Models for Building a Spring Boot REST API
Updated: May 3, 2023
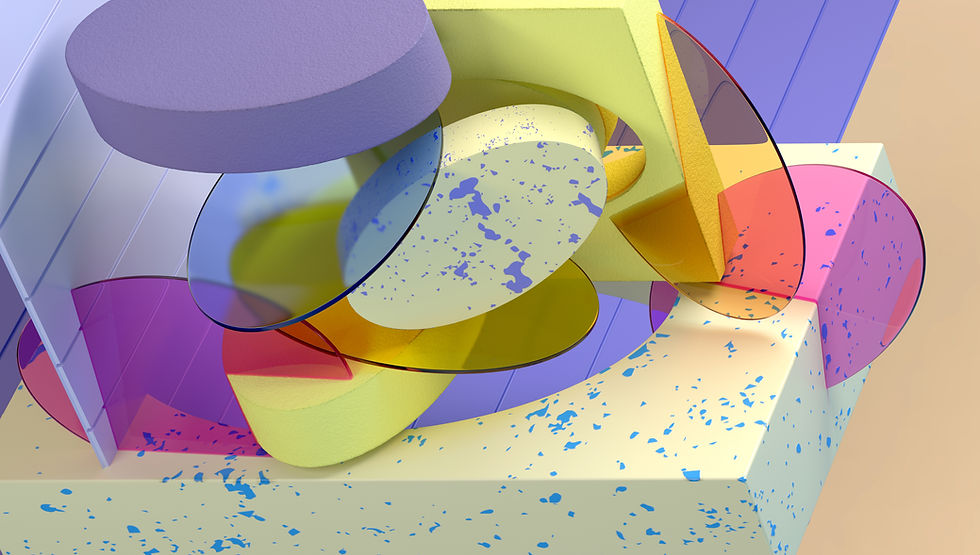
Here are some popular models for building a Spring Boot job board REST API:
MVC (Model-View-Controller) architecture: This is a well-known design pattern that separates an application into three interconnected parts: the model (business logic), the view (user interface), and the controller (handles user input and manages the flow of data between the model and view).
Repository pattern: This pattern is used to separate the data access logic from the business logic. It provides a way to interact with the database or other data sources without exposing the underlying data structures.
DAO (Data Access Object) pattern: This is another pattern used for data access, where the DAO acts as an intermediary between the application and the data source. It abstracts away the details of data retrieval and storage, making the application more flexible and easier to maintain.
Service layer pattern: This pattern is used to encapsulate the business logic of an application into a separate layer. The service layer interacts with the DAO layer and provides a clean API for the controller layer to interact with.
Dependency Injection pattern: This pattern is used to manage the dependencies of an application by injecting them into the classes that need them, rather than hard-coding them. This makes the application more flexible and easier to test.
JWT (JSON Web Token) authentication: This is a popular method of authenticating users in REST APIs. It involves generating a token that contains user information, which is sent with each request to authenticate the user.
RESTful design principles: This is not a pattern per se, but a set of principles that govern the design of REST APIs. These include using HTTP verbs to represent actions (GET, POST, PUT, DELETE), using resource URIs to represent resources, and using hypermedia to provide links to related resources.
In spring boot, how DAO pattern is used along with Repository pattern, service layer pattern and provides an additional layer of separation between the business logic and data access logic, making it easier to maintain and test the application? DAO interface and implementation are not strictly necessary since the Repository pattern provides similar functionality. However, the DAO pattern can still be used in conjunction with the Repository pattern for added flexibility and abstraction. The DAO pattern provides an additional layer of abstraction between the data access logic and the business logic. It allows for greater control over the data access operations and can be useful in cases where the Repository pattern is not sufficient for the needs of the application. Additionally, some developers may prefer the DAO pattern over the Repository pattern due to personal preference or familiarity with the pattern. Ultimately, the decision to use one pattern over the other depends on the specific needs and requirements of the application. How MVC (Model-View-Controller) architecture is used in spring boot REST API? Spring Boot provides a powerful framework for building REST APIs using the Model-View-Controller (MVC) architecture. The MVC architecture separates an application into three interconnected components: the Model, the View, and the Controller. In a Spring Boot REST API, the Model represents the data and business logic of the application. This can include entities, DTOs (Data Transfer Objects), and services that interact with the data. The View component is typically not used in a REST API, as the response is usually in the form of JSON or XML and does not require a separate View component. The Controller component handles HTTP requests and responses and acts as the intermediary between the Model and the View. The Controller receives requests, passes them to the appropriate service, and returns a response to the client.
Comments