A Comprehensive Guide to Components in React
- CODING Z2M
- May 7, 2023
- 3 min read
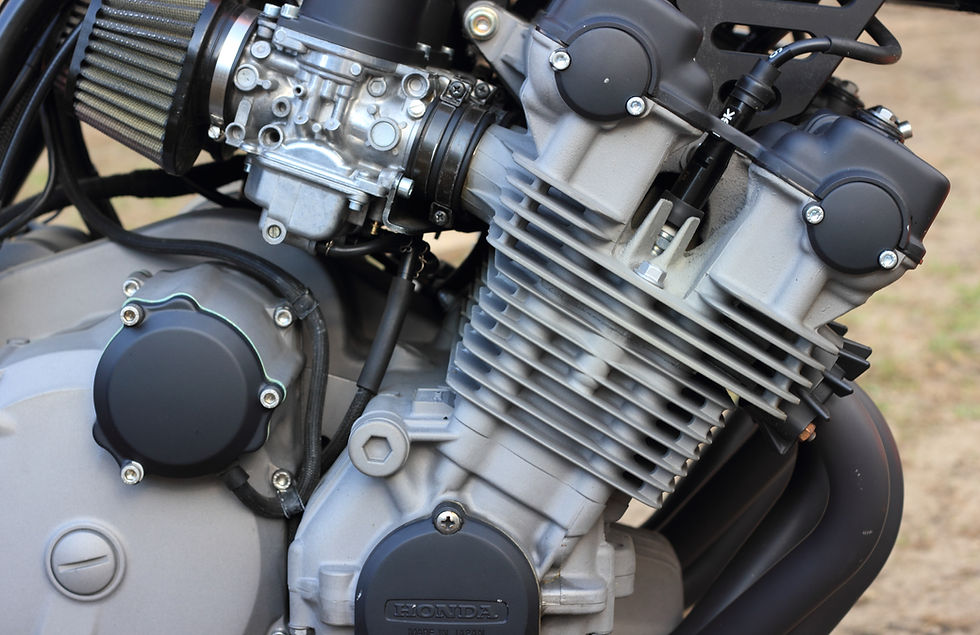
What is Component in React
React is a popular JavaScript library that provides a way for developers to build dynamic and interactive user interfaces. One of the key features of React is its component-based architecture, which allows developers to create reusable UI elements. In this blog post, we'll explore what components are in React, why they're important, and provide a real-world example to help you understand how to use them in your own projects.
Components are the building blocks of React applications. They're reusable UI elements that can be used to build complex user interfaces. Components can be divided into two types: functional and class components.
Functional components are JavaScript functions that return a React element. They're used for simple UI elements that don't have any state or lifecycle methods. Class components, on the other hand, are ES6 classes that extend the React.Component class. They're used for more complex UI elements that require state or lifecycle methods.
Why are Components Important in React?
Components are important in React because they provide a way to create reusable UI elements. Instead of writing the same code over and over again, developers can create components that can be reused throughout their application. This makes it easier to maintain and update the application as a whole.
Real-Word Examples
Here's an example of building a simple navigation bar component in React:
import React from 'react';
const NavBar = () => {
return (
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
<li><a href="/contact">Contact</a></li>
</ul>
</nav>
);
}
export default NavBar;
In this example, we're creating a functional component called NavBar that returns a basic navigation bar with three links: "Home", "About", and "Contact". We're using JSX syntax to create the HTML elements, which makes it easy to write HTML-like code directly in our JavaScript.
We can then use this NavBar component in our other React components, like so: import React from 'react';
import NavBar from './NavBar';
const App = () => {
return (
<div>
<NavBar />
<h1>Welcome to my app!</h1>
<p>Here's some content...</p>
</div>
);
}
export default App;
In this example, we're importing the NavBar component and including it at the top of our App component. This will render the navigation bar at the top of the page, above the main content.
By using components in this way, we can create reusable pieces of UI that can be easily shared across different pages and applications. We can also pass props to our components to customize their behavior or appearance, making them even more flexible and powerful.
Let's take a look at another example of using components in React. This time, we'll create a simple shopping cart component that displays a list of items and their prices, along with a total price.
import React from 'react';
const ShoppingCart = ({ items }) => {
const totalPrice = items.reduce((acc, item) => acc + item.price, 0);
return (
<div>
<h2>Shopping Cart</h2>
<ul>
{items.map((item) => (
<li key={item.id}>
{item.name} - ${item.price}
</li>
))}
</ul>
<h3>Total Price: ${totalPrice}</h3>
</div>
);
};
export default ShoppingCart;
In this example, we're creating a functional component called ShoppingCart that takes a prop called items. The items prop is an array of objects, where each object represents a product and has a name and price property.
Inside the component, we're using the reduce method to calculate the total price of all the items in the shopping cart. We're then using JSX to create a list of items and their prices, along with a total price at the bottom.
We can then use this ShoppingCart component in our other React components, like so: import React from 'react';
import ShoppingCart from './ShoppingCart';
const App = () => {
const items = [
{ id: 1, name: 'Shirt', price: 25.99 },
{ id: 2, name: 'Pants', price: 39.99 },
{ id: 3, name: 'Shoes', price: 69.99 },
];
return (
<div>
<h1>Welcome to my store!</h1>
<ShoppingCart items={items} />
</div>
);
};
export default App;
In this example, we're creating an array of items and passing it as a prop to the ShoppingCart component. This will render the shopping cart with the list of items and their prices, along with a total price at the bottom.
By using components in this way, we can create complex UI elements that are easy to reuse and customize. We can also pass data to our components via props, making them even more flexible and powerful.
Conclusion
Components are an essential part of React development, providing a way to create reusable UI elements that can be used throughout your application. By understanding what components are, why they're important, and how to use them in your own projects, you can become a more proficient React developer and build better user interfaces for your users. Whether you're building a simple "Hello, World!" component or a complex UI element with state and lifecycle methods, understanding how to use components in React is key to becoming a proficient developer.
Commenti