Using Spring Security 6 in Spring Boot REST API
Updated: May 3, 2023
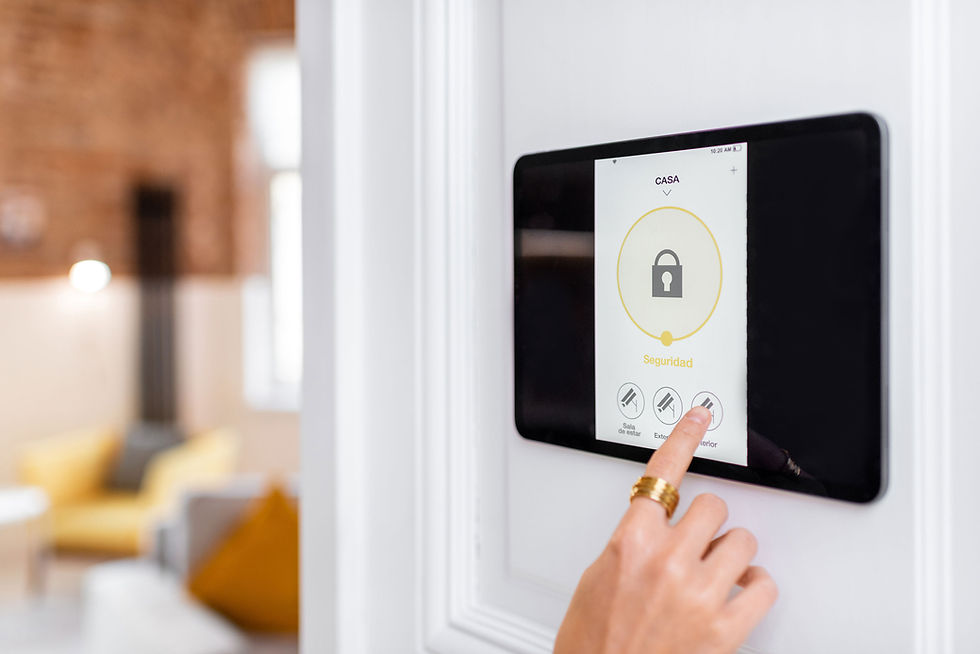
Overview Spring Security 6 is the latest version of Spring Security, which is used to provide security features to web applications. It provides features like authentication, authorization, and other security-related tasks. In this section, we will discuss how to use Spring Security 6 in a Spring Boot application. Example
@Configuration
@EnableWebSecurity
@EnableMethodSecurity
public class UserAuthentication {
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
// Authentication
@Bean
public UserDetailsService userDetailsManager(PasswordEncoder encoder) {
UserDetails admin = User
.withUsername("admin")
.password(encoder.encode("admin123"))
.roles("ADMIN")
.build();
UserDetails guest = User
.withUsername("guest")
.password(encoder.encode("guest123"))
.roles("GUEST")
.build();
return new InMemoryUserDetailsManager(admin, guest);
}
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity httpSecurity) throws Exception {
httpSecurity.csrf().disable()
.authorizeHttpRequests( (authorize) -> authorize
.requestMatchers("/savingsaccount/welcome", "/h2-console").permitAll()
.anyRequest().authenticated()
)
.headers(headers -> headers.frameOptions().sameOrigin())
.httpBasic(Customizer.withDefaults());
return httpSecurity.build();
}
} Explanation The code is a configuration class in a Spring Boot application that sets up user authentication using Spring Security. Let's break down each part of the code:
Annotations:
The @Configuration annotation indicates that the class is a configuration class and can be used to configure the Spring application context.
The @EnableWebSecurity annotation enables Spring Security in the application.
The @EnableMethodSecurity annotation enables method-level security in the application.
PasswordEncoder Bean:
The passwordEncoder() method is a Bean that returns a BCryptPasswordEncoder instance, which is used to encode user passwords.
UserDetailsService Bean:
The userDetailsManager() method is a Bean that returns an InMemoryUserDetailsManager instance, which is an implementation of the UserDetailsService interface. It creates two users, admin and guest, with their respective roles and encoded passwords. These users will be used for authentication later.
SecurityFilterChain Bean:
The securityFilterChain() method is a Bean that returns a SecurityFilterChain instance, which is used to configure the security of the application.
The method configures the HttpSecurity by disabling the CSRF protection and setting up the request authorization rules. It also enables the HTTP basic authentication and sets the default headers. The configuration allows access to specific URLs without authentication and requires authentication for all other URLs. Request Authorization:
The authorizeHttpRequests() method is used to specify which requests are authorized based on the request matchers. In this case, the URLs "/savingsaccount/welcome" and "/h2-console" are permitted to be accessed without authentication, and any other URL requires authentication.
Headers Configuration:
The headers() method is used to set up the security headers of the application. In this case, it enables the X-Frame-Options header with the value of "SAMEORIGIN" to prevent clickjacking attacks.
HTTP Basic Authentication:
The httpBasic() method is used to enable HTTP basic authentication. This method accepts a Customizer object that allows customization of the HTTP basic authentication configuration.
Return Statement:
Finally, the method returns the HttpSecurity object that was configured using the previous methods. This object is used to create a SecurityFilterChain instance, which is then returned as a Bean.
In summary, this code configures Spring Security to authenticate users using a UserDetailsService implementation, sets up request authorization rules, and enables HTTP basic authentication. The configuration allows access to specific URLs without authentication and requires authentication for all other URLs.
Comments