Useful Middleware in Node.js Express REST API
Updated: May 3, 2023
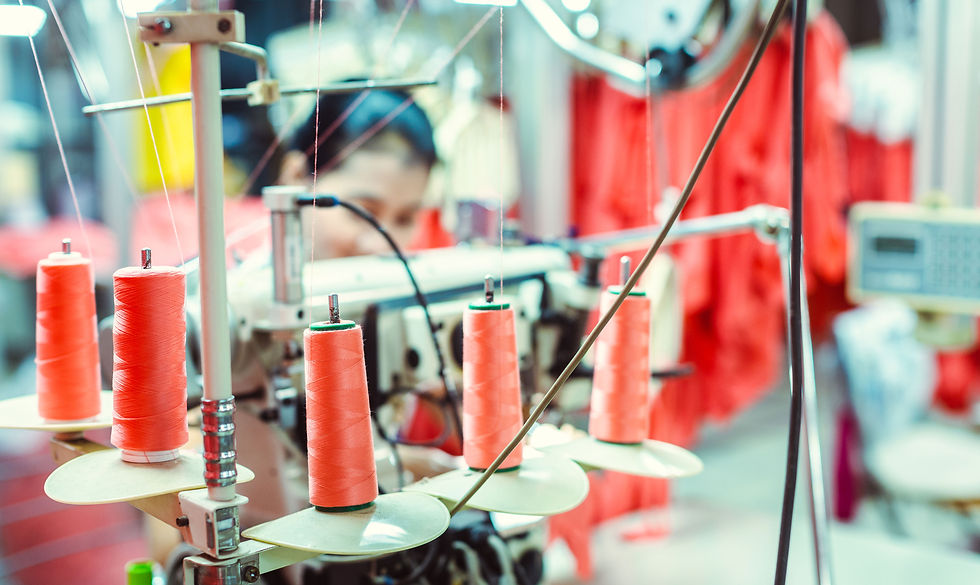
CORS (Cross-Origin Resource Sharing) Middleware
CORS (Cross-Origin Resource Sharing) is a mechanism that allows web applications running in a browser to access resources from a different origin than the one that served the original web page. The Same-Origin Policy (SOP) enforced by modern browsers limits this capability in order to prevent cross-site scripting (XSS) attacks.
To enable cross-origin resource sharing, servers must include specific HTTP headers in their responses that indicate which origins are allowed to access their resources. The most common headers used for CORS are Access-Control-Allow-Origin, Access-Control-Allow-Methods, Access-Control-Allow-Headers, and Access-Control-Allow-Credentials.
In Node.js, the cors npm package provides middleware to enable CORS in a server application. This package can be used with popular web frameworks like Express, Koa, and Hapi.
Using the cors middleware, you can specify which origins are allowed to access your API, which HTTP methods are allowed for each endpoint, and which headers are allowed to be sent in requests. The middleware automatically adds the necessary headers to your responses to enable CORS.
Here's an example of how to use the cors middleware with Express: const express = require('express');
const cors = require('cors');
const app = express();
// Enable CORS for all routes
app.use(cors());
// Enable CORS for a specific route
app.get('/my-route', cors(), (req, res) => {
// ...
});
// Enable CORS for a specific origin
app.use(cors({
origin: 'https://example.com'
}));
// Enable CORS for multiple origins
app.use(cors({
origin: ['https://example.com', 'https://example.org']
}));
app.listen(3000, () => {
console.log('Server started on port 3000');
});
urlencoded Middleware In Express, the urlencoded middleware is used to parse incoming requests with URL-encoded payloads. URL encoding is a way to represent data in a format that can be transmitted over the web using only ASCII characters.
When a form is submitted with application/x-www-form-urlencoded content type, the data is sent in a key-value pair format in the request body. The urlencoded middleware can be used to extract this data from the request body and make it available in the req.body object.
Here's an example of how to use the urlencoded middleware with Express:
const express = require('express');
const app = express();
// parse application/x-www-form-urlencoded
app.use(express.urlencoded({ extended: false }));
// handle POST requests to /my-form
app.post('/my-form', (req, res) => {
const name = req.body.name;
const email = req.body.email;
// ...
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
In this example, we've used the urlencoded middleware to parse incoming requests with application/x-www-form-urlencoded payloads (app.use(express.urlencoded({ extended: false }))). We've then handled a POST request to /my-form and accessed the form data in the req.body object.
The urlencoded middleware accepts an options object with a extended property that can be set to true or false. When extended is true, the middleware uses the qs library to parse the URL-encoded payload with rich objects and arrays support. When extended is false, the payload is parsed with Node.js built-in querystring library and only supports simple key-value pairs. The extended option is set to false by default. json middleware In Node.js, the json middleware is used to parse incoming requests with JSON payloads. JSON (JavaScript Object Notation) is a lightweight data format that is widely used for exchanging data between client and server applications.
When a client sends a request with a JSON payload to a server, the json middleware can be used to extract the data from the request body and make it available in the req.body object.
Here's an example of how to use the json middleware with Node.js:
const express = require('express');
const app = express();
// parse application/json
app.use(express.json());
// handle POST requests to /my-endpoint
app.post('/my-endpoint', (req, res) => {
const data = req.body;
// do something with the data
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
In this example, we've used the json middleware to parse incoming requests with JSON payloads (app.use(express.json())). We've then handled a POST request to /my-endpoint and accessed the JSON data in the req.body object.
Note that the json middleware only handles JSON payloads. If you expect to receive payloads in other formats, you'll need to use different middleware to handle those formats, such as urlencoded for URL-encoded payloads, or text for plain text payloads.
Also note that the json middleware uses the body-parser package under the hood to parse incoming requests. If you're using a version of Express that's 4.16.0 or later, you can use the express.json() middleware instead of body-parser.json(), which has been deprecated.
Here are some other important middleware that are commonly used in Node.js applications:
morgan: This middleware is used to log HTTP requests to the console or a file. It provides information such as the request method, URL, status code, response time, and more.
helmet: This middleware provides security-related HTTP headers to protect your application from common attacks such as XSS, clickjacking, and CSRF.
compression: This middleware compresses response bodies before sending them to the client, reducing the amount of data sent over the network and improving performance.
cookie-parser: This middleware parses Cookie headers and populates a cookies object in the request object.
express-session: This middleware enables server-side session management for your application. It creates a unique session ID for each client and stores session data on the server.
passport: This middleware provides authentication and authorization functionality for your application. It supports a wide range of authentication strategies such as local authentication, OAuth, and OpenID.
Comments