React Router Dom Params
- CODING Z2M
- Dec 9, 2024
- 3 min read
Updated: Jan 27
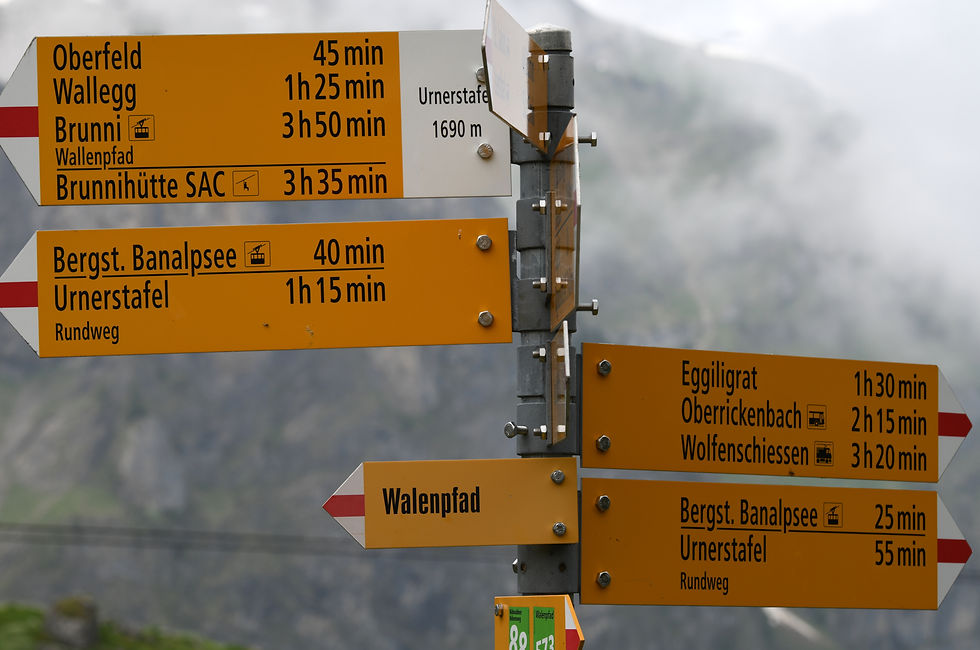
React Router DOM Params & UseNavigation React Router
What are useParams and useNavigate Hooks?
Both useParams and useNavigate are hooks provided by React Router (version 6+) to help you handle navigation and dynamic routes in React applications.
useParams:This hook allows you to access the dynamic parameters of the current route, like the ID in a URL path (/user/:id). It is useful when you're dealing with dynamic URLs in your app.
useNavigate:This hook is used to programmatically navigate between different routes in your app. It allows you to perform navigation actions like redirecting users after form submission or clicking a button.
useParams Hook
The useParams hook gives access to the dynamic parameters in the URL, such as IDs or other parameters, that are defined in the route.
Example: Displaying User Details Based on User ID
Imagine you have a list of users and want to display each user's details on a separate page. The user is selected by clicking their name from a list, and the URL changes to something like /user/:id.
Setup:
Route: /user/:id
Params: The id in the URL is dynamic and will change depending on which user is clicked.
Example Code:
import React from 'react';
import { useParams } from 'react-router-dom';
function UserDetails() {
const { id } = useParams(); // Get the dynamic 'id' parameter from the URL
// Mock user data (in a real app, you would fetch this from an API)
const user = {
1: { name: 'John Doe', age: 28 },
2: { name: 'Jane Smith', age: 34 },
3: { name: 'Sam Green', age: 22 }
};
const userDetails = user[id] || { name: 'Unknown', age: 'N/A' };
return (
<div>
<h1>User Details</h1>
<p>Name: {userDetails.name}</p>
<p>Age: {userDetails.age}</p>
</div>
);
}
export default UserDetails;
Explanation:
The URL could be something like /user/1, /user/2, etc.
useParams() gives you the id from the URL, which is used to retrieve and display the correct user's details.
App Component (with Routes):
import React from 'react';
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom';
import UserDetails from './UserDetails';
function App() {
return (
<Router>
<Routes>
<Route path="/user/:id" element={<UserDetails />} />
</Routes>
</Router>
);
}
export default App;
useNavigate Hook
The useNavigate hook allows you to programmatically navigate to a different route. This is helpful when you want to navigate after a user action, like submitting a form or clicking a button.
Example: Redirecting After Form Submission
Imagine you have a form where a user submits their information, and you want to redirect them to a "Thank You" page after they submit.
Example Code:
import React, { useState } from 'react';
import { useNavigate } from 'react-router-dom';
function SubmitForm() {
const [name, setName] = useState('');
const navigate = useNavigate(); // Initialize useNavigate hook
const handleSubmit = (e) => {
e.preventDefault();
// After form submission, navigate to the 'thank-you' page
navigate('/thank-you');
};
return (
<div>
<h1>Submit Your Information</h1>
<form onSubmit={handleSubmit}>
<input
type="text"
placeholder="Enter your name"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<button type="submit">Submit</button>
</form>
</div>
);
}
export default SubmitForm;
App Component (with Routes):
import React from 'react';
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom';
import SubmitForm from './SubmitForm';
import ThankYou from './ThankYou';
function App() {
return (
<Router>
<Routes>
<Route path="/submit" element={<SubmitForm />} />
<Route path="/thank-you" element={<ThankYou />} />
</Routes>
</Router>
);
}
export default App;
Thank You Page:
import React from 'react';
function ThankYou() {
return <h1>Thank you for your submission!</h1>;
}
export default ThankYou;
Explanation:
The useNavigate hook is used in the SubmitForm component to navigate the user to the /thank-you route after the form is submitted.
When to Use useParams and useNavigate Together
These hooks are often used together in apps that require dynamic routing, such as e-commerce platforms (product details pages) or social media apps (user profiles).
Example: Navigating to a Product Detail Page
You can navigate to a product detail page using useNavigate.
Once there, you can use useParams to get the product ID from the URL and fetch the relevant product details.
Conclusion
useParams: Accesses dynamic route parameters, making it easy to handle dynamic URLs in your app.
useNavigate: Programmatically navigates to different routes, giving you control over the flow of the application.
Commenti