standards for interacting with resources. This includes using HTTP methods, URIs, and media types.
Client-Server Architecture: REST APIs follow a client-server architecture, where the client and server are separate components that communicate with each other over the network.
Cacheable: REST APIs are designed to be cacheable, which means that the server can indicate to the client whether a response can be cached or not.
HATEOAS: HATEOAS (Hypermedia as the Engine of Application State) is a constraint of the REST architectural style that requires the server to provide hyperlinks in the response that enable the client to discover and interact with related resources.
What is web service?
A web service is a software system designed to allow communication between different applications over the internet. It provides a standardized way for applications to exchange information, regardless of the programming language, platform or operating system they use. Web services typically use XML or JSON for data exchange and are based on a set of industry standards such as HTTP, XML, SOAP, and WSDL.
Web services can be used for a wide range of purposes, such as integrating different software systems, sharing data between applications, or exposing functionality to other applications or devices. They are often used in the context of service-oriented architectures (SOA) and can be deployed on various platforms, including cloud computing environments.
Web services can be accessed using a variety of protocols, such as SOAP, REST, and XML-RPC, and can be implemented using various programming languages and technologies, such as Java, .NET, and PHP.
What is json? JSON (JavaScript Object Notation) is a lightweight, text-based data interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is commonly used for transmitting data between a server and a web application, as an alternative to XML.
JSON is based on a key-value pair data structure, which consists of a collection of name-value pairs separated by commas and enclosed in curly braces. The name or key is always a string and the value can be a string, number, Boolean, null, object or an array.
Here is an example of a simple JSON object: {
"name": "John Doe",
"age": 30,
"city": "New York"
}
JSON is also widely used in web APIs to exchange data between different applications and services.
What is event-driven model in Node.js?
In Node.js, the event-driven model is a programming paradigm that enables asynchronous I/O operations. It is based on the idea of triggering and handling events, where events are actions or occurrences that happen in the system, such as a file being read, a network request being made, or a timer expiring.
In the event-driven model, Node.js provides an event loop that continuously listens for events and dispatches them to their respective event handlers. Event handlers are functions that are registered to handle a specific event. When an event is triggered, the event loop will execute the associated event handler function, which can perform some action in response to the event.
One of the key benefits of the event-driven model in Node.js is that it allows developers to write non-blocking, asynchronous code that can handle a large number of concurrent connections without blocking the event loop. This makes Node.js particularly well-suited for building high-performance web applications and servers.
In summary, the event-driven model in Node.js allows developers to write efficient, scalable, and responsive applications by leveraging the power of asynchronous programming and event-driven architecture.
Comments