Asynchronous Programming in Node.js
- CODING Z2M
- Mar 4, 2023
- 2 min read
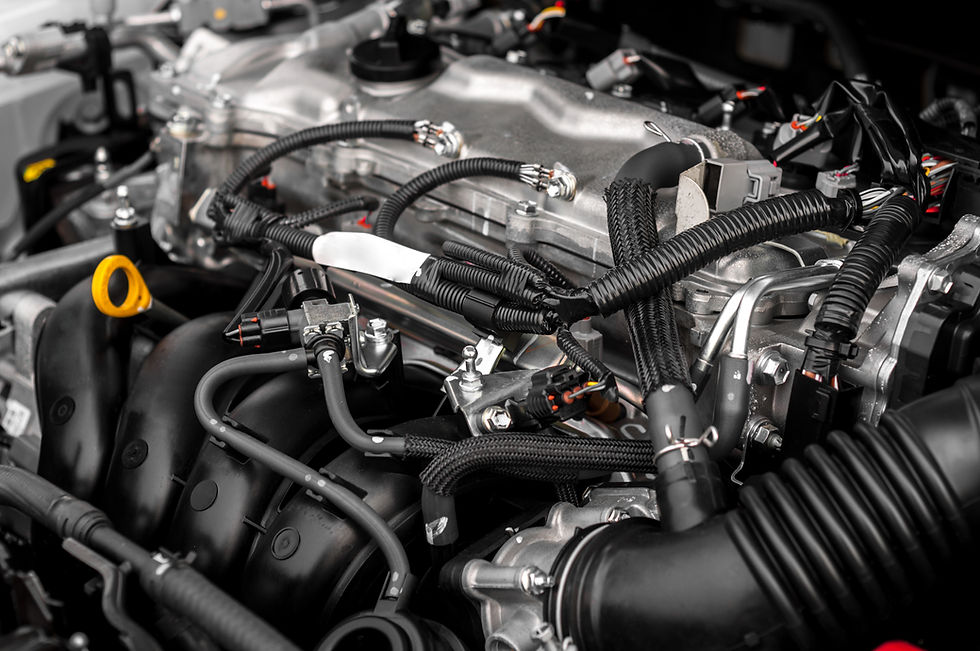
Why Asynchronous programming is important for Node.js?
Asynchronous programming is a programming paradigm that allows you to write code that doesn't block the execution of the program while waiting for an operation to complete. In Node.js, asynchronous programming is especially important because Node.js is designed to handle a large number of concurrent connections, and blocking code can quickly lead to performance issues.
Node.js provides several mechanisms for asynchronous programming, including callbacks, Promises, and async/await syntax. These mechanisms allow you to write non-blocking code that can handle many requests at the same time.
How to use async and await in Node.js - Implementing Asynchronous Programming
To use async and await in Node.js, you need to define an async function that returns a Promise. Inside the async function, you can use the await keyword to wait for asynchronous operations to complete before continuing with the rest of the function. In the below example, assume getContact function is reading data from a database and returns a Promise that can take some time for the operation to complete. If you use synchronous code to perform these operations, the entire program would be blocked until the operation is finished. Asynchronous code, on the other hand, allows you to initiate the data reading from the database operation and then move on to other tasks, while the database operation continues in the background. When the read data operation is complete, it calls the callback function, passing any errors and the read data. Meanwhile, the rest of the program can continue to execute, without being blocked by the read operation.
Note: Here,the get function uses await to wait for the Promise to resolve and then logs the result to the console. If an error occurs, the catch block logs the error to the console.
const express = require('express');
const app = express();
const port = 3000;
// Define a function that returns a Promise
async function getContact(name) {
return new Promise((resolve, reject) => {
if (name) {
resolve(`Hello, ${name}!`);
} else {
reject(new Error('No name provided'));
}
});
}
// Define a route that uses async/await and the Promise
app.get('/greeting', async (req, res) => {
try {
const name = req.query.name;
const greeting = await getContact(name);
res.json({greeting });
} catch (error) {
res.status(400).send(error.message);
}
});
// Start the server
app.listen(port, () => {
console.log(`Example app listening at http://localhost:${port}`);
}); |
In the above example, we define a function called getContact that returns a Promise. The Promise resolves with a greeting string if a name is provided, and rejects with an error if no name is provided.
We then define a route for the /greeting endpoint that uses async/await and the getContact Promise. The async keyword tells Node.js that this function returns a Promise and can be used with await. Inside the route function, we use await to wait for the getGreeting Promise to resolve or reject. If it resolves, we send the greeting string as a response. If it rejects, we send an HTTP 400 error with the error message as the response body.
Example In the below example,the async connectDb function uses await to wait for the Promise 'mongoose.connect' to resolve and then logs the result to the console. If an error occurs, the catch block logs the error to the console.
const mongoose = require ("mongoose");
const connectDb = async () => {
try {
const connect = await mongoose.connect(process.env.CONNECTION_STRING);
console.log("DB Connected", connect.connection.host, connect.connection.name);
} catch(err) {
console.log(err);
process.exit(1);
}
};
module.exports = connectDb; |
Comentarios