Props in React with Real-World Example
- CODING Z2M
- May 7, 2023
- 3 min read
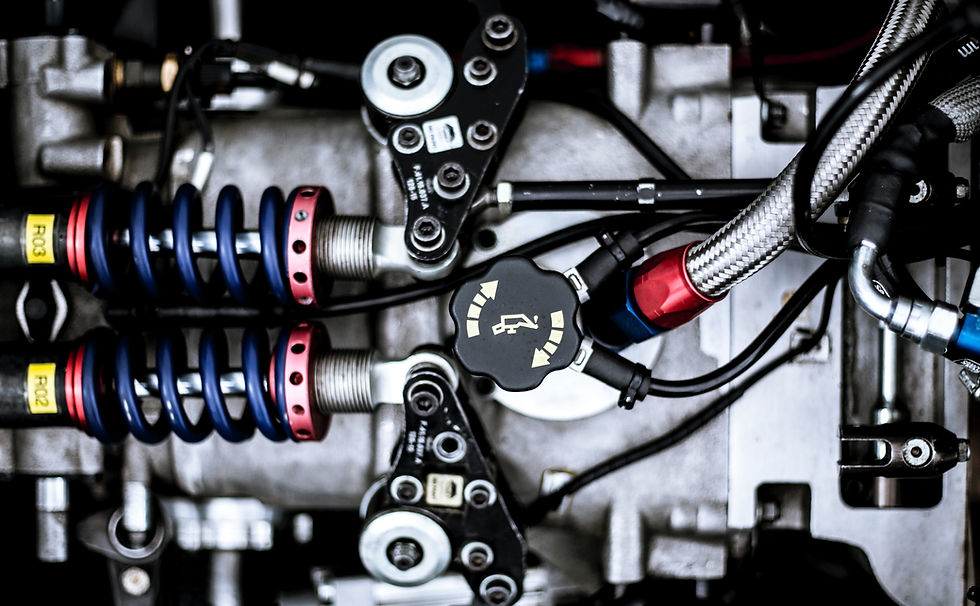
React is a popular JavaScript library for building user interfaces. One of the key features of React is its ability to create reusable components that can be passed data through props. In this blog post, we'll explore what props are in React, why they're important, and provide a real-world example to help you understand how to use them in your own projects.
How to pass data between components in React using Props & What Props are all about?
Props, short for properties, are a way to pass data from a parent component to a child component. They are essentially arguments passed to a component function or class, and they allow you to customize the behavior of a component based on the data passed to it.
In other words, props are a way to make a component dynamic by passing in different data depending on the use case. Props can be any type of data, including strings, numbers, booleans, objects, and even functions.
In React, each component is like a separate function that takes in some inputs (called "props") and returns some output (called "JSX"). They are passed from the parent component to the child component as attributes, and can be accessed inside the child component using the pre-defined "props" object.
Common use cases for props in React:
Passing data: You can pass data from a parent component to a child component using props. This allows you to reuse the same component with different data in different parts of your app.
Customizing behavior: You can pass functions as props to a child component, which allows the child component to call that function when a certain event occurs. This allows you to customize the behavior of your components based on user interactions.
Styling: You can pass CSS styles as props to a child component, which allows you to style the child component based on the parent's state or props.
Conditional rendering: You can pass a boolean value as a prop to a child component, which allows you to conditionally render the child component based on the parent's state or props.
Why are Props Important in React?
Props are important in React because they allow you to create reusable components that can be customized based on the data passed to them. This makes it easier to maintain and update your application, as you can reuse the same components in different parts of your app with different data.
Using props also makes your code more readable and easier to understand, as it allows you to separate the logic of your components from the data they use.
Real-World Example
Let's say you're building an e-commerce website, and you need to display a list of products on a page. You could create a reusable component called ProductCard that displays information about each product, such as the name, image, price, and description.
To make this component dynamic, you can use props to pass in the data for each product. Here's an example of what the ProductCard component might look like:
import React from 'react';
function ProductCard(props) {
return (
<div>
<img src={props.image} alt={props.name} />
<h2>{props.name}</h2>
<p>{props.description}</p>
<h3>{props.price}</h3>
</div>
);
}
export default ProductCard;
In this example, we're defining a new function component called ProductCard. This component takes four props: image, name, description, and price. These props are used to display information about each product.
To use this component in our application, we can import it and pass in the props for each product like this:
import React from 'react';
import ProductCard from './ProductCard';
function ProductList() {
const products = [
{
id: 1,
name: 'Product 1',
image: 'https://example.com/product1.jpg',
description: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit.',
price: '$10.99'
},
{
id: 2,
name: 'Product 2',
image: 'https://example.com/product2.jpg',
description: 'Nulla facilisi. Duis tristique sapien vitae turpis tincidunt.',
price: '$19.99'
},
// add more products here
];
return (
<div>
{products.map(product => (
<ProductCard
key={product.id}
image={product.image}
name={product.name}
description={product.description}
price={product.price}
/>
))}
</div>
);
}
export default ProductList;
In this example, we're creating an array of products, each with its own id, name, image, description, and price. We're then using the map method to loop through the array and create a ProductCard component for each product, passing in the props for each one.
By using props to pass in the data for each product, we've created a reusable ProductCard component that can be used to display information about any product in our application. This makes it easier to maintain and update our code, and allows us to create a consistent look and feel across our entire website.
Conclusion
Props are an essential part of React development, providing a way to pass data from a parent component to a child component and create dynamic, reusable components. By understanding what props are, why they're important, and how to use them in your own projects, you can become a more proficient React developer and build better user interfaces for your users.
Comments