Redux Toolkit Tutorial
- CODING Z2M
- Mar 16
- 5 min read
Updated: Mar 17
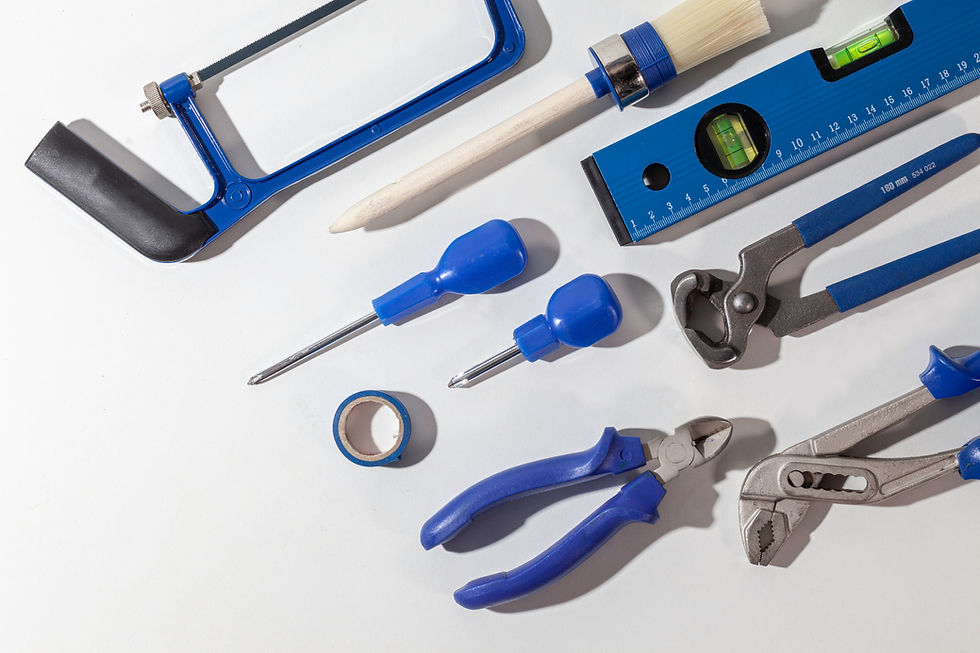
Redux Toolkit Tutorial: What is Redux?
Redux is a state management tool for JavaScript apps, helping you manage and share state across components without prop drilling (passing props down multiple components). It uses a single source of truth called the Store.
What is React Redux?
React Redux provides easy-to-use tools to connect your React components with the Redux store. It ensures that your components automatically re-render when the state changes.
Core Concepts (Redux Fundamentals)
Store
The Store is a JavaScript object that holds the entire state of your application. There is only one store for the whole app. Think of it as a central brain.
Actions
Actions are plain JavaScript objects that describe an event or intention to change the state.
They have a type property that describes what happened and can carry data through a payload property. Example: { type: 'counter/increment' }
{ type: 'counter/decrement' }
Action creators
export const { increment, decrement } = counterSlice.actions; // Action creators
counterSlice.actions - Action creators automatically generated by createSlice().
counterSlice.actions is an object containing action creators for each reducer function.
The increment and decrement exported using .actions are action creators(functions).
The action creators generate action objects that trigger the reducer functions when dispatched.
console.log(increment());
// Output: { type: 'counter/increment' }
console.log(decrement());
// Output: { type: 'counter/decrement' }
What’s Actually Happening?
When you import and use increment() or decrement() action creators in your React components, you’re just calling functions that produce action objects.
These objects are then dispatched to the store where the actual reducer functions (inside reducers: {}) handle the state change.
Reducers
Reducers are pure functions that take the current state and an action, and return a new state. They specify how the state changes in response to actions.
Dispatch
The dispatch() function is used to send actions to the store, triggering the reducers to update the state.
Building the Counter App
We'll build a simple Counter App with increment and decrement buttons.
Step 1: Create the Store & Reducer (store.js)
// src/store.js
import { configureStore, createSlice } from '@reduxjs/toolkit';
// Creating a slice - A slice is a collection of reducer logic and actions for a single feature of your app.
const counterSlice = createSlice({
name: 'counter', // Name of the slice
initialState: { value: 0 }, // Initial state of this slice
reducers: {
increment: (state) => { // Reducer function
state.value += 1;
},
decrement: (state) => { // Reducer function
state.value -= 1;
}
}
});
// Exporting the "Action Creators" to be used in components
export const { increment, decrement } = counterSlice.actions;
// Creating and exporting the store
const store = configureStore({
reducer: { counter: counterSlice.reducer }
});
export default store;
Let's break down the store.js code line-by-line and explain what’s happening and why.
import { configureStore, createSlice } from '@reduxjs/toolkit';
We are importing configureStore and createSlice from @reduxjs/toolkit.
configureStore is a function that helps us create the Redux store.
createSlice is a function that makes it easy to create a slice of state (a part of our global state) with its actions and reducers in one place.
// Creating a slice of state for the counter feature
const counterSlice = createSlice({
name: 'counter', // The name of this slice of state. It's just a label.
initialState: { value: 0 }, // The starting state. Here, it's an object with a single property: value (initially 0).
reducers: { // Reducers: Functions that define how to update the state based on actions.
increment: (state) => { // ✅ Reducer function for incrementing the counter
state.value += 1; // Directly modifying the state. Redux Toolkit uses "Immer" to make this safe.
},
decrement: (state) => { // ✅ Reducer function for incrementing the counter
state.value -= 1;
}
}
});
Breaking Down createSlice()
name: This is the identifier for this part of the state.
Think of it as giving a name to a folder of related data.
initialState: The default value of this slice's state.
Here, we have an object { value: 0 }, meaning our state starts with a value of 0.
reducers: These are functions that describe how the state should change.
In Redux, reducers are pure functions, but Redux Toolkit uses a library called Immer that allows us to mutate state directly like state.value += 1. Under the hood, Immer creates a copy and updates the copy, making the code simpler and safer.
increment: Increases value by 1.
decrement: Decreases value by 1.
export const { increment, decrement } = counterSlice.actions;
counterSlice.actions - Action creators automatically generated by createSlice().
counterSlice.actions is an object containing action creators for each reducer function.
The increment and decrement exported using .actions are action creators(functions).
// Creating the Redux store
const store = configureStore({
reducer: { counter: counterSlice.reducer }
});
This creates a Redux store with a counter slice of state. Breaking Down configureStore()
configureStore() is a helper function that Sets up the store with the reducer we created.
You are telling Redux:
“Hey, here’s my entire state structure. I have a piece of state called counter, and all its updates should be handled by this reducer function: counterSlice.reducer.”
What does counterSlice.reducer actually represent?
It is a single reducer function created by createSlice().
Under the hood, it combines all the individual reducer functions (increment, decrement) into one big reducer function.
What does counterSlice.reducer do?
It’s a function that takes two arguments:
The current state.
An action object.
It checks the type of the action object.
If the action type matches one of the actions you defined (increment or decrement), it calls the corresponding reducer function to update the state.
Otherwise, it returns the current state unchanged.
Step 2: Provide the Store to Your App (index.js)
// src/index.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { Provider } from 'react-redux'; // Allows React components to access the Redux store
import store from './store'; // Importing the store we created
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
What is <Provider>?
<Provider> is a React component that comes from the react-redux library.
Its purpose is to make the Redux store available to all React components within your application.
Why do we need <Provider>?
When you create a Redux store:
const store = configureStore({
reducer: { counter: counterSlice.reducer }
});
The store contains your entire application state and the logic to update that state.
But for your React components to access and interact with this store, they need a way to "see" the store.
How is the store provided to the app?
You wrap your entire React application with <Provider> and pass the store as a prop:
What's Happening Here?
<Provider> takes the store and puts it into React's Context System.
It makes the store accessible to any child component within the <Provider> through React-Redux hooks like:
useSelector() (to read state)
useDispatch() (to dispatch actions)
Step 3: Create the Counter Component (App.js)
// src/App.js
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { increment, decrement } from './store';
function App() {
const count = useSelector(state => state.counter.value); // Accessing state from the store
const dispatch = useDispatch(); // Allows us to trigger actions
return (
<div style={{ textAlign: 'center', marginTop: '50px' }}>
<h1>Counter: {count}</h1>
<button onClick={() => dispatch(increment())}>Increment</button>
<button onClick={() => dispatch(decrement())}>Decrement</button>
</div>
);
}
export default App;
What's Happening Here?
createSlice() - Helps you create actions and reducers more easily by bundling them together.
configureStore() - Creates the Redux store with your reducers.
useSelector() - Allows you to read state from the Redux store.
useDispatch() - Provides a dispatch function to send actions to the Redux store.
Actions (increment() / decrement()) - Triggered by clicking the buttons and handled by the reducers.
Komentar