State Management in React with Redux Toolkit
- CODING Z2M
- Mar 9, 2023
- 1 min read
Updated: Mar 25, 2023
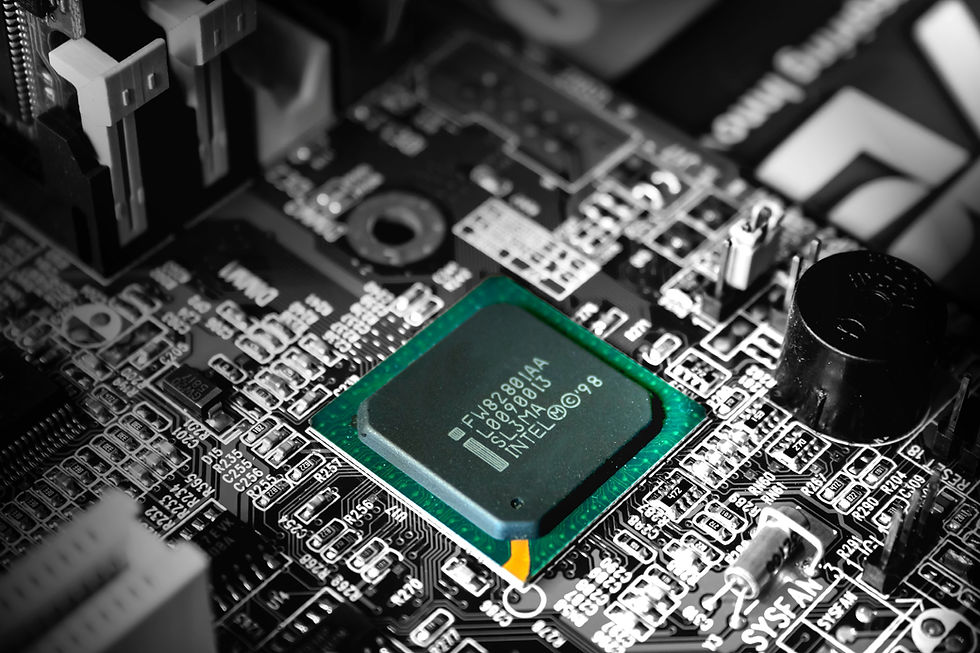
What is Redux in React?
Redux is a state management library for JavaScript applications. It is often used with React to manage the application state in a predictable and centralized manner. Redux provides a way to manage the state of an application and update the user interface in response to user actions, network requests, and other events.
The core idea behind Redux is to have a single source of truth for the state of the application, which is represented as an immutable object. Any changes to the state must be made through actions, which are plain JavaScript objects that describe what happened in the application. These actions are then dispatched to the Redux store, which is responsible for managing the state of the application and updating the user interface accordingly.
One of the benefits of using Redux is that it makes it easier to debug and test your application, as the state of the application is centralized and predictable. It also allows for easier collaboration between developers working on the same application, as everyone is working with the same state management paradigm.
How we can use Redux Toolkit to manage state in a React application?
For example, the following shows how to manage shopping cart state using Redux Toolkit
1. Define your shopping cart state slice using createSlice() function from Redux Toolkit:
import { createSlice } from '@reduxjs/toolkit';
const cartSlice = createSlice({
name: 'cart',
initialState: {
items: [
]
},
reducers: {
addItem: (state, action) => {
const newItem = action.payload;
const existingItem = state.items.find(item => item.id === newItem.id);
if (existingItem) {
existingItem.quantity += 1;
} else {
state.items.push(newItem);
}
},
decrementItem(state, action) {
const index = state.items.findIndex(item => item.id === action.payload);
if (index !== -1) {
state.items[index].quantity--;
}
},
removeItem: (state, action) => {
const id = action.payload;
state.items = state.items.filter(item => item.id !== id);
},
updateQuantity: (state, action) => {
const { id, quantity } = action.payload;
const item = state.items.find(item => item.id === id);
if (item) {
item.quantity = quantity;
}
},
clearCart: (state) => {
state.items = [];
},
}
});
export default cartSlice.reducer
export const { addItem, removeItem, updateQuantity, clearCart, decrementItem } = cartSlice.actions;
|
2. Create a Redux store that includes your shopping cart state slice:
import { configureStore } from '@reduxjs/toolkit';
import cartReducer from '../features/shopping/cart';
export const store = configureStore({
reducer: {
cart: cartReducer,
},
});
|
3. Use useSelector() hook from React-Redux to get shopping cart state in your components & use useDispatch() hook from React-Redux to dispatch actions to modify shopping cart state in the following "ShoppingCart" component
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { addItem, removeItem, updateQuantity, clearCart, decrementItem } from '../features/shopping/cart';
function ShoppingCart() {
const products = [ {id:1, title:"product1", price: "$12.00", quantity:1},
{id:2, title:"product2", price: "$14.00", quantity:1},
{id:3, title:"product3", price: "$13.00", quantity:1}
]
const items = useSelector(state => state.cart.items);
const dispatch = useDispatch();
const handleAddItem = (item) => {
dispatch(addItem(item));
};
const handleRemoveItem = (id) => {
dispatch(removeItem(id));
};
const handleDecrementItem = (id) => {
dispatch(decrementItem(id));
};
const handleQuantityChange = (id, quantity) => {
dispatch(updateQuantity({ id, quantity }));
};
return (
<div>
<h2>Products</h2>
<ul>
{products.map(item => (
<li key={item.id}>
{item.title} - ${item.price}
<button onClick={()=> handleAddItem(item)}>Add Item</button>
</li>
))}
</ul>
<h2>Cart Items</h2>
<ul>
{items.map(item => (
<li key={item.id}>
{item.title} - ${item.price}
{item.quantity }
<button onClick={() => handleRemoveItem(item.id)}>Remove</button>
<button onClick={() => handleDecrementItem(item.id)}>Decrement</button>
<button onClick={() => dispatch(clearCart())}>Clear Cart</button>
<button onClick={()=> handleAddItem(item)}>Add Item</button>
</li>
))}
</ul>
</div>
);
}
export default ShoppingCart;
|
ความคิดเห็น