Hibernate One-To-Many & Many-To-One Bi & Uni Directional Mappings
- CODING Z2M
- Apr 26, 2023
- 3 min read
Updated: May 3, 2023
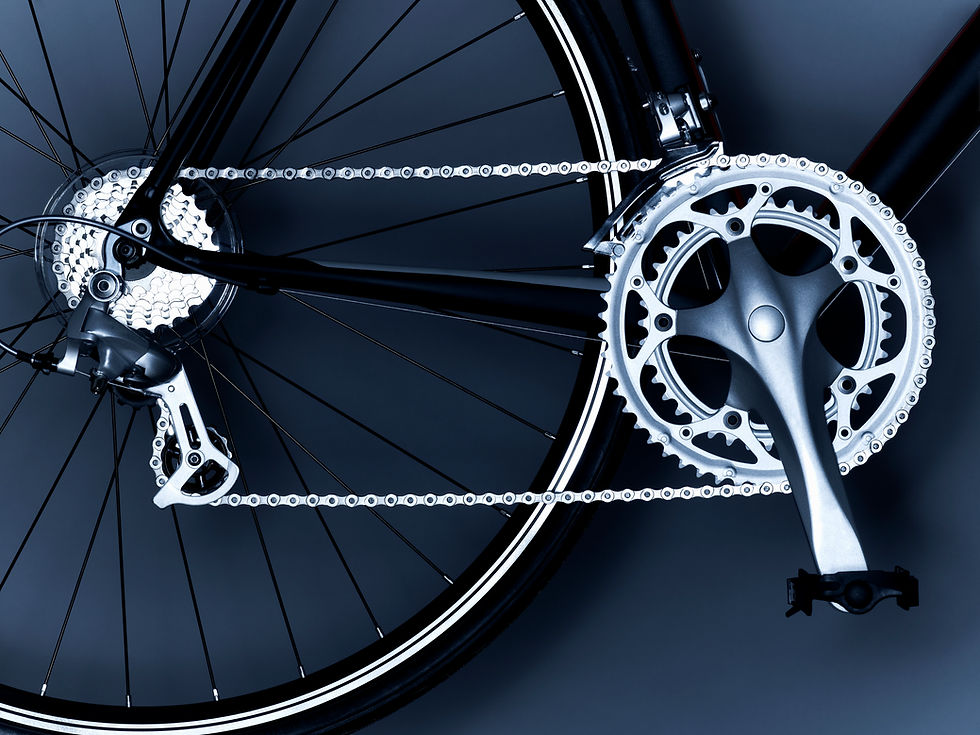
Hibernate is a widely-used Java framework that simplifies the process of interacting with databases. It provides an Object-Relational Mapping (ORM) technique to map database tables to Java objects. One of the most common scenarios in database modeling is a one-to-many relationship, where a single object is related to many other objects, and vice versa. In Hibernate, one-to-many and many-to-one relationships can be implemented using bidirectional or unidirectional mappings.
Let's consider an example of a Spring Boot Rest API that manages a library system. In this system, we have two entities: Book and Author. An author can write many books, but a book can only be written by one author. This is a classic one-to-many relationship.
One-to-Many Bidirectional Mapping
In a bidirectional one-to-many mapping, the relationship is defined in both entities. In our example, the Author entity has a collection of Book objects, and the Book entity has a reference to its Author.
Author Entity @Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@OneToMany(mappedBy = "author", cascade = CascadeType.ALL, orphanRemoval = true)
private List<Book> books = new ArrayList<>();
//getters and setters
}
The @OneToMany annotation is used to specify the relationship between the Author and Book entities. The mappedBy attribute indicates that the relationship is mapped by the author field in the Book entity. The cascade attribute specifies that any changes made to the Author entity should be propagated to its associated Book entities. The orphanRemoval attribute specifies that any Book entities that are no longer associated with an Author entity should be removed from the database.
Book Entity
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "author_id")
private Author author;
//getters and setters
}
The @ManyToOne annotation is used to specify the relationship between the Book and Author entities. The fetch attribute specifies that the associated Author entity should be fetched lazily. The JoinColumn annotation is used to specify the foreign key column in the Book entity that references the id column in the Author entity.
With this bidirectional mapping, we can easily retrieve all the books written by a specific author or the author of a specific book.
One-to-Many Unidirectional Mapping
In a unidirectional one-to-many mapping, the relationship is only defined in one entity. In our example, we can define the relationship in the Book entity only.
Book Entity @Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "author_id")
private Author author;
//getters and setters
}
The @ManyToOne annotation is used to specify the relationship between the Book and Author entities, and the JoinColumn annotation is used to specify the foreign key column in the Book entity that references the id column in the Author entity.
In this unidirectional mapping, we cannot easily retrieve all the books written by a specific author, but we can retrieve the author of a specific book.
In conclusion, one-to-many and many-to-one relationships are common in database modeling, and Hibernate provides bidirectional and unidirectional mappings to implement these relationships. In a bidirectional mapping, the relationship is defined in both entities, while in a unidirectional mapping, the relationship is defined in one entity only.
In a Spring Boot Rest API that manages a library system, we considered an example where an author can write many books, but a book can only be written by one author. We defined the relationship between the Book and Author entities using both bidirectional and unidirectional mappings.
In a bidirectional one-to-many mapping, the Author entity has a collection of Book objects, and the Book entity has a reference to its Author. We used the @OneToMany annotation in the Author entity to specify the relationship between the two entities, and the @ManyToOne annotation in the Book entity to specify the relationship back to the Author entity.
In a unidirectional one-to-many mapping, we defined the relationship only in the Book entity. We used the @ManyToOne annotation to specify the relationship between the Book and Author entities, and the @JoinColumn annotation to specify the foreign key column in the Book entity that references the id column in the Author entity.
In both mappings, we used the CascadeType.ALL attribute in the @OneToMany annotation to specify that any changes made to the Author entity should be propagated to its associated Book entities. We also used the FetchType.LAZY attribute in the @ManyToOne annotation to specify that the associated Author entity should be fetched lazily.
Using Hibernate one-to-many and many-to-one relationships in a Spring Boot Rest API can simplify the process of interacting with databases and improve the performance of the application.
Comments