How To Style React Components using Tailwind CSS
- CODING Z2M
- Feb 18, 2023
- 2 min read
Updated: Mar 25, 2023
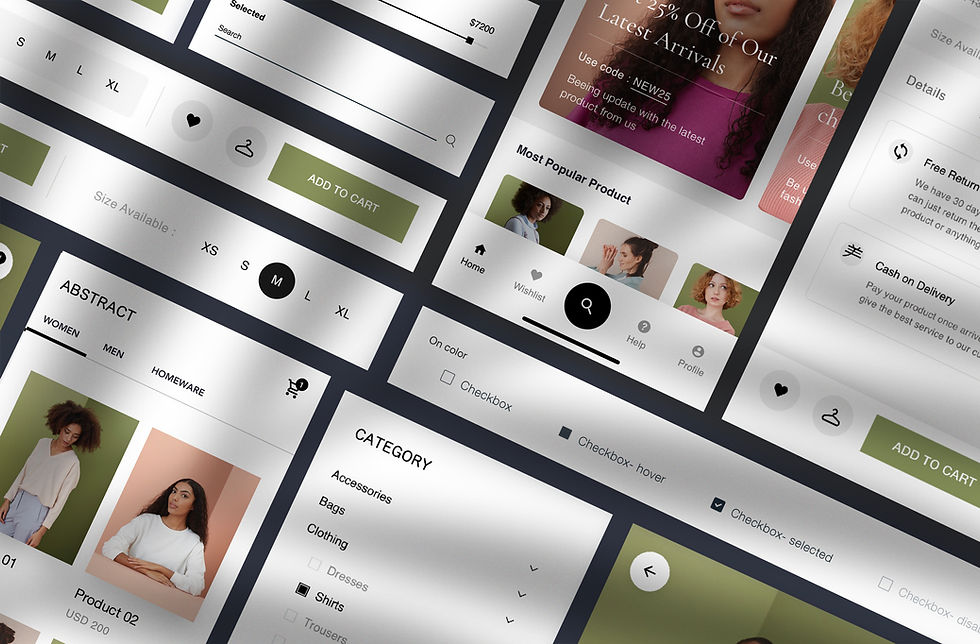
First, read, Getting Started with React to create and run basic react project.
Tailwind CSS is a utility-first CSS framework which lets you to rapidly style react components while building modern websites using its built-in CSS classes. Styling React components with Tailwind CSS is a straightforward process that involves the following steps:
Setting up your React project with Tailwind CSS - Tailwind CSS Configuration with React Project
1. Install Tailwind CSS: The first step is to install Tailwind CSS in your React project. You can do this by running the following command in your terminal:
> npm install -D tailwindcss |
2. Create a configuration file: After installing Tailwind CSS, you need to create a configuration file where you can customize the default styles. You can generate a configuration file by running the following command and your app will get tailwind.config.js file in the root folder of your project.
> npx tailwindcss init
3. Configuring your template paths - Adding the paths to all of your template files in your tailwind.config.js file.
/** @type {import('tailwindcss').Config} */
module.exports = {
content: ["./src/**/*.{html,js,jsx}"],
theme: { extend: {}, },
plugins: [],
} |
4. Add the '@tailwind' directives to your main CSS (index.css) file.
@tailwind base; @tailwind components; @tailwind utilities; |
Now, we can start using built-in Tailwind CSS classes in our React Components.
import React from 'react' const App = () => { return ( <div className='mt-0 flex flex-col min-h-screen w-screen'> <h1>Styling React Components using Tailwind CSS </h1> </div> ) } export default App |
Using Tailwind-Styled-Component
Tailwind-Styled-Component is a library that combines the power of Tailwind CSS with the flexibility of Styled Components in React. With Tailwind-Styled-Component, you can define your styles using the familiar syntax of Styled Components, while also being able to use Tailwind CSS classes to apply pre-defined styles to your components. This makes it easy to create custom components that have a consistent look and feel, while also being responsive to different screen sizes and device types.
Installation using NPM:
> npm i -D tailwind-styled-components
Usage:
import tailwind-styled-components and create a Tailwind Styled Component with Tailwind rules as shown below.In this example, we define components using Styled Components syntax. We then use the tw utility from Tailwind-Styled-Component to apply Tailwind CSS classes to the component.
import React from 'react' import tw from "tailwind-styled-components" import {BsCart2} from "react-icons/bs"; import { Link } from 'react-router-dom'; const Product = ( {product} ) => { return ( <ProductContainer> <ProductDetails> <Link to={`/product/${product.id}`}> <img src={product.image} width={334} height={500}/> </Link> <Details className='flex items-center justify-center'> <span className='w-72 px-4 uppercase text-sm font-normal overflow-hidden text-ellipsis whitespace-nowrap '>{product.title}</span> <span className='text-sm font-semibold'>${product.price}.00</span> <div className='flex gap-2'> <CartIcon/> <span className='text-xs cursor-pointer' onClick="" >ADD TO CART</span> </div> </Details> </ProductDetails> </ProductContainer> ) } export default Product const ProductContainer= tw.div` flex flex-col items-center justify-center my-2 sm:my-0 `; const ProductDetails = tw.div` flex flex-col gap-3 items-center justify-center border-2 pt-0 pb-6 `; const Details = tw.div` flex flex-col gap-1 items-center justify-center mt-2 `; const CartIcon = tw(BsCart2)` w-4 h-4 cursor-pointer `; |
Comments