React Custom Hooks Best Practices
- CODING Z2M
- Dec 9, 2024
- 2 min read
Updated: Jan 27
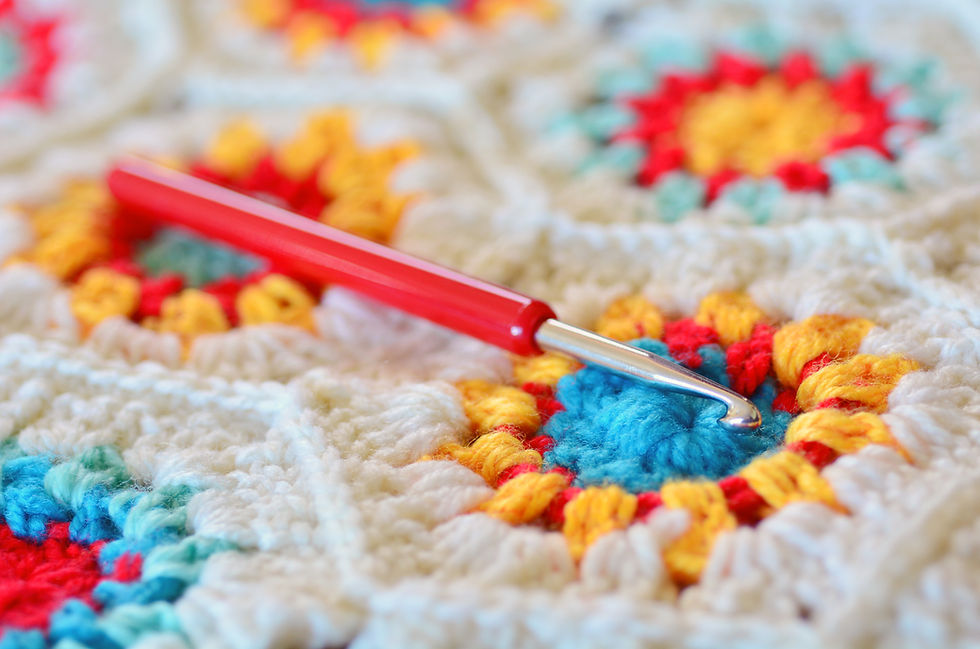
React Custom Hooks Best Practices
What is a Custom Hook in React & How can developers use custom hooks to simplify complex features and avoid duplicating code?
React Custom Hooks Best Practices: A custom hook is a reusable JavaScript function in React that allows you to encapsulate and reuse stateful logic across multiple components. It starts with the prefix use (e.g., useFetch, useAuth), signaling that it follows React's rules of hooks, such as calling hooks only inside React function components or other custom hooks.
Custom hooks are a way to:
Abstract complex logic.
Share stateful functionality between components.
Simplify code by avoiding duplication.
Why Use Custom Hooks?
Custom hooks help:
Encapsulate Reusable Logic: Logic can be written once and used across multiple components.
Reduce Boilerplate: Instead of repeating the same logic in multiple places, use a custom hook.
Enhance Readability: Moves implementation details out of components, making them cleaner and more focused.
Maintain Consistency: Ensures that shared logic behaves consistently.
How to Create a Custom Hook
Step 1: Identify Reusable Logic
Find logic that's repeated in multiple components or is too complex to keep inline.
Step 2: Create the Hook
Write a function that contains the reusable logic, adhering to the rules of hooks.
Step 3: Use the Hook
Call the custom hook in components where the logic is needed.
To save a custom hook in a React project, follow these steps:
Create a Separate File for the Hook
Organize your code by saving the custom hook in its own file. This makes it reusable and maintainable.
Name the file based on the hook's functionality, and ensure the function name starts with use.
src/
hooks/
useFetch.js
components/
App.js
Write the Hook in the File
Example: useFetch.js:
Imagine multiple components need to fetch data from an API. Instead of repeating the fetching logic, we create a custom hook.
import { useState, useEffect } from 'react';
function useFetch(url) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
setLoading(true);
try {
const response = await fetch(url);
if (!response.ok) throw new Error('Network response was not ok');
const result = await response.json();
setData(result);
} catch (err) {
setError(err.message);
} finally {
setLoading(false);
}
};
fetchData();
}, [url]);
return { data, loading, error };
}
export default useFetch;
Export the Hook
Use export to make the custom hook available for import in other files. In most cases, you'll use export default for a single hook per file.
Import and Use the Hook in Components
Import the custom hook into components where it's needed.
Example: Using the Hook in App.jsx:
import useFetch from '../hooks/useFetch'
function App() {
const { data, loading, error } = useFetch('https://api.example.com/users');
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error}</p>;
return (
<ul>
{data.map((user) => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
Comments