React Hooks Form Validation
- CODING Z2M
- Dec 24, 2024
- 3 min read
Updated: Jan 22
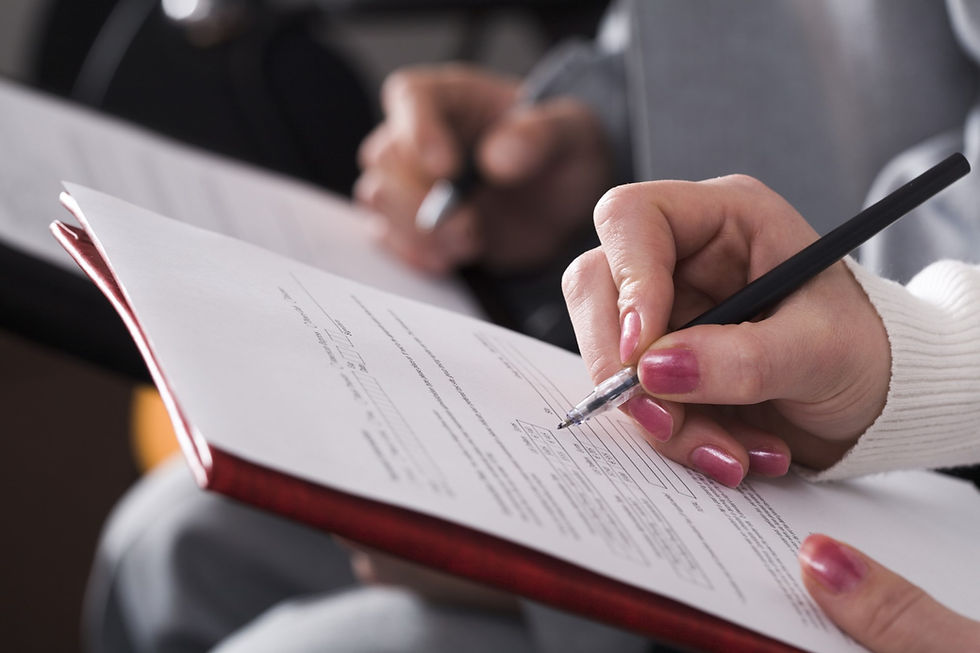
React Hooks Form Validation
React Hook Form is a popular library in React for handling form validation and form state management in an easy, scalable, and performance-optimized way. It uses React hooks to manage form data, making it lightweight and simple to use.
Key Features of React Hook Form:
Minimal Re-Renders: React Hook Form minimizes re-renders by only re-rendering components when necessary, making it highly performant compared to traditional form libraries.
Easy Integration with UI Libraries: It works seamlessly with UI component libraries like Material-UI, Ant Design, and more, allowing you to easily manage form inputs with your preferred library.
Less Boilerplate Code: It reduces the need for verbose form handling code (such as onChange handlers and state updates) by utilizing hooks like useForm for managing form state.
Built-in Validation: React Hook Form integrates easily with schema validation libraries like Yup, Zod, and Joi, allowing you to validate form data without much effort.
Uncontrolled Components: By default, React Hook Form uses uncontrolled components, which means it doesn't rely on React’s state management for form inputs, leading to improved performance.
Custom Input Components: You can create your custom form inputs and hook them up with React Hook Form using the Controller component.
Key Hooks in React Hook Form:
useForm: This is the primary hook used to initialize the form, manage the form state, handle validation, and perform actions such as resetting the form or submitting it.
Controller: This is used to handle custom components or components that don’t directly integrate with React Hook Form.
useFieldArray: This hook helps manage dynamic form fields, allowing you to add/remove fields at runtime.
Basic Example:
import React from 'react';
import { useForm } from 'react-hook-form';
function MyForm() {
// useForm hook initializes the form and provides methods for validation and submission
const { register, handleSubmit, formState: { errors } } = useForm();
// Form submission handler
const onSubmit = data => {
console.log(data); // Data will be the form values
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<div>
<label htmlFor="name">Name:</label>
<input
id="name"
{...register('name', { required: 'Name is required' })}
/>
{errors.name && <span>{errors.name.message}</span>}
</div>
<div>
<label htmlFor="email">Email:</label>
<input
id="email"
type="email"
{...register('email', {
required: 'Email is required',
pattern: {
value: /^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,4}$/,
message: 'Invalid email address'
}
})}
/>
{errors.email && <span>{errors.email.message}</span>}
</div>
<button type="submit">Submit</button>
</form>
);
}
export default MyForm;
Explanation:
useForm Hook: Initializes the form state and provides functions like register for registering input fields, handleSubmit for form submission, and formState to access validation errors.
register Method: Registers each input field and binds them to the form state. It also accepts validation rules (e.g., required, pattern).
handleSubmit Method: This method is used to handle form submission. It takes a function (onSubmit) that will be called when the form is successfully submitted.
React Hooks Form Validation Errors: If there are validation errors (e.g., the name or email fields are not filled in correctly), they are captured in errors and displayed next to the input field.
Steps to Get Form Values:
Use useForm Hook: Initialize the form with the useForm hook, which provides methods like register, handleSubmit, and formState.
Define the onSubmit Handler: Create a function that will receive the form data as an argument when the form is submitted.
Use handleSubmit: Wraps the onSubmit function to handle validation and pass the form data once the form is valid.
Access Submitted Values: The values are passed to the onSubmit function when the form is successfully submitted.
Form Submission: When the form is submitted, the handleSubmit(onSubmit) function is called. It validates the form inputs based on the rules defined in the register method. If the form passes validation, the onSubmit function is executed, and the form values are passed to it as an object (data).
Submitted Data:
In the onSubmit function, the data parameter holds the form values. For example, if the user fills out the form with:
Name: "John"
Email: "john@example.com"
The data object passed to onSubmit will look like:
{
name: "John",
email: "john@example.com"
}
How to Use the Data:
You can use the data object in any way you need. For example, you can send it to an API or display it on the page after submission:
const onSubmit = (data) => {
console.log("Form submitted with values:", data);
// Example of sending data to an API
// fetch('/api/submit', { method: 'POST', body: JSON.stringify(data) });
};
Comments